


What are the usage scenarios of heap and priority queue in Python?
Heap is a special binary tree structure that is often used to efficiently maintain a dynamic collection. The heapq module in Python provides a heap implementation and can easily perform heap operations.
Priority queue is also a special data structure. Different from ordinary queues, each element of it has a priority associated with it. The highest priority element is taken out first. The heapq module in Python can also implement the priority queue function.
Below we introduce some specific scenarios of using heaps and priority queues, and give relevant code examples.
- Finding the Top K problem
It is a common problem to solve the first k largest or smallest elements in a sequence, such as solving the first k largest numbers or the first k smallest numbers. . This problem can be easily solved using a heap of size k or a priority queue.
import heapq def top_k_smallest(nums, k): heap = [] for num in nums: heapq.heappush(heap, num) if len(heap) > k: heapq.heappop(heap) return heap nums = [5, 3, 8, 2, 7, 1, 9] k = 3 result = top_k_smallest(nums, k) print(result) # 输出 [3, 2, 1]
- Merge ordered arrays
It is a common problem to merge multiple ordered numbers to form an ordered array. It can be implemented using a priority queue. Each time, the smallest element is taken from each array and put into the priority queue, and then the elements in the queue are taken out in turn.
import heapq def merge_sorted_arrays(arrays): result = [] pq = [] for array in arrays: if array: heapq.heappush(pq, (array[0], array)) while pq: smallest, array = heapq.heappop(pq) result.append(smallest) if array[1:]: heapq.heappush(pq, (array[1], array[1:])) return result arrays = [[1, 3, 5], [2, 4, 6], [0, 7, 8]] result = merge_sorted_arrays(arrays) print(result) # 输出 [0, 1, 2, 3, 4, 5, 6, 7, 8]
