// +----------------------------------------------------------------------+
// | PHP Version 4 |
// +----------------------------------------------------------------------+
// | Copyright (c) 1997-2002 The PHP Group |
// +----------------------------------------------------------------------+
// | This source file is subject to version 2.02 of the PHP license, |
// | that is bundled with this package in the file LICENSE, and is |
// | available at through the world-wide-web at |
// | http://www.php.net/license/2_02.txt. |
// | If you did not receive a copy of the PHP license and are unable to |
// | obtain it through the world-wide-web, please send a note to |
// | license@php.net so we can mail you a copy immediately. |
// +----------------------------------------------------------------------+
// | Authors: Richard Heyes
// +----------------------------------------------------------------------+
/**
* Pager class
*
* Handles paging a set of data. For usage see the example.php provided.
*
*/
class Pager {
/**
* Current page
* @var integer
*/
var $_currentPage;
/**
* Items per page
* @var integer
*/
var $_perPage;
/**
* Total number of pages
* @var integer
*/
var $_totalPages;
/**
* Item data. Numerically indexed array...
* @var array
*/
var $_itemData;
/**
* Total number of items in the data
* @var integer
*/
var $_totalItems;
/**
* Page data generated by this class
* @var array
*/
var $_pageData;
/**
* Constructor
*
* Sets up the object and calculates the total number of items.
*
* @param $params An associative array of parameters This can contain:
* currentPage Current Page number (optional)
* perPage Items per page (optional)
* itemData Data to page
*/
function pager($params = array())
{
global $HTTP_GET_VARS;
$this->_currentPage = max((int)@$HTTP_GET_VARS['pageID'], 1);
$this->_perPage = 8;
$this->_itemData = array();
foreach ($params as $name => $value) {
$this->{'_' . $name} = $value;
}
$this->_totalItems = count($this->_itemData);
}
/**
* Returns an array of current pages data
*
* @param $pageID Desired page ID (optional)
* @return array Page data
*/
function getPageData($pageID = null)
{
if (isset($pageID)) {
if (!empty($this->_pageData[$pageID])) {
return $this->_pageData[$pageID];
} else {
return FALSE;
}
}
if (!isset($this->_pageData)) {
$this->_generatePageData();
}
return $this->getPageData($this->_currentPage);
}
/**
* Returns pageID for given offset
*
* @param $index Offset to get pageID for
* @return int PageID for given offset
*/
function getPageIdByOffset($index)
{
if (!isset($this->_pageData)) {
$this->_generatePageData();
}
if (($index % $this->_perPage) > 0) {
$pageID = ceil((float)$index / (float)$this->_perPage);
} else {
$pageID = $index / $this->_perPage;
}
return $pageID;
}
/**
* Returns offsets for given pageID. Eg, if you
* pass it pageID one and your perPage limit is 10
* it will return you 1 and 10. PageID of 2 would
* give you 11 and 20.
