How to use metaprogramming techniques in Python
Introduction: Metaprogramming is a programming paradigm that refers to the ability to create or modify code at runtime. As a dynamic language, Python has powerful metaprogramming capabilities. This article will introduce commonly used metaprogramming techniques in Python and give specific code examples.
1. Using metaclasses
Metaclasses are classes used to create classes. By defining your own metaclass, you can customize the class creation process. The following is an example of using a metaclass:
class Meta(type): def __new__(cls, name, bases, attrs): attrs['attribute'] = '新增的属性' return super().__new__(cls, name, bases, attrs) class MyClass(metaclass=Meta): pass my_obj = MyClass() print(my_obj.attribute) # 输出:新增的属性
In this example, we have customized a metaclass Meta
, whose __new__
method will be used when creating the class Adds a new attribute attribute
to the class's attribute dictionary. When we create an instance of MyClass
, the instance will inherit the behavior of the metaclass Meta
and thus have new attributes.
2. Use decorators
A decorator is a special function used to decorate a function or class, which can dynamically modify the behavior of the modified object at runtime. The following is an example of using a decorator:
def decorator(func): def wrapper(*args, **kwargs): print("在函数调用之前执行的操作") result = func(*args, **kwargs) print("在函数调用之后执行的操作") return result return wrapper @decorator def my_function(): print("这是我的函数") my_function() # 输出:在函数调用之前执行的操作 # 这是我的函数 # 在函数调用之后执行的操作
In this example, we define a decorator decorator
, which will be called before the decorated function my_function
and perform some operations respectively after calling. By using the @decorator
syntax, we apply decorator
to my_function
, thus modifying the behavior of my_function
.
3. Use meta-attributes
Meta-attributes are a special form of class attributes, which can be used to dynamically modify the attributes of a class when defining a class. The following is an example of using meta attributes:
class MyMeta(type): def __new__(cls, name, bases, attrs): attrs['meta_attribute'] = '元属性的值' return super().__new__(cls, name, bases, attrs) class MyMetaClass(metaclass=MyMeta): pass print(MyMetaClass.meta_attribute) # 输出:元属性的值
In this example, we define a metaclass MyMeta
whose __new__
method is used during the creation of the class Added a meta-attribute meta_attribute
to the class's attributes dictionary. When we access the class attribute meta_attribute
of MyMetaClass
, we can get the value of the meta attribute.
Summary:
This article introduces commonly used metaprogramming techniques in Python and gives specific code examples. By using metaclasses, decorators, and metaproperties, we can dynamically create, modify, and extend code at runtime, enabling more flexible and powerful programming capabilities. Metaprogramming is a powerful feature in Python, and mastering these skills will be of great help to our development work. I hope this article can help you understand and use metaprogramming techniques in Python!
The above is the detailed content of How to use metaprogramming techniques in Python. For more information, please follow other related articles on the PHP Chinese website!
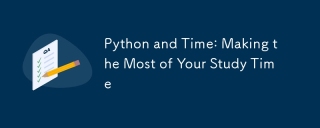
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
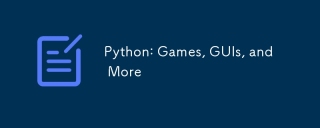
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
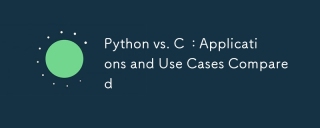
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
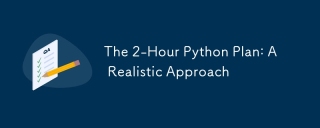
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
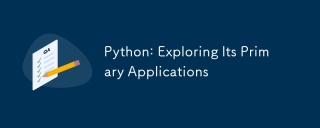
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
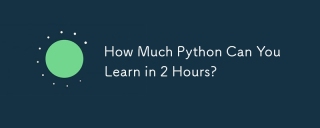
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
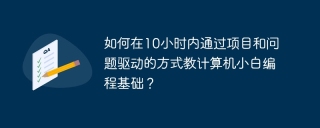
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
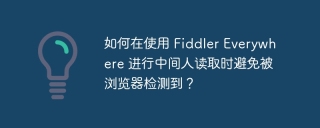
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
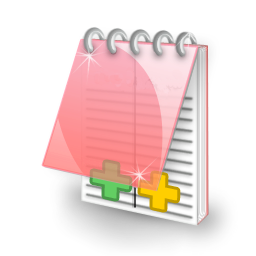
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
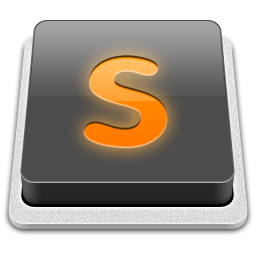
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor