How to implement dynamic titles and SEO optimization in Vue
When developing using Vue, dynamic titles and SEO optimization are an important task. In this article, we will introduce how to use Vue to implement dynamic titles and provide some SEO optimization tips and code examples.
1. Implementation of dynamic titles
When developing using Vue, single page applications (SPA) are very common. In SPA, the title will not change when the page is switched, which will affect the user experience and SEO optimization of the website.
In order to solve this problem, we can use Vue's routing and hook functions to dynamically modify the title. The specific steps are as follows:
-
Install the vue-router plug-in
npm install vue-router
-
Introduce the vue-router plug-in into Vue's main.js and configure routing
import VueRouter from 'vue-router' import Home from './Home.vue' Vue.use(VueRouter) const routes = [ { path: '/', name: 'Home', component: Home }, // 其他路由配置... ] const router = new VueRouter({ mode: 'history', routes }) new Vue({ router, render: h => h(App) }).$mount('#app')
-
Achieve dynamic titles by setting routing titles in each component
export default { name: 'Home', created() { this.$nextTick(() => { document.title = '首页' }) } }
2. SEO optimization skills
In addition to implementing dynamic titles, optimizing your website to be search engine friendly is also crucial. The following are some SEO optimization tips and code examples:
-
Use the vue-meta plug-in to set the meta tag information of the page.
npm install vue-meta
// main.js import VueMeta from 'vue-meta' Vue.use(VueMeta) // Home.vue export default { name: 'Home', metaInfo: { title: '首页', meta: [ { name: 'keywords', content: '关键词1,关键词2' }, { name: 'description', content: '页面描述' } ], link: [ { rel: 'canonical', href: '当前页面的规范URL' } ] }, created() { // ... } }
-
Prerendering
For SPA, search engine crawlers usually cannot correctly parse content dynamically generated by JavaScript. To solve this problem, we can use pre-rendering technology to render dynamically generated content into static HTML in advance and return it to the crawler through server-side rendering (SSR).
For example, you can use the prerender-spa-plugin plugin for pre-rendering.
npm install prerender-spa-plugin
// vue.config.js const PrerenderSPAPlugin = require('prerender-spa-plugin') module.exports = { configureWebpack: { plugins: [ new PrerenderSPAPlugin({ staticDir: path.join(__dirname, 'dist'), routes: ['/', '/about', '/contact'], }) ] } }
-
URL Rewriting
Use URL rewriting technology to rewrite dynamically generated URLs into static URLs, which helps search engine crawlers better index the website Content.
For example, you can use vue-router's configuration items to set URL rewriting rules.
