


What is the difference between iterators and generators in Python?
What is the difference between iterators and generators in Python?
In Python programming, iterators and generators are tools used to process iterable objects. Both of them can be used to traverse data, but there are some differences in implementation.
An iterator is an object that implements the iterator protocol. The iterator object needs to contain two methods: __iter__()
and __next__()
. Among them, the __iter__()
method returns the iterator object itself, while the __next__()
method returns the next element in the iterable object. If there are no more elements to iterate over, the __next__()
method must raise a StopIteration exception. Here is a simple iterator example:
class MyIterator: def __init__(self, limit): self.limit = limit self.current = 0 def __iter__(self): return self def __next__(self): if self.current < self.limit: value = self.current self.current += 1 return value else: raise StopIteration my_iterator = MyIterator(5) for num in my_iterator: print(num)
A generator is a special kind of iterator whose implementation is more concise. Generators use the keyword yield
to define functions. When the function is called, it returns a generator object. Each time the __next__()
method of the generator object is called, the function will resume execution until it encounters the yield
statement, returning the value after yield to the caller, and pausing the function. implement. Then, the next time the __next__()
method is called, the function continues execution from where it paused the last yield statement until the yield statement is encountered again. The following is sample code for implementing the Fibonacci sequence using generators:
def fib_generator(limit): a, b = 0, 1 for _ in range(limit): yield a a, b = b, a + b fib = fib_generator(5) for num in fib: print(num)
Although iterators and generators differ in how they are implemented, they are very similar in use. By using a for loop, we can iterate through the iterator and generator objects and get each element they produce. For example, the iterator object my_iterator
and the generator object fib
in the above example code can access the elements they produce one by one through a for loop.
It should be noted that generators are lazily evaluated, which means that they only generate values when needed, rather than generating all values in advance. This makes generators very efficient when processing large amounts of data, as they do not need to load all the data into memory at once.
To summarize, an iterator is an object that implements the iterator protocol, and a generator is a special iterator that uses the yield statement to define functions. Both can be used to iterate over data, but generators are simpler to implement and feature lazy evaluation. In actual development, selecting appropriate tools according to specific needs can improve the efficiency and readability of the program.
The above is the detailed content of What is the difference between iterators and generators in Python?. For more information, please follow other related articles on the PHP Chinese website!
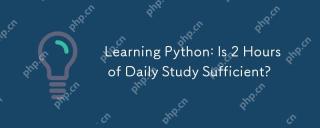
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
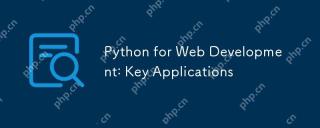
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
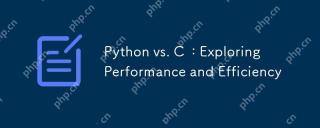
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
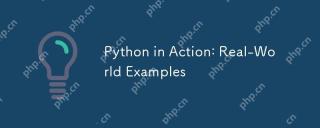
Python's real-world applications include data analytics, web development, artificial intelligence and automation. 1) In data analysis, Python uses Pandas and Matplotlib to process and visualize data. 2) In web development, Django and Flask frameworks simplify the creation of web applications. 3) In the field of artificial intelligence, TensorFlow and PyTorch are used to build and train models. 4) In terms of automation, Python scripts can be used for tasks such as copying files.
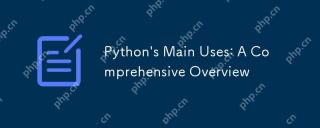
Python is widely used in data science, web development and automation scripting fields. 1) In data science, Python simplifies data processing and analysis through libraries such as NumPy and Pandas. 2) In web development, the Django and Flask frameworks enable developers to quickly build applications. 3) In automated scripts, Python's simplicity and standard library make it ideal.
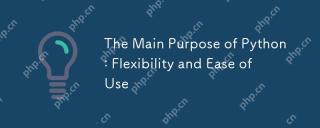
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
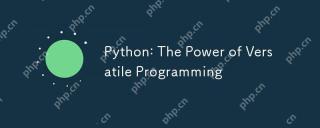
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
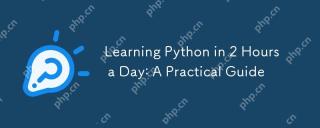
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
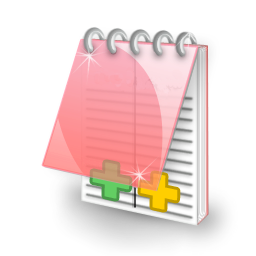
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
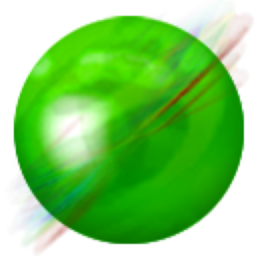
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment