Python environment variable configuration steps: 1. Open the Python interpreter; 2. Import the os module; 3. Set the environment variables; 4. Get the environment variables.
#To configure environment variables in Python, you can follow the steps below:
1. Open the Python interpreter. You can open the Python interpreter by typing python at the command line.
2. Import the os module. Enter the following code in the Python interpreter:
import os
3. Set environment variables. You can use the os.environ property to set environment variables. For example, to set an environment variable named MY_VAR, you would use the following code:
os.environ['MY_VAR'] = 'my_value'
If you want to set multiple environment variables, you can use a dictionary to associate the variable names and values, and then pass the entire dictionary to os.environ:
os.environ.update({ 'VAR1': 'value1', 'VAR2': 'value2' })
4. Get environment variables. You can use the os.environ property to get the value of an environment variable. For example, to get the value of an environment variable named MY_VAR, you can use the following code:
my_var_value = os.environ['MY_VAR']
If you want to get the value of multiple environment variables, you can iterate over the os.environ dictionary:
for key, value in os.environ.items(): print(f'{key}={value}')
5. If you are using a Python virtual environment, you can set environment variables in the virtual environment. For example, to set an environment variable named MY_VAR, you would use the following code:
import os # 设置环境变量 os.environ['MY_VAR'] = 'my_value' # 运行Python代码 python my_script.py
This will set the MY_VAR environment variable in the virtual environment and run the my_script.py script.
The above is the detailed content of python environment variable configuration. For more information, please follow other related articles on the PHP Chinese website!
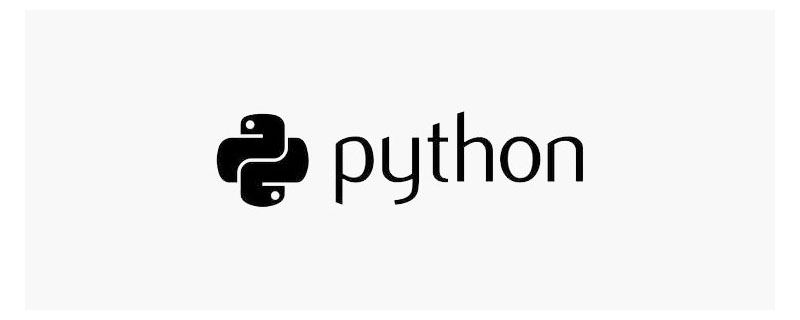
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
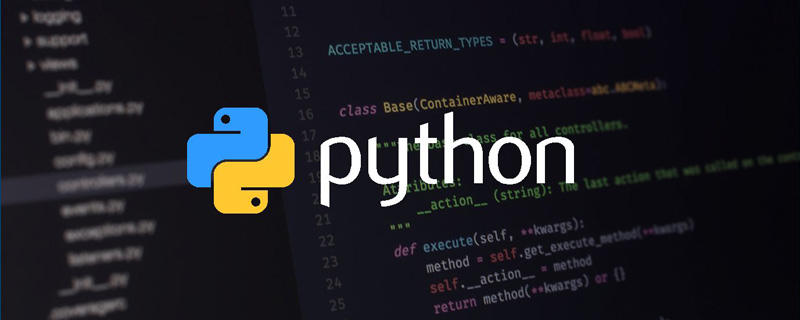
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
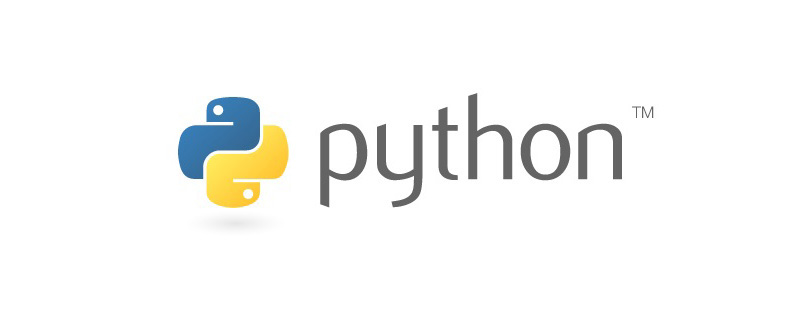
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
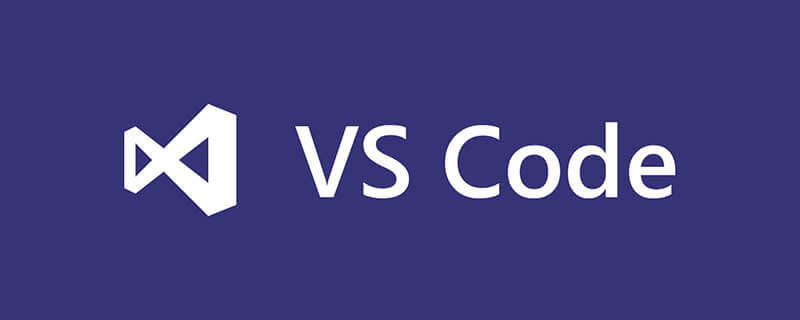
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
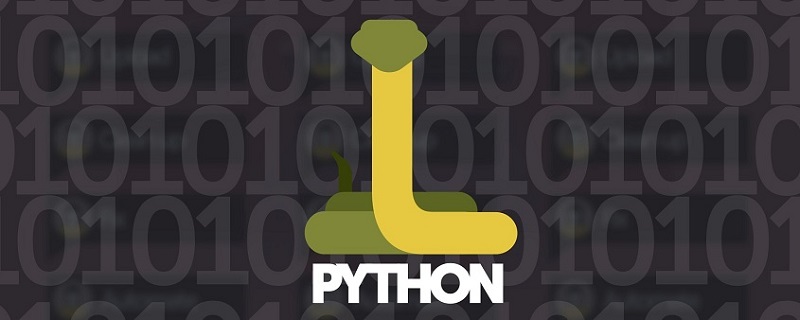
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
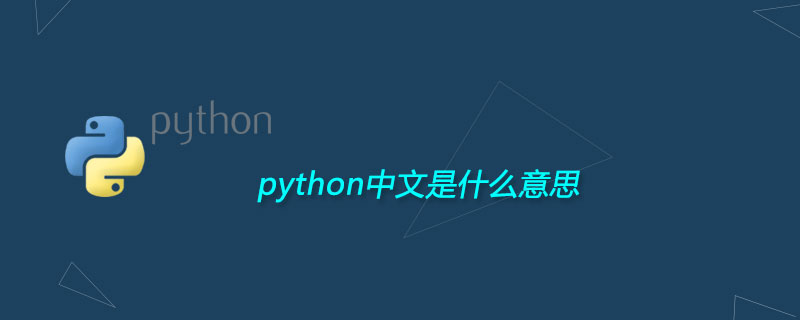
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。
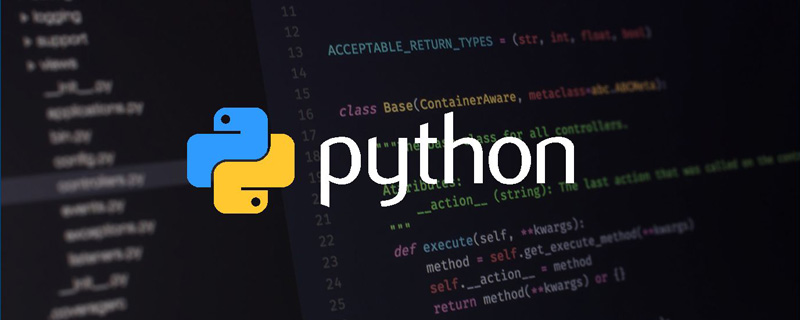
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
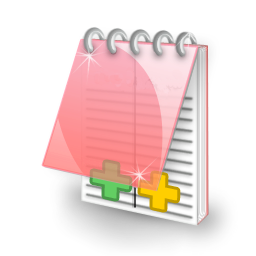
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
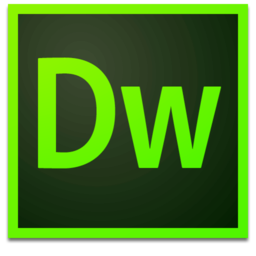
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
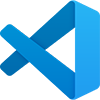
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
