


How to use JavaScript to achieve infinite loading effect of tab content?
How to use JavaScript to achieve infinite loading effect of tab content?
In web design and development, tabs are a commonly used function for displaying and switching content. When there is a lot of tab content, in order to improve the user experience, you can use the infinite loading effect to load the content in batches. This article explains how to use JavaScript to achieve this effect, with specific code examples.
First, we need an HTML structure for displaying the tab content. Taking a simple tab as an example, we can use the following code:
<div class="tab-container"> <div class="tab"> <div class="tab-item active" data-tab="tab1">选项卡1</div> <div class="tab-item" data-tab="tab2">选项卡2</div> <div class="tab-item" data-tab="tab3">选项卡3</div> </div> <div class="tab-content"> <div id="tab1" class="tab-pane active">选项卡1的内容</div> <div id="tab2" class="tab-pane">选项卡2的内容</div> <div id="tab3" class="tab-pane">选项卡3的内容</div> </div> </div>
Next, the key to achieving an infinite loading effect in JavaScript is to listen to scroll events and load the next batch of content when scrolling to the bottom. We can use the following code to implement this function:
// 获取选项卡内容容器和当前活跃的选项卡索引 var tabContent = document.querySelector('.tab-content'); var activeTabIndex = 0; // 监听滚动事件 window.addEventListener('scroll', function() { // 当滚动到底部时 if (window.innerHeight + window.pageYOffset >= tabContent.offsetHeight) { // 加载下一批内容 loadMoreContent(); } }); // 加载下一批内容 function loadMoreContent() { // 根据当前活跃的选项卡索引获取下一个选项卡的索引 var nextTabIndex = (activeTabIndex + 1) % 3; // 这里假设一共有3个选项卡 // 根据选项卡索引获取对应的选项卡内容 var nextTabContent = document.getElementById('tab' + (nextTabIndex + 1)); // 创建新的选项卡内容 div 元素,并将下一个选项卡内容添加到其中 var newTabContent = document.createElement('div'); newTabContent.classList.add('tab-pane'); newTabContent.appendChild(nextTabContent.cloneNode(true)); // 将新的选项卡内容添加到选项卡内容容器中 tabContent.appendChild(newTabContent); // 更新活跃的选项卡索引 activeTabIndex = nextTabIndex; }
The above code implements the function of listening to scroll events and loading the next batch of content when scrolling to the bottom. The specific implementation steps are as follows:
- First obtain the tab content container and the currently active tab index.
- Then listen to the scroll event and trigger the function to load the next batch of content when scrolling to the bottom.
- In the load next batch of content function, get the index of the next tab based on the currently active tab index.
- Get the corresponding tab content based on the tab index and create a new tab content element.
- Add the next tab content to a new tab content element and add it to the tab content container.
- Last update active tab index.
Through the above code, we can achieve the infinite loading effect of tab content. When the user scrolls to the bottom, the next batch of content is automatically loaded, improving the user experience.
I hope this article can help you understand how to use JavaScript to achieve infinite loading of tab content. If you have any other questions, please feel free to ask further.
The above is the detailed content of How to use JavaScript to achieve infinite loading effect of tab content?. For more information, please follow other related articles on the PHP Chinese website!
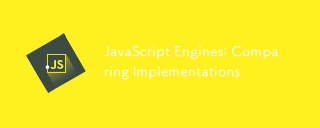
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
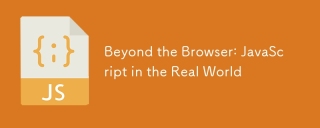
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
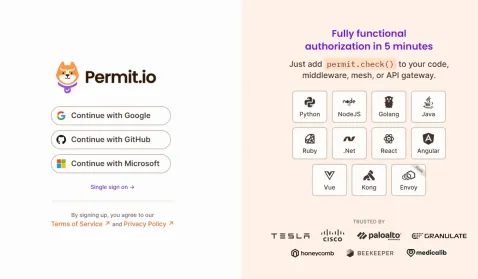
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
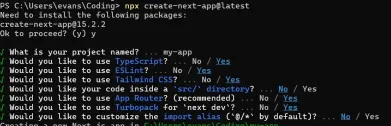
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
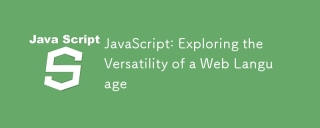
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
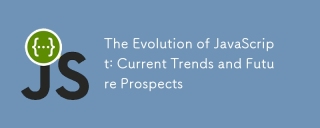
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
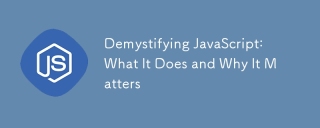
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
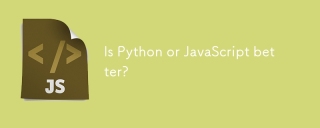
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
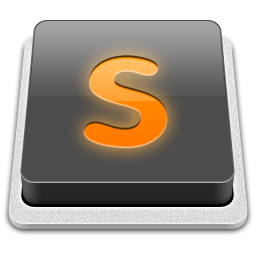
SublimeText3 Mac version
God-level code editing software (SublimeText3)
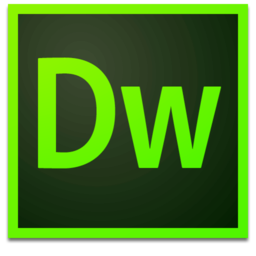
Dreamweaver Mac version
Visual web development tools