


How to use Go language and Redis to develop an online question and answer platform
How to use Go language and Redis to develop an online question and answer platform
- Introduction
The online question and answer platform is a very common social platform that allows users to Post a question and get answers from other users. In this article, we will use Go language and Redis database to develop a simple online question and answer platform. Go is an efficient and reliable programming language, and Redis is a fast, scalable NoSQL database that is ideal for storing and retrieving questions and answers. - Environment preparation
Before you start, please make sure you have installed the Go language and Redis database, and have correctly configured the relevant environment variables. Project structure
We first need to create a new Go project, which can organize the code according to the following directory structure:-
project
- main.go
- question.go
- answer.go
在`main.go`文件中,我们将实现整个应用程序的入口点。在`question.go`和`answer.go`文件中,我们将定义问题和回答的相关结构和方法。
-
Connect Redis
atIn the main.go
file, we first need to import thegithub.com/go-redis/redis
package and create a Redis client instance. We can achieve this through the following code:package main import ( "fmt" "github.com/go-redis/redis" ) func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", // 无密码 DB: 0, // 默认数据库 }) pong, err := client.Ping().Result() fmt.Println(pong, err) }
In the above code, we created a Redis client instance named
client
and used thePing
method to test whether the connection is normal. -
Define the question structure
Next, in thequestion.go
file, we will define the structure of the question and related methods. The question structure can contain attributes such as title, content, creation time, etc. We can use the following code to achieve this:package main import "time" type Question struct { ID string `json:"id"` Title string `json:"title"` Content string `json:"content"` CreatedAt time.Time `json:"created_at"` } func (q *Question) Save(client *redis.Client) error { // 使用Redis的HSET命令保存问题 err := client.HSet("questions", q.ID, q).Err() if err != nil { return err } return nil } func (q *Question) GetByID(client *redis.Client, id string) error { // 使用Redis的HGET命令获取问题 val, err := client.HGet("questions", id).Result() if err != nil { return err } err = json.Unmarshal([]byte(val), q) if err != nil { return err } return nil } func (q *Question) GetAll(client *redis.Client) ([]Question, error) { // 使用Redis的HGETALL命令获取所有问题 vals, err := client.HGetAll("questions").Result() if err != nil { return nil, err } questions := make([]Question, len(vals)) i := 0 for _, val := range vals { err = json.Unmarshal([]byte(val), &questions[i]) if err != nil { return nil, err } i++ } return questions, nil }
In the above code, we define a
Question
structure and implement methods to save questions, obtain questions based on ID, and obtain all questions. -
Define the answer structure
Similarly, in theanswer.go
file, we will define the answer structure and related methods. The answer structure can contain attributes such as question ID, answer content, creation time, etc. We can use the following code to achieve this:package main import "time" type Answer struct { ID string `json:"id"` QuestionID string `json:"question_id"` Content string `json:"content"` CreatedAt time.Time `json:"created_at"` } func (a *Answer) Save(client *redis.Client) error { // 使用Redis的HSET命令保存回答 err := client.HSet("answers", a.ID, a).Err() if err != nil { return err } return nil } func (a *Answer) GetByQuestionID(client *redis.Client, questionID string) ([]Answer, error) { // 使用Redis的HGETALL命令获取指定问题的所有回答 vals, err := client.HGetAll("answers").Result() if err != nil { return nil, err } answers := make([]Answer, 0) for _, val := range vals { answer := Answer{} err = json.Unmarshal([]byte(val), &answer) // 遍历所有回答,找到与指定问题ID相匹配的回答 if answer.QuestionID == questionID { answers = append(answers, answer) } } return answers, nil }
In the above code, we define a
Answer
structure and implement the method of saving the answer and obtaining the answer based on the question ID. -
Using the Q&A platform
In themain
function of themain.go
file, we can test and demonstrate how to use online Q&A platform. We can implement it according to the following code:package main import ( "fmt" "github.com/go-redis/redis" ) func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", // 无密码 DB: 0, // 默认数据库 }) // 创建问题 question := Question{ ID: "1", Title: "如何学习Go语言?", Content: "我想学习Go语言,请问有什么好的学习资源推荐吗?", CreatedAt: time.Now(), } err := question.Save(client) if err != nil { fmt.Println("保存问题失败:", err) return } // 根据ID获取问题 err = question.GetByID(client, "1") if err != nil { fmt.Println("根据ID获取问题失败:", err) return } fmt.Println("问题标题:", question.Title) // 获取所有问题 questions, err := question.GetAll(client) if err != nil { fmt.Println("获取所有问题失败:", err) return } for _, q := range questions { fmt.Println("问题标题:", q.Title) } // 创建回答 answer := Answer{ ID: "1", QuestionID: "1", Content: "推荐去官方网站学习Go语言。", CreatedAt: time.Now(), } err = answer.Save(client) if err != nil { fmt.Println("保存回答失败:", err) return } // 根据问题ID获取回答 answers, err := answer.GetByQuestionID(client, "1") if err != nil { fmt.Println("根据问题ID获取回答失败:", err) return } for _, a := range answers { fmt.Println("回答内容:", a.Content) } }
In the above code, we demonstrate how to use the online Q&A platform by creating questions, getting questions based on IDs, getting all questions, creating answers, getting answers based on question IDs, etc.
So far, we have developed a simple online question and answer platform using Go language and Redis. Through this platform, users can post questions and get answers from other users. By studying the sample code provided in this article, you should be able to further extend and improve this platform to make it more suitable for practical application scenarios. Hope this article is helpful to you!
The above is the detailed content of How to use Go language and Redis to develop an online question and answer platform. For more information, please follow other related articles on the PHP Chinese website!
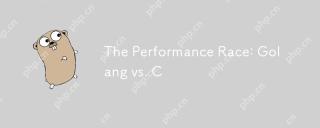
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
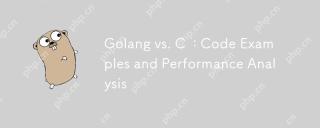
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
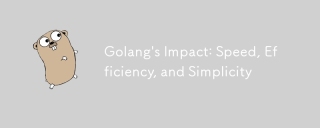
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
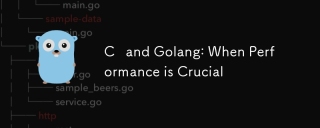
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
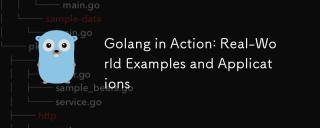
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
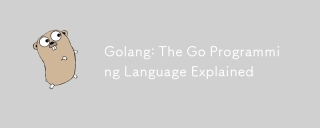
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
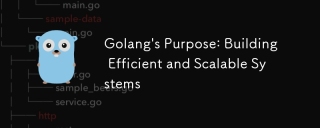
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
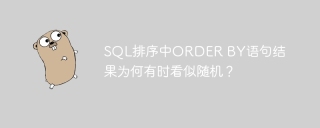
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
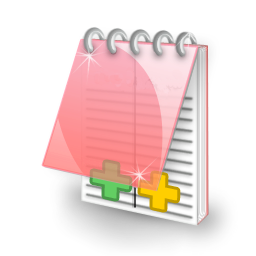
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
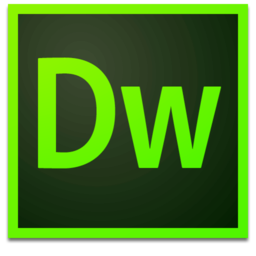
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor