How to implement task queue using Go language and Redis
How to implement task queue using Go language and Redis
Introduction:
In actual software development, we often encounter scenarios where a large number of tasks need to be processed. In order to improve processing efficiency and reliability, we can use task queues to distribute and execute these tasks. This article will introduce how to use Go language and Redis to implement a simple task queue, as well as specific code examples.
1. What is a task queue
Task queue is a common mechanism for distributing and executing tasks. It stores pending tasks in a queue, and then multiple consumers (also called worker threads) take the tasks out of the queue and execute them. The advantage of the task queue is that it can realize asynchronous processing of tasks and improve the overall processing capability and reliability.
2. Preparation
Before using Go language and Redis to implement task queue, we need to install and configure the Go language and Redis environment. Make sure you have installed the Go language environment and can execute Go commands normally. In addition, we also need to install Redis and start the Redis server. You can download the latest version of Redis through the Redis official website (https://redis.io).
3. Code Implementation
Next, we will use Go language to write a simple task queue code example. First, we need to install the Go Redis client (go-redis), which can be installed with the following command:
go get github.com/go-redis/redis/v8
Then, we create a file named main.go and write the following code in the file:
package main import ( "fmt" "github.com/go-redis/redis/v8" "time" ) func main() { // 创建Redis客户端 client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", // Redis服务器地址和端口 Password: "", // Redis密码,如果有的话 DB: 0, // 连接的Redis数据库编号 }) // 向任务队列中添加任务 err := client.RPush("task_queue", "task1").Err() if err != nil { panic(err) } // 从任务队列中取出任务并执行 for { result, err := client.LPop("task_queue").Result() if err == redis.Nil { // 队列为空,暂停一段时间后继续轮询 time.Sleep(time.Second) continue } else if err != nil { panic(err) } // 执行任务 fmt.Println("执行任务:", result) } }
In the above code, we first create a Redis client and specify the address and port of the connected Redis server. We then added a task to the task queue using the RPush
function. Next, we use the LPop
function to remove the task from the task queue and execute it. If the task queue is empty, polling will continue after a period of pause.
4. Run the code
After completing the code writing, we can run the code through the following command:
go run main.go
After the code is executed, you will see the output of an executing Task.
5. Summary
This article introduces how to use Go language and Redis to implement a simple task queue, and gives specific code examples. By using task queues, we can implement asynchronous processing of tasks and improve the processing power and reliability of the system. I hope the content of this article can help you understand and apply the concepts and techniques of task queues.
The above is the detailed content of How to implement task queue using Go language and Redis. For more information, please follow other related articles on the PHP Chinese website!
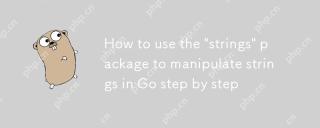
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
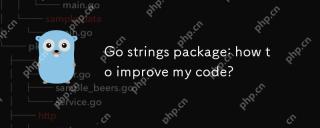
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
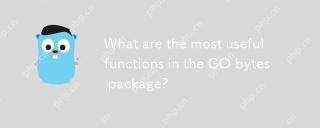
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
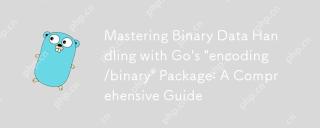
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
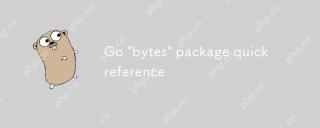
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
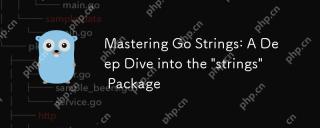
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
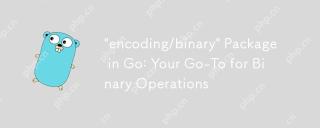
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
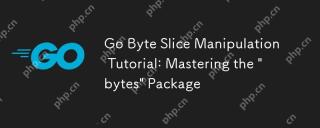
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
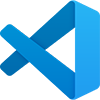
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
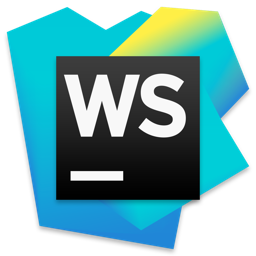
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Notepad++7.3.1
Easy-to-use and free code editor
