


How to use PHP7’s anonymous functions and closures to optimize the maintainability and readability of your code?
PHP is a very popular programming language that is widely used in web development. PHP7 introduces many new features and improvements, including anonymous functions and closures. In this article, we’ll explore how to leverage PHP7’s anonymous functions and closures to optimize code maintainability and readability.
1. The basic concept of anonymous functions
Anonymous functions are functions that are not explicitly named and can be used directly in the code. Anonymous functions have been significantly improved in PHP7, supporting more syntax and features.
1.1 Basic syntax
The basic syntax of an anonymous function is as follows:
$func = function (参数列表) { // 函数体 };
For example, we can create a simple anonymous function that calculates the sum of two numbers:
$sum = function ($a, $b) { return $a + $b; }; echo $sum(1, 2); // 输出3
1.2 The concept of closure function
A closure function is a special anonymous function that can access variables in its outer scope. This makes closure functions very flexible and powerful and can be used in many complex programming scenarios.
2. Optimize code through anonymous functions and closures
2.1 Callback function
The callback function is a common programming pattern that can pass a function as a parameter to another function. To achieve more flexible control flow and logic processing. In PHP7, anonymous functions and closure functions are very suitable for use as callback functions.
For example, suppose we have an array and need to process each element and return a new array. The traditional approach is to use a foreach loop to traverse the array, and then add the processing results to a new array:
$array = [1, 2, 3]; $result = []; foreach ($array as $item) { $result[] = $item * 2; } print_r($result); // 输出[2, 4, 6]
Using anonymous functions and closure functions, we can achieve the same function more concisely through the array_map function :
$array = [1, 2, 3]; $result = array_map(function ($item) { return $item * 2; }, $array); print_r($result); // 输出[2, 4, 6]
2.2 Function combination
Function combination is a technology that combines multiple functions to implement complex logic. Anonymous functions and closures are great for function composition.
For example, suppose we have an array and need to process each element and then concatenate the results into a string. The traditional approach is to use a loop to iterate through the array and then process and join the elements one by one.
$array = [1, 2, 3]; $result = ''; foreach ($array as $item) { $result .= $item * 2 . ', '; } $result = rtrim($result, ', '); echo $result; // 输出2, 4, 6
Using anonymous functions and closure functions, we can achieve similar functions through the array_reduce function:
$array = [1, 2, 3]; $result = array_reduce($array, function ($carry, $item) { return $carry .= $item * 2 . ', '; }, ''); $result = rtrim($result, ', '); echo $result; // 输出2, 4, 6
By using anonymous functions and closure functions, we can further simplify and optimize the code, and Improve maintainability and readability.
Conclusion
The anonymous functions and closure functions introduced in PHP7 provide us with more programming flexibility and opportunities to optimize code. By rationally utilizing anonymous functions and closure functions, we can simplify and optimize code logic and improve code maintainability and readability. I hope this article can help you better understand and apply PHP7's anonymous functions and closure functions.
The above is the detailed content of How to use PHP7's anonymous functions and closures to optimize code maintainability and readability?. For more information, please follow other related articles on the PHP Chinese website!
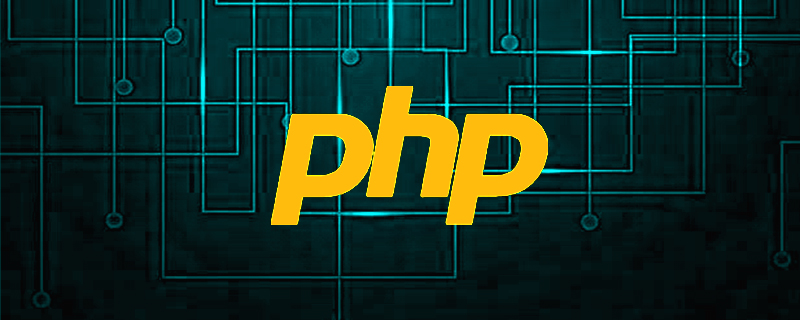
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
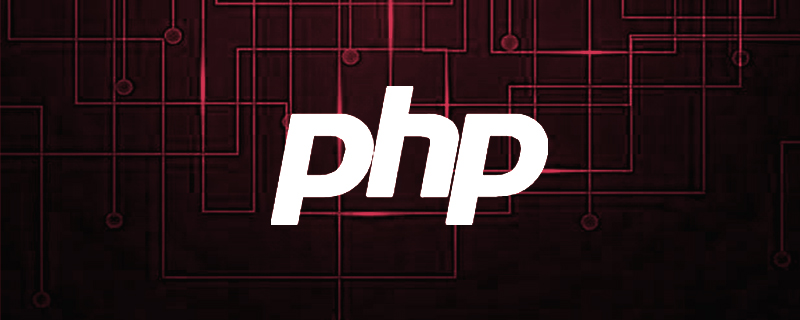
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
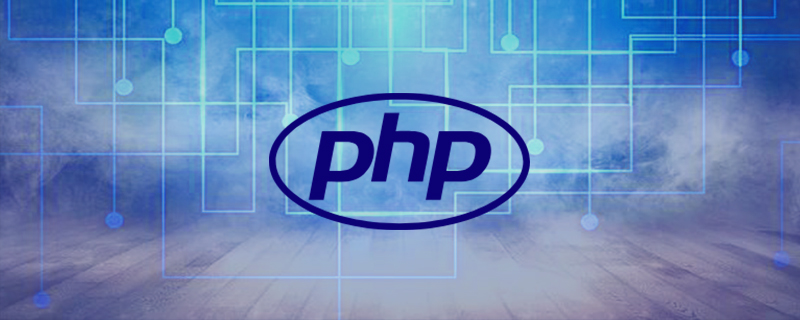
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
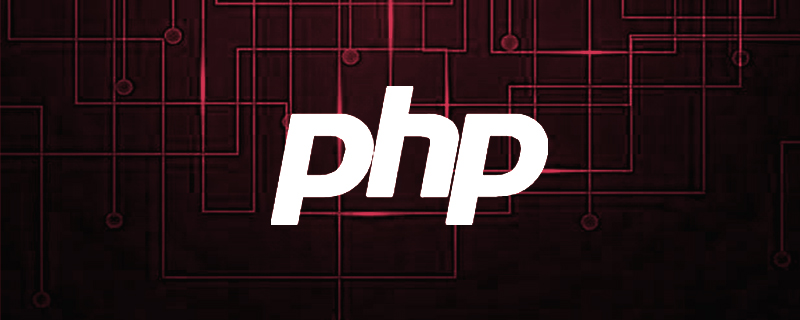
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
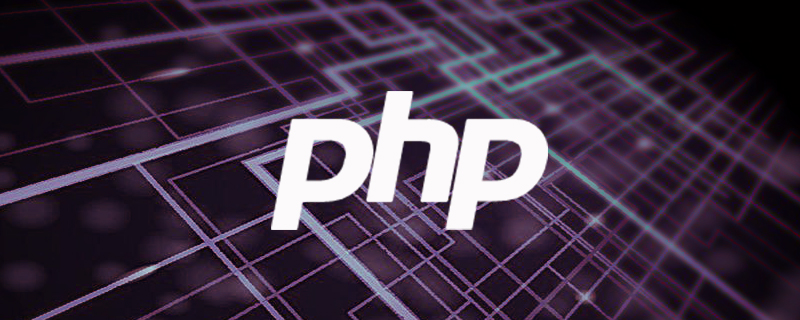
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
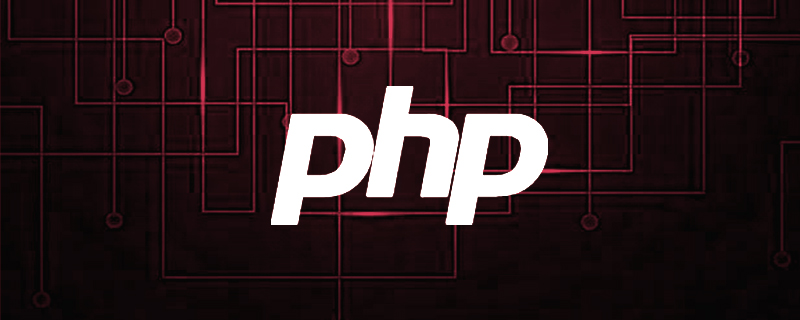
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
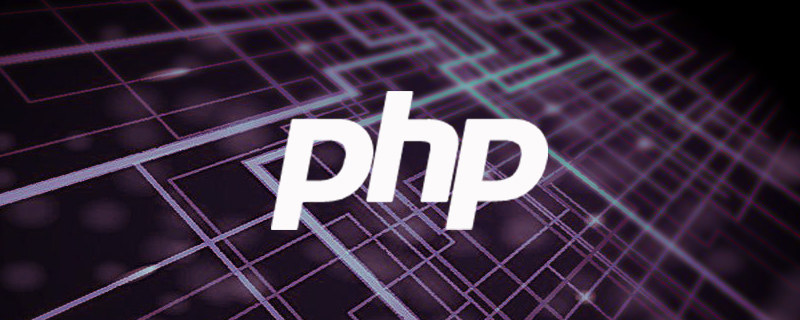
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
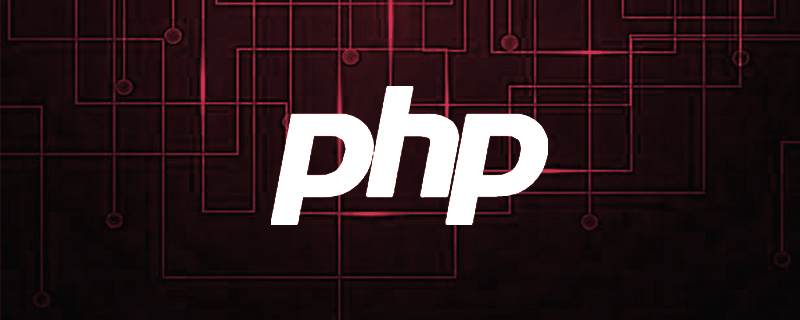
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
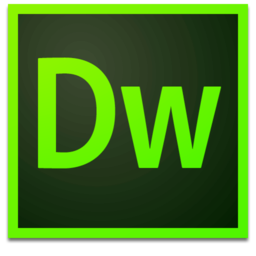
Dreamweaver Mac version
Visual web development tools
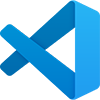
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