- Finding the median
Finding the median of a sequence is a classic problem. This can be achieved using two heaps, a max heap for the first half of the sequence and a min heap for the second half of the sequence. Keeping the sizes of the two heaps equal or different by one, the median can be taken at the top of the heap.
import heapq def median(nums): min_heap = [] max_heap = [] for num in nums: if len(max_heap) == 0 or num <= -max_heap[0]: heapq.heappush(max_heap, -num) else: heapq.heappush(min_heap, num) if len(max_heap) > len(min_heap) + 1: heapq.heappush(min_heap, -heapq.heappop(max_heap)) elif len(min_heap) > len(max_heap): heapq.heappush(max_heap, -heapq.heappop(min_heap)) if len(max_heap) > len(min_heap): return -max_heap[0] elif len(min_heap) > len(max_heap): return min_heap[0] else: return (-max_heap[0] + min_heap[0]) / 2 nums = [4, 2, 5, 7, 1, 8, 3, 6] result = median(nums) print(result) # 输出 4.5
The above are some common usage scenarios and sample codes of heap and priority queue in Python. Heaps and priority queues are some commonly used data structures, and mastering their use is very helpful for solving some complex problems.
The above is the detailed content of What are the usage scenarios of heap and priority queue in Python?. For more information, please follow other related articles on the PHP Chinese website!
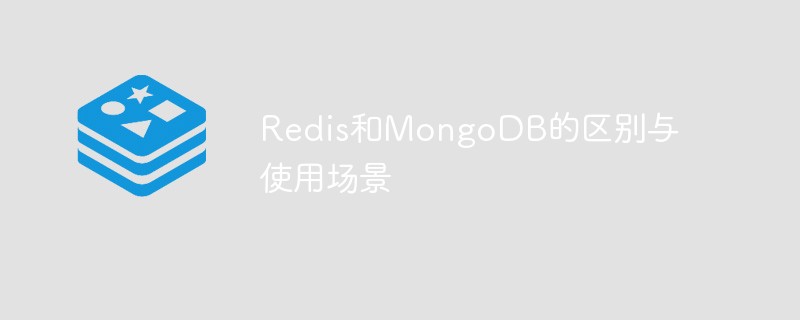
Redis和MongoDB都是流行的开源NoSQL数据库,但它们的设计理念和使用场景有所不同。本文将重点介绍Redis和MongoDB的区别和使用场景。Redis和MongoDB简介Redis是一个高性能的数据存储系统,常被用作缓存和消息中间件。Redis以内存为主要存储介质,但它也支持将数据持久化到磁盘上。Redis是一款键值数据库,它支持多种数据结构(例
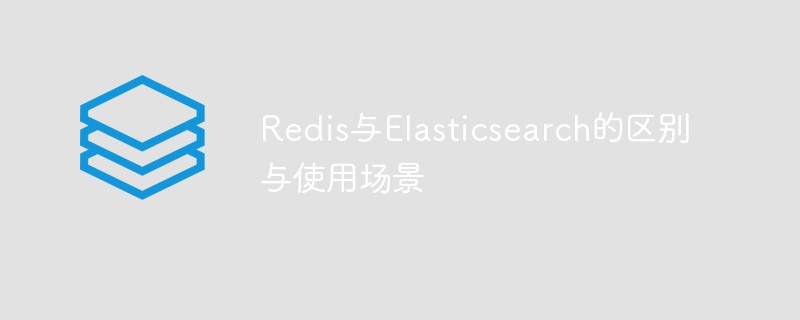
Redis与Elasticsearch的区别与使用场景随着互联网信息的快速发展和海量化,数据的高效存储和检索变得越来越重要。为此,NoSQL(NotOnlySQL)类型的数据库出现了,其中又以Redis和Elasticsearch较为流行。本文将对Redis和Elasticsearch进行比较,并探讨它们的使用场景。Redis与Elasticsearch
