How to use the Hyperf framework for request merging
With the development of the Internet and the increase in user needs, the number of requests in web applications is also increasing. In order to improve performance and efficiency, request merging has become an important technical means. In the Hyperf framework, we can easily implement the requested merge operation.
1. Project preparation
Before starting, make sure that the Hyperf framework has been installed and a new project has been created.
2. Create a service class for merge requests
First, we need to create a service class to handle merge requests. In the app/Service directory, create a file named RequestMergeService.
<?php declare(strict_types=1); namespace AppService; use HyperfGuzzleClientFactory; use HyperfUtilsApplicationContext; class RequestMergeService { public function sendRequests(array $urls): array { $client = $this->getClient(); $promises = []; foreach ($urls as $url) { $promises[$url] = $client->getAsync($url); } $results = []; foreach ($promises as $url => $promise) { $response = $promise->wait(); $results[$url] = $response->getBody()->getContents(); } return $results; } private function getClient() { $container = ApplicationContext::getContainer(); $factory = $container->get(ClientFactory::class); return $factory->create(); } }
3. Create a controller for merging requests
Next, we need to create a controller to receive the request and call the method in RequestMergeService to merge the request. In the app/Controller directory, create a file named RequestMergeController.
<?php declare(strict_types=1); namespace AppController; use AppServiceRequestMergeService; use HyperfHttpServerAnnotationController; use HyperfHttpServerAnnotationGetMapping; use HyperfDiAnnotationInject; /** * @Controller * @GetMapping("/request/merge") */ class RequestMergeController { /** * @Inject * @var RequestMergeService */ private $requestMergeService; public function index() { $urls = [ 'http://example.com/api/user/1', 'http://example.com/api/user/2', 'http://example.com/api/user/3', ]; $result = $this->requestMergeService->sendRequests($urls); return $result; } }
4. Configure routing
Open the config/routes.php file and add the following routing configuration:
use AppControllerRequestMergeController; Router::addRoute(['GET', 'POST', 'HEAD'], '/request/merge', [RequestMergeController::class, 'index']);
5. Test request merging
Start the Hyerpf project and use the browser Visit http://localhost:9501/request/merge to get the results of the merge request.
6. Summary
This article introduces how to use the Hyperf framework for request merging. By creating the RequestMergeService service class and the RequestMergeController controller, we can easily implement the request merging function. In this way, it can not only improve performance and reduce the number of requests, but also reduce network overhead and improve user experience.
The above is the detailed content of How to use Hyperf framework for request merging. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
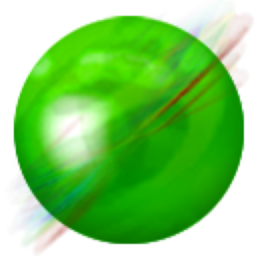
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