*
* @params pageID PageID to get offsets for
* @return array First and last offsets
*/
function getOffsetByPageId($pageid = null)
{
$pageid = isset($pageid) ? $pageid : $this->_currentPage;
if (!isset($this->_pageData)) {
$this->_generatePageData();
}
if (isset($this->_pageData[$pageid])) {
return array(($this->_perPage * ($pageid - 1)) + 1, min($this->_totalItems, $this->_perPage * $pageid));
} else {
return array(0,0);
}
}
/**
* Returns back/next and page links
*
* @param $back_html HTML to put inside the back link
* @param $next_html HTML to put inside the next link
* @return array Back/pages/next links
*/
function getLinks($back_html = '>')
{
$url = $this->_getLinksUrl();
$back = $this->_getBackLink($url, $back_html);
$pages = $this->_getPageLinks($url);
$next = $this->_getNextLink($url, $next_html);
return array($back, $pages, $next, 'back' => $back, 'pages' => $pages, 'next' => $next);
}
/**
* Returns number of pages
*
* @return int Number of pages
*/
function numPages()
{
return $this->_totalPages;
}
/**
* Returns whether current page is first page
*
* @return bool First page or not
*/
function isFirstPage()
{
return ($this->_currentPage == 1);
}
/**
* Returns whether current page is last page
*
* @return bool Last page or not
*/
function isLastPage()
{
return ($this->_currentPage == $this->_totalPages);
}
/**
* Returns whether last page is complete
*
* @return bool Last age complete or not
*/
function isLastPageComplete()
{
return !($this->_totalItems % $this->_perPage);
}
/**
* Calculates all page data
*/
function _generatePageData()
{
$this->_totalItems = count($this->_itemData);
$this->_totalPages = ceil((float)$this->_totalItems / (float)$this->_perPage);
$i = 1;
if (!empty($this->_itemData)) {
foreach ($this->_itemData as $value) {
$this->_pageData[$i][] = $value;
if (count($this->_pageData[$i]) >= $this->_perPage) {
$i++;
}
}
} else {
$this->_pageData = array();
}
}
/**
* Returns the correct link for the back/pages/next links
*
* @return string Url
*/
function _getLinksUrl()
{
global $HTTP_SERVER_VARS;
// Sort out query string to prevent messy urls
$querystring = array();
if (!empty($HTTP_SERVER_VARS['QUERY_STRING'])) {
$qs = explode('&', $HTTP_SERVER_VARS['QUERY_STRING']);
for ($i = 0, $cnt = count($qs); $i
list($name, $value) = explode('=', $qs[$i]);
if ($name != 'pageID') {
$qs[$name] = $value;
}
unset($qs[$i]);
}
}
if(is_array($qs)){
foreach ($qs as $name => $value) {
$querystring[] = $name . '=' . $value;
}
}
return $HTTP_SERVER_VARS['SCRIPT_NAME'] . '?' . implode('&', $querystring) . (!empty($querystring) ? '&' : ') . 'pageID=';
}
/**
* Returns back link
*
* @param $url URL to use in the link
* @param $link HTML to use as the link
* @return string The link
*/
function _getBackLink($url, $link = '
{
// Back link
if ($this->_currentPage > 1) {
$back = '' . $link . '';
} else {
$back = ';
}
return $back;
}
/**
* Returns pages link
*
* @param $url URL to use in the link
* @return string Links
*/
function _getPageLinks($url)
{
// Create the range
$params['itemData'] = range(1, max(1, $this->_totalPages));
$pager =& new Pager($params);
$links = $pager->getPageData($pager->getPageIdByOffset($this->_currentPage));
for ($i=0; $i
$links[$i] = '' . $links[$i] . '';
}
}
return implode(' ', $links);
}
/**
* Returns next link
*
* @param $url URL to use in the link
* @param $link HTML to use as the link
* @return string The link
*/
function _getNextLink($url, $link = 'Next >>')
{
if ($this->_currentPage _totalPages) {
$next = '' . $link . '';
} else {
$next = ';
}
return $next;
}
}
?>