const router = new VueRouter({ mode: 'history', routes: [ { path: '/article/:id', component: Article } ] })
<!-- Article.vue --> <template> <div class="article"> <!-- 文章内容 --> </div> </template> <script> export default { name: 'Article', created() { const articleId = this.$route.params.id // 根据文章ID获取文章内容并渲染 } } </script>
Conclusion
When using Vue for development, dynamic titles and SEO optimization are important means to improve website user experience and promotion effects. Through the above steps and techniques, we can easily implement dynamic titles and SEO optimization, thereby improving the accessibility and searchability of the website. Hope this article helps you!
The above is the detailed content of How to implement dynamic titles and SEO optimization in Vue. For more information, please follow other related articles on the PHP Chinese website!
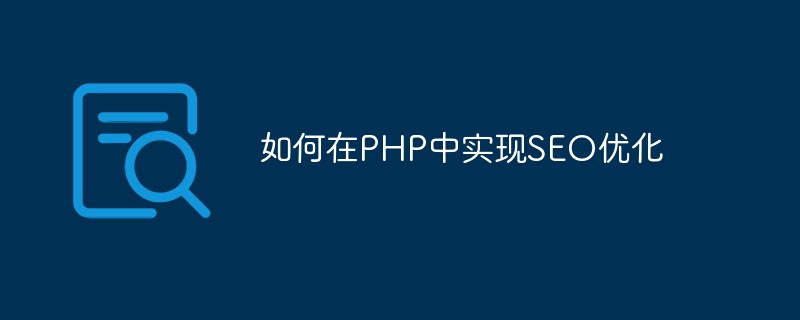
随着互联网的发展,SEO(SearchEngineOptimization,搜索引擎优化)已经成为了网站优化的重要一环。如果您想要使您的PHP网站在搜索引擎中获得更高的排名,就需要对SEO的内容有一定的了解了。本文将会介绍如何在PHP中实现SEO优化,内容包括网站结构优化、网页内容优化、外部链接优化,以及其他相关的优化技巧。一、网站结构优化网站结构对于S
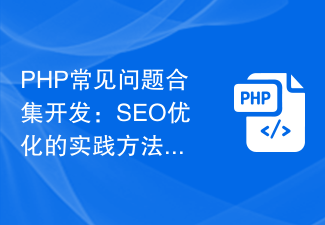
PHP常见问题合集开发:SEO优化的实践方法SEO(SearchEngineOptimization)是指通过优化网站的结构和内容,提高网站在搜索引擎中的排名,以增加网站流量和曝光度的过程。在网站开发中,优化网站的SEO是一项重要的工作。PHP(PHP:HypertextPreprocessor)是一种广泛使用的服务器端脚本语言,被广泛应用于网站开发
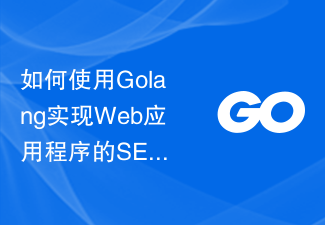
SEO(SearchEngineOptimization,搜索引擎优化)是提高网站在搜索引擎结果页面中排名的策略和技术,而Web应用程序的SEO优化则是指如何使Web应用程序能更好地被搜索引擎识别和展示。使用Golang编写Web应用程序时,如何实现SEO优化是每个开发者都需要关注的问题。下面将介绍一些使用Golang实现Web应用程序的SEO优化的方法
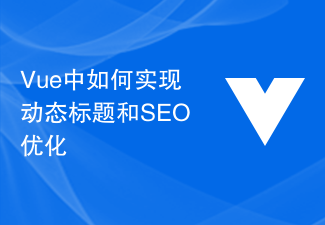
Vue中如何实现动态标题和SEO优化在使用Vue进行开发时,动态标题和SEO优化是一项重要的任务。在这篇文章中,我们将介绍如何使用Vue来实现动态标题,并提供一些SEO优化的技巧和代码示例。一、动态标题的实现在使用Vue进行开发时,单页面应用(SPA)是非常常见的。在SPA中,页面切换时标题不会随之改变,这会影响网站的用户体验和SEO优化。为了解决这个问题,
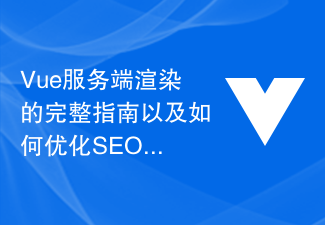
Vue服务端渲染是一种使得Web开发更加高效、可靠和快速的解决方案。它使得Vue框架能够在服务器上预处理组件,并在将其传输到浏览器之前直接输出HTML。这意味着Vue服务端渲染可以提高网站的性能并且优化SEO,因为搜索引擎可以使用呈现的HTML来更好地索引内容。本文将介绍Vue服务端渲染的完整指南以及如何优化SEO。Vue服务端渲染的工作原理Vue服务端渲染
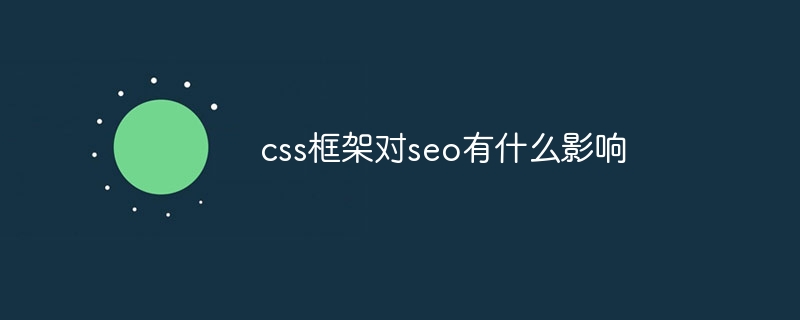
CSS框架对SEO有一定的影响,可以影响网站的加载速度、影响网站的响应式设计、影响网站的可访问性和影响网站的结构和语义化。开发人员应该选择轻量级、响应式、可访问性强、结构良好的CSS框架,并进行适当的优化和测试,以确保网站在搜索引擎结果页面上获得良好的排名。同时,开发人员还应该遵循SEO最佳实践,以提高网站的可见性和用户体验 。
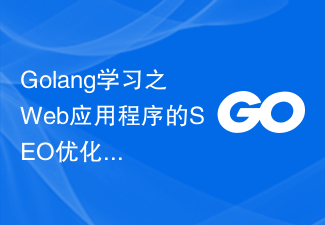
在当今互联网时代,网站的SEO优化已经成为了网站经营者们必须要面对的问题。而对于使用Golang语言开发Web应用程序的开发者们来说,如何进行SEO优化又是一个新的挑战。那么究竟该如何进行GolangWeb应用程序的SEO优化呢?在本文中,我们将为大家分享一些实践经验和技巧。URL结构优化在进行SEO优化时,一个清晰、简洁、易于理解的URL结构不仅可以帮助
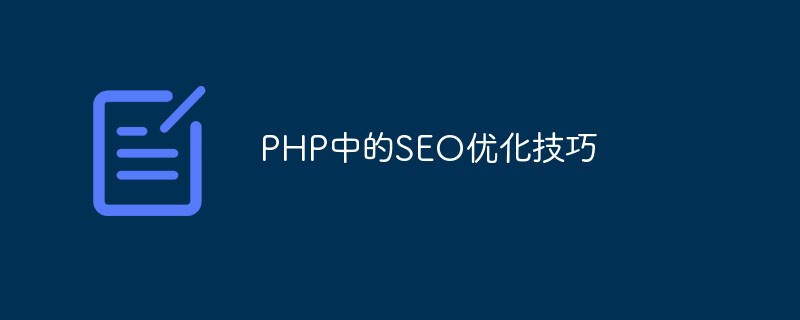
随着互联网发展的不断深入,越来越多的企业开始重视SEO优化技巧,以提高自己的品牌知名度和业务流量。其中,PHP是一种常用的编程语言,几乎所有的网站都使用它来开发和维护自己的网站。因此,在PHP中使用一些简单易学而又好用的SEO优化技巧,可以有效地提高网站在搜索引擎中的排名。本篇文章将为大家介绍一些常用的PHP中的SEO优化技巧,帮助大家提高网站排名。一、使用


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
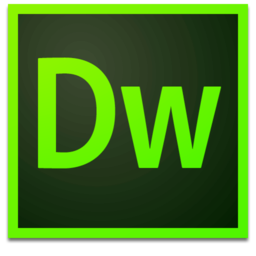
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
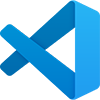
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
