ChatGPT Java: How to build a personalized recommendation system
ChatGPT Java: How to build a personalized recommendation system, specific code examples are required
In today's era of information explosion, personalized recommendation systems have become a key issue in the business field an important technology. By analyzing users' historical behavior and interests, these systems are able to provide users with recommended content that matches their personal preferences and needs. This article will introduce how to use Java to build a simple personalized recommendation system and provide specific code examples.
- Data collection and preprocessing
The core of the personalized recommendation system is the user's behavioral data. We need to collect users' historical browsing records, purchasing behavior, rating data, etc. In Java, a database can be used to store and manage this data. The following is a simple code example that connects to the database via Java JDBC and inserts the user's browsing history data:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.SQLException; public class DataCollector { private static final String JDBC_URL = "jdbc:mysql://localhost:3306/recommendation_system"; private static final String USERNAME = "root"; private static final String PASSWORD = "password"; public static void main(String[] args) { try(Connection connection = DriverManager.getConnection(JDBC_URL, USERNAME, PASSWORD)) { String sql = "INSERT INTO user_browsing_history (user_id, item_id, timestamp) VALUES (?, ?, ?)"; PreparedStatement statement = connection.prepareStatement(sql); // 假设有一个用户浏览了商品1和商品2 statement.setInt(1, 1); // 用户ID statement.setInt(2, 1); // 商品ID statement.setTimestamp(3, new java.sql.Timestamp(System.currentTimeMillis())); // 事件时间戳 statement.executeUpdate(); statement.setInt(1, 1); statement.setInt(2, 2); statement.setTimestamp(3, new java.sql.Timestamp(System.currentTimeMillis())); statement.executeUpdate(); } catch (SQLException e) { e.printStackTrace(); } } }
- User similarity calculation
In order to achieve personalization Recommendation, we need to find other users or products with similar interests to the target user. Here, we can use collaborative filtering algorithm to calculate the similarity between users. The following is a simple code example that uses cosine similarity to calculate the similarity between users:
import java.util.HashMap; import java.util.Map; public class SimilarityCalculator { public static void main(String[] args) { // 假设有两位用户 Map<Integer, Map<Integer, Integer>> userItems = new HashMap<>(); userItems.put(1, new HashMap<>()); userItems.get(1).put(1, 5); // 用户1对商品1的评分是5 userItems.get(1).put(2, 3); // 用户1对商品2的评分是3 userItems.put(2, new HashMap<>()); userItems.get(2).put(1, 4); // 用户2对商品1的评分是4 userItems.get(2).put(2, 2); // 用户2对商品2的评分是2 int userId1 = 1; int userId2 = 2; double similarity = calculateCosineSimilarity(userItems.get(userId1), userItems.get(userId2)); System.out.println("用户1和用户2的相似度为:" + similarity); } private static double calculateCosineSimilarity(Map<Integer, Integer> user1, Map<Integer, Integer> user2) { double dotProduct = 0.0; double normUser1 = 0.0; double normUser2 = 0.0; for (Integer itemId : user1.keySet()) { if (user2.containsKey(itemId)) { dotProduct += user1.get(itemId) * user2.get(itemId); } normUser1 += Math.pow(user1.get(itemId), 2); } for (Integer itemId : user2.keySet()) { normUser2 += Math.pow(user2.get(itemId), 2); } return dotProduct / (Math.sqrt(normUser1) * Math.sqrt(normUser2)); } }
- Recommendation algorithm implementation
With the similarity between users Based on the calculation results, we can use the neighborhood-based collaborative filtering algorithm to make recommendations. The following is a simple code example that generates recommendation results for target users based on the similarity between users:
import java.util.*; public class RecommendationEngine { public static void main(String[] args) { // 假设有3位用户 Map<Integer, Map<Integer, Integer>> userItems = new HashMap<>(); userItems.put(1, new HashMap<>()); userItems.get(1).put(1, 5); // 用户1对商品1的评分是5 userItems.get(1).put(2, 3); // 用户1对商品2的评分是3 userItems.get(1).put(3, 4); // 用户1对商品3的评分是4 userItems.put(2, new HashMap<>()); userItems.get(2).put(1, 4); // 用户2对商品1的评分是4 userItems.get(2).put(3, 2); // 用户2对商品3的评分是2 userItems.put(3, new HashMap<>()); userItems.get(3).put(2, 5); // 用户3对商品2的评分是5 userItems.get(3).put(3, 2); // 用户3对商品3的评分是2 int targetUserId = 1; Map<Integer, Double> recommendItems = generateRecommendations(userItems, targetUserId); System.out.println("为用户1生成的推荐结果为:" + recommendItems); } private static Map<Integer, Double> generateRecommendations(Map<Integer, Map<Integer, Integer>> userItems, int targetUserId) { Map<Integer, Double> recommendItems = new HashMap<>(); Map<Integer, Integer> targetUserItems = userItems.get(targetUserId); for (Integer userId : userItems.keySet()) { if (userId != targetUserId) { Map<Integer, Integer> otherUserItems = userItems.get(userId); double similarity = calculateCosineSimilarity(targetUserItems, otherUserItems); for (Integer itemId : otherUserItems.keySet()) { if (!targetUserItems.containsKey(itemId)) { double rating = otherUserItems.get(itemId); double weightedRating = rating * similarity; recommendItems.put(itemId, recommendItems.getOrDefault(itemId, 0.0) + weightedRating); } } } } return recommendItems; } private static double calculateCosineSimilarity(Map<Integer, Integer> user1, Map<Integer, Integer> user2) { // 略,与上一个代码示例中的calculateCosineSimilarity()方法相同 } }
Through the above steps, we can use Java to build a simple personalized recommendation system. Of course, this is only the basis of a personalized recommendation system, and there is still a lot of room for optimization and expansion. I hope this article will help you understand the process of building a personalized recommendation system.
The above is the detailed content of ChatGPT Java: How to build a personalized recommendation system. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
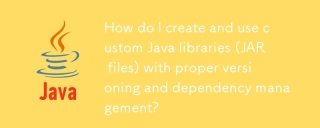
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
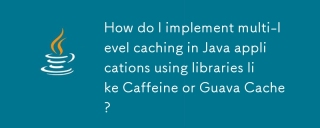
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
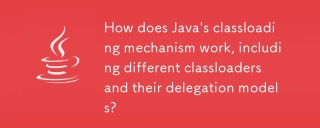
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
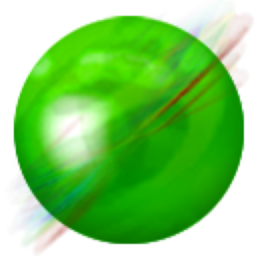
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
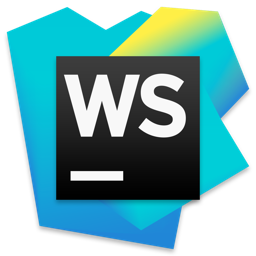
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version