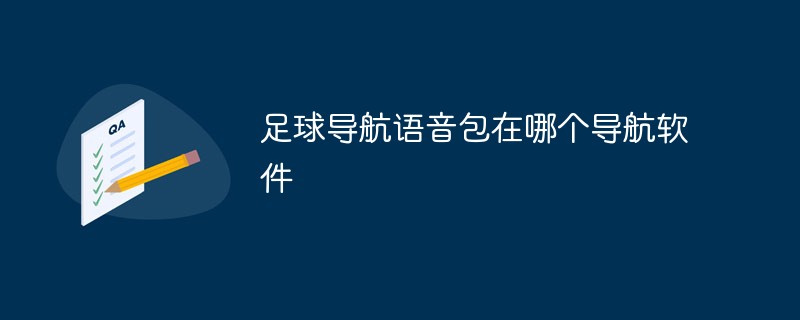
足球导航语音包在“高德导航”软件中,是高德地图车机版导航语音包的其中一种,内容为黄健翔足球解说版本的导航语音。设置方法:1、打开高德地图软件;2、点击进入“更多工具”-“导航语音”选项;3、找到“黄健翔热血语音”,点击“下载”;4、在弹出的页面,点击“使用语音”即可。
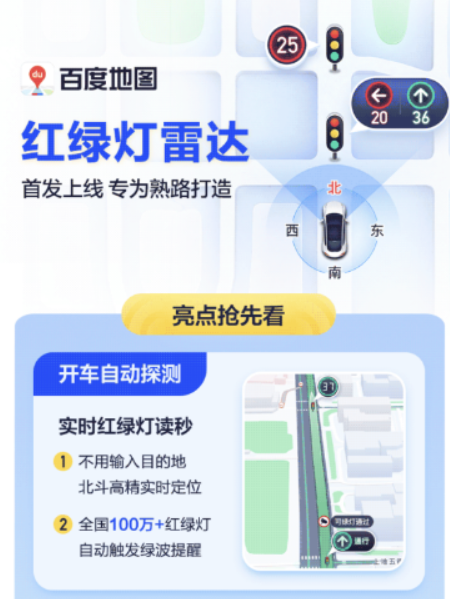
百度地图App安卓版/iOS版均已发布18.8.0版本,首次引入红绿灯雷达功能,业内领先据官方介绍,开启红绿灯雷达后,支持开车自动探测红绿灯,不用输入目的地,北斗高精可以实时定位,全国100万+红绿灯自动触发绿波提醒。除此之外,新功能还提供全程静音导航,使图区更简洁,关键信息一目了然,且无语音播报,使驾驶员更加专注驾驶百度地图于2020年10月上线红绿灯倒计时功能,支持实时读秒预判,导航会在接近红绿灯路口时,自动展示倒计时剩余秒数,让用户时刻掌握前方路况。截至2022年12月31日,红绿灯倒计时
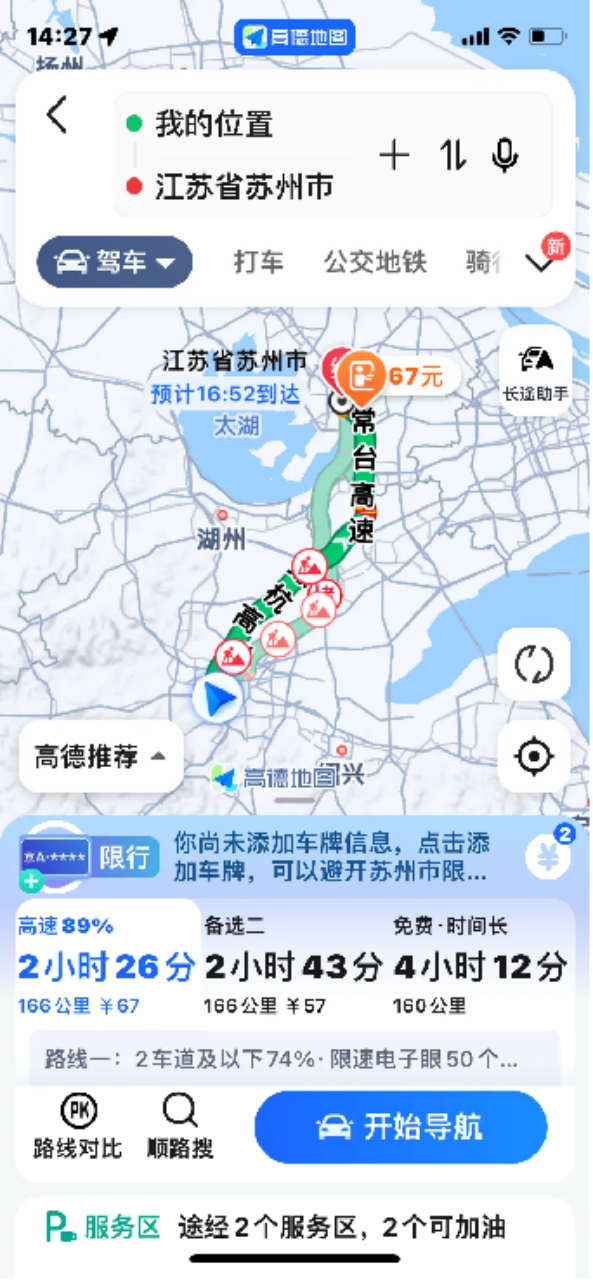
本站4月29日消息,高德地图官宣推出升级版的驾车ETA(本站注:ETA即预估到达时间,指的是用户在当前时刻出发按照给定路线前往目的地预计需要的时长)服务,该服务旨在帮助用户的路线规划时长和路况预估更为精准,辅助用户进行出行决策。该地图应用是最新升级的高德地图App,引入了“超大规模图卷积神经网络模型”,该模型可以更好地捕捉和学习交通流动规律,支持城市道路网络、高速公路系统,能以高精度刻画交通状况的时空动态变化。在此外,全新版本的地图还进一步融合了iTransformer时序预测模型,支持实时解析
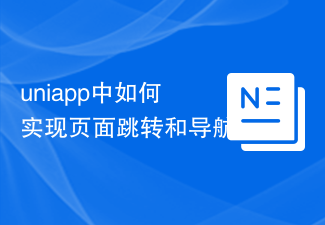
uniapp中如何实现页面跳转和导航uniapp是一款支持一次编码多端发布的前端框架,它基于Vue.js,开发者可以使用uniapp快速开发移动端应用。在uniapp中,实现页面跳转和导航是非常常见的需求。本文将介绍uniapp中如何实现页面跳转和导航,并提供具体的代码示例。一、页面跳转使用uniapp提供的方法进行页面跳转uniapp提供了一组方法用于实现
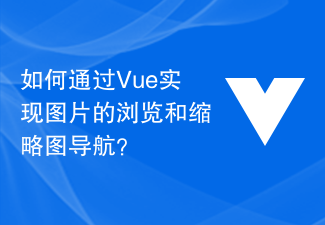
如何通过Vue实现图片的浏览和缩略图导航?随着Web应用程序的发展,图片在我们的日常生活中扮演着越来越重要的角色。在许多情况下,我们需要实现图片的浏览和缩略图导航功能。这篇文章将介绍如何利用Vue框架实现这一功能,并提供代码示例。在Vue中,我们可以使用Vue插件来实现图片的浏览和缩略图导航功能。一个流行的插件是vue-gallery,它提供了简单易用的接口
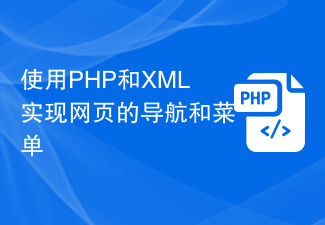
使用PHP和XML实现网页的导航和菜单导航和菜单是网页中常见的元素,它们可以使用户快速地找到所需的信息或功能。在网页开发中,PHP和XML经常被用来处理和存储导航和菜单的数据。本文将介绍如何使用PHP和XML实现网页导航和菜单,并提供相关的代码示例。一、创建XML菜单数据文件首先,我们需要创建一个XML文件来存储我们的菜单数据。以下是一个示例的XML文件,它
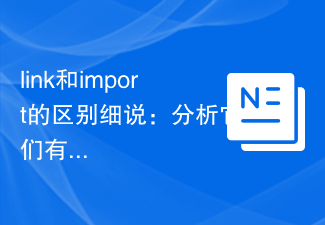
深入解析:link与import的区别是什么?在开发网页或应用程序时,我们经常需要引入外部的CSS文件或JavaScript库来增强或定制我们的代码。在这个过程中,link和import是两种常用的方法。虽然它们的目的都是引入外部资源,但在具体的使用上存在一些区别。语法和位置:link:使用link标签将外部资源链接到HTML文件中,通常位于HTML文档的头


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
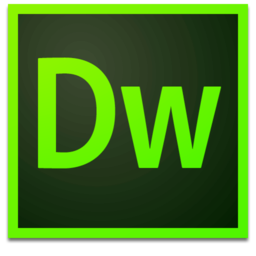
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
