


ChatGPT Python SDK Development Guide: Tips to Improve Conversation Experience
ChatGPT Python SDK Development Guide: Tips to Improve Dialogue Experience
Introduction:
ChatGPT is a powerful dialogue generation model launched by OpenAI, which can generate Smooth, logical natural language responses. In the process of using ChatGPT for conversational interaction, how to improve the user experience and make the conversation more coherent and intelligent has become the focus of developers. This article will introduce how to use the ChatGPT Python SDK, and provide some tips and specific code examples to help you improve your ChatGPT conversation experience.
1. Install ChatGPT Python SDK
1. First, make sure you have installed the Python3 environment.
2. Use the following command to install the ChatGPT Python SDK:
pip install openai
2. Create a ChatGPT instance
First, import the required libraries and modules:
import openai import json
Next, set up OpenAI API key:
openai.api_key = "YOUR_OPENAI_API_KEY"
Then, create a conversation session:
response = openai.ChatCompletion.create( model="gpt-3.5-turbo", messages=[ {"role": "system", "content": "You are a helpful assistant."}, {"role": "user", "content": "Who won the world series in 2020?"}, {"role": "assistant", "content": "The Los Angeles Dodgers won the World Series in 2020."}, {"role": "user", "content": "Where was it played?"} ] )
In the above code, we use the openai.ChatCompletion.create()
function to create a session, And the model of the conversation (gpt-3.5-turbo is used here) and the message list of the conversation are passed in. The message list contains the role (user or assistant) and content.
3. Tips for optimizing the conversation experience
1. Control the length of the reply
When conducting conversational interactions, we can enhance the coherence of the conversation by controlling the length of the reply. For example, you can set a maximum length for a reply, after which replies will be truncated.
response = openai.ChatCompletion.create( model="gpt-3.5-turbo", messages=[ {"role": "user", "content": "Translate the following English text to French: 'Hello, how are you?'"} ], max_tokens=30 )
2. Change the temperature of reply
The temperature of reply is a parameter that controls the creativity and diversity of replies. Lower temperatures (e.g., 0.2) generate more deterministic and conservative responses, while higher temperatures (e.g., 0.8) generate more creative and random responses.
response = openai.ChatCompletion.create( model="gpt-3.5-turbo", messages=[ {"role": "user", "content": "What's the weather like in New York?"} ], temperature=0.5 )
3. Readability of responses
Sometimes, the responses generated by ChatGPT may have some linguistic deviations or errors. The readability of the reply can be improved by assigning false
to the include_private
parameter.
response = openai.ChatCompletion.create( model="gpt-3.5-turbo", messages=[ {"role": "user", "content": "Who is the president of the United States?"} ], include_private=false )
4. Error handling and user prompts
If ChatGPT cannot generate a valid reply or the reply is too long, we can handle it accordingly based on the error information returned. The following is a sample code:
response = openai.ChatCompletion.create( model="gpt-3.5-turbo", messages=[ {"role": "user", "content": "Tell me a joke!"} ] ) if 'error' in response: print(response['error']['message']) else: # 处理回复逻辑
5. Summary
This article introduces how to use ChatGPT Python SDK to develop a dialogue system, and provides some tips and specific code examples to improve the dialogue experience. By properly setting parameters and handling errors, we can further improve the intelligence and consistency of responses generated by ChatGPT. I hope these tips can help you develop better dialogue interaction systems. If you need to know more about ChatGPT Python SDK, please refer to the official documentation.
References:
- OpenAI ChatGPT Python SDK documentation: https://github.com/openai/openai-python
- OpenAI ChatGPT API documentation: https: //platform.openai.com/docs/api-reference/chat/create
The above is the detailed content of ChatGPT Python SDK Development Guide: Tips to Improve Conversation Experience. For more information, please follow other related articles on the PHP Chinese website!
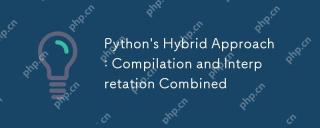
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
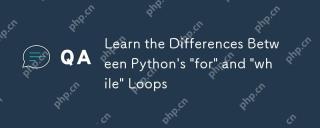
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
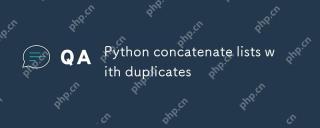
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.
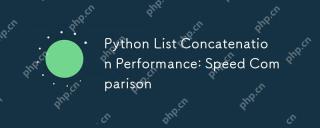
ThefastestmethodforlistconcatenationinPythondependsonlistsize:1)Forsmalllists,the operatorisefficient.2)Forlargerlists,list.extend()orlistcomprehensionisfaster,withextend()beingmorememory-efficientbymodifyinglistsin-place.
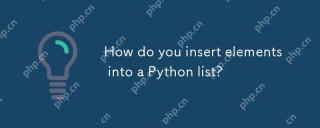
ToinsertelementsintoaPythonlist,useappend()toaddtotheend,insert()foraspecificposition,andextend()formultipleelements.1)Useappend()foraddingsingleitemstotheend.2)Useinsert()toaddataspecificindex,thoughit'sslowerforlargelists.3)Useextend()toaddmultiple
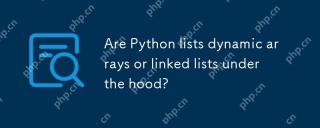
Pythonlistsareimplementedasdynamicarrays,notlinkedlists.1)Theyarestoredincontiguousmemoryblocks,whichmayrequirereallocationwhenappendingitems,impactingperformance.2)Linkedlistswouldofferefficientinsertions/deletionsbutslowerindexedaccess,leadingPytho
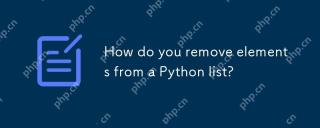
Pythonoffersfourmainmethodstoremoveelementsfromalist:1)remove(value)removesthefirstoccurrenceofavalue,2)pop(index)removesandreturnsanelementataspecifiedindex,3)delstatementremoveselementsbyindexorslice,and4)clear()removesallitemsfromthelist.Eachmetho
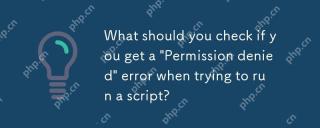
Toresolvea"Permissiondenied"errorwhenrunningascript,followthesesteps:1)Checkandadjustthescript'spermissionsusingchmod xmyscript.shtomakeitexecutable.2)Ensurethescriptislocatedinadirectorywhereyouhavewritepermissions,suchasyourhomedirectory.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
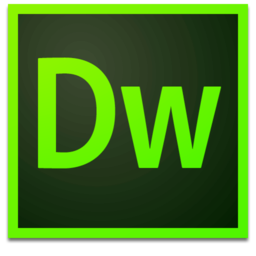
Dreamweaver Mac version
Visual web development tools
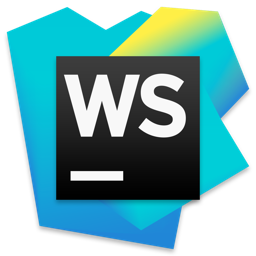
WebStorm Mac version
Useful JavaScript development tools
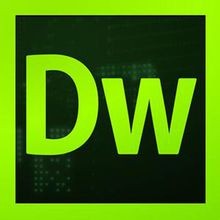
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