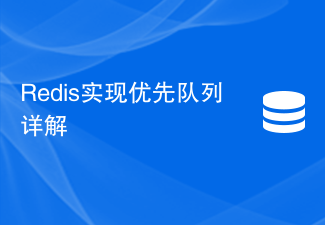
Redis实现优先队列详解优先队列是一种常见的数据结构,它可以按照某种规则对元素进行排序,并在队列操作时保持这个排序,从而使得队列中取出的元素总是按照预设的优先级进行。Redis作为一种内存数据库,因其快速、高效的数据访问能力,在实现优先队列时也有着优势。本文将详细介绍Redis实现优先队列的方法和应用。一、Redis实现基本原理Redis实现优先队列的基本
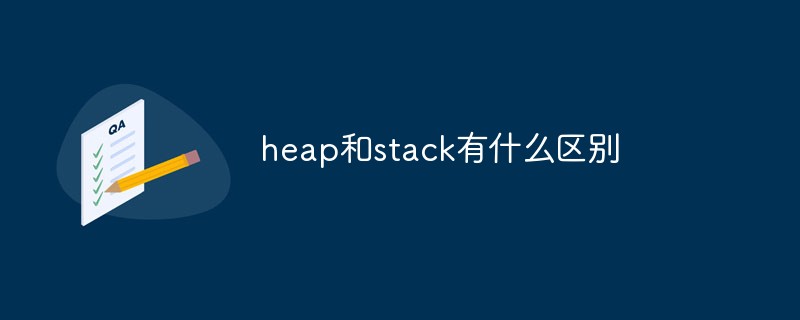
区别:1、堆(heap)的空间一般由程序员分配释放;而栈(stack)的空间由操作系统自动分配释放 。2、heap是存放在二级缓存中,生命周期由虚拟机的垃圾回收算法来决定;而stack使用的是一级缓存,通常都是被调用时处于存储空间中,调用完毕立即释放。3、数据结构不同,heap可以被看成是一棵树,而stack是一种先进后出的数据结构。
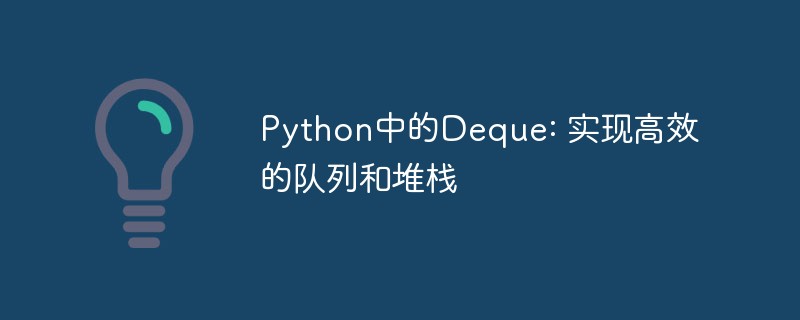
Python 中的 deque 是一个低级别的、高度优化的双端队列,对于实现优雅、高效的Pythonic 队列和堆栈很有用,它们是计算中最常见的列表式数据类型。本文中,云朵君将和大家一起学习如下:开始使用deque有效地弹出和追加元素访问deque中的任意元素用deque构建高效队列开始使用Deque向 Python 列表的右端追加元素和弹出元素的操作,一般非常高效。如果用大 O 表示时间复杂性,那么可以说它们是 O(1)。而当 Python 需要重新分配内存来增加底层列表以接受新的元素时,这些
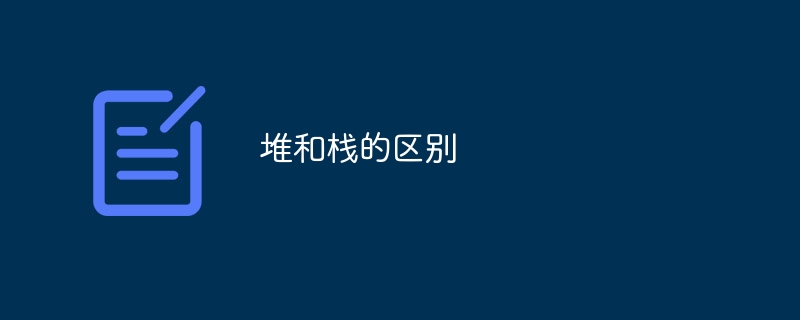
堆和栈的区别:1、内存分配方式不同,堆是由程序员手动分配和释放的,而栈是由操作系统自动分配和释放的;2、大小不同,栈的大小是固定的,而堆的大小是动态增长的;3、数据访问方式不同,在堆中,数据的访问是通过指针来实现的,而在栈中,数据的访问是通过变量名来实现的;4、数据的生命周期,在堆中,数据的生命周期可以很长,而在栈中,变量的生命周期是由其所在的作用域来决定的。
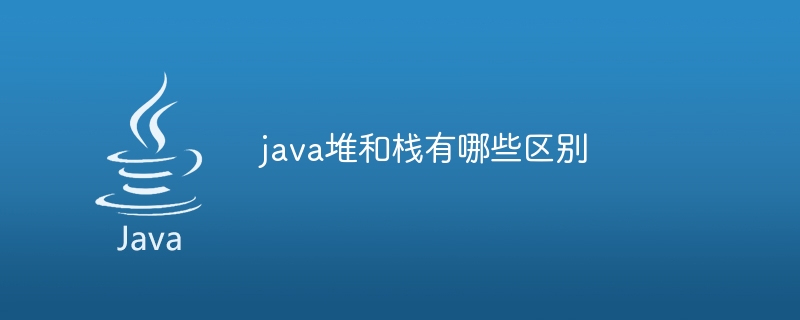
java堆和栈的区别:1、内存分配和管理;2、存储内容;3、线程执行和生命周期;4、性能影响。详细介绍:1、内存分配和管理,Java堆是动态分配的内存区域,主要用来存储对象实例,在Java中,对象是通过堆内存进行分配的,当创建一个对象时,Java虚拟机会在堆上分配相应的内存空间,并自动进行垃圾回收和内存管理,堆的大小可以在运行时动态调整,通过JVM参数进行配置等等。
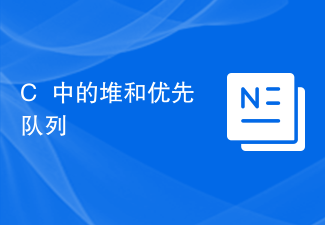
堆和优先队列是C++中常用的数据结构,它们都具有重要的应用价值。本文将分别对堆和优先队列进行介绍和解析,帮助读者更好地理解和使用它们。一、堆堆是一种特殊的树形数据结构,它可以用来实现优先队列。在堆中,每个节点都满足如下性质:它的值不小于(或不大于)其父节点的值。它的左右子树也是一个堆。我们将不小于其父节点的堆称为“最小堆”,将不大于其父节点的堆称为“最大堆”


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
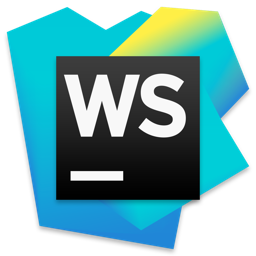
WebStorm Mac version
Useful JavaScript development tools
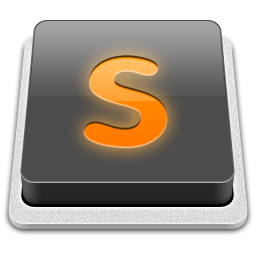
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
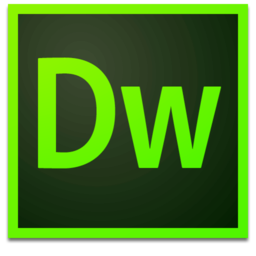
Dreamweaver Mac version
Visual web development tools
