


Annotation-based route parsing and dynamic controller loading implementation in PHP
Annotation-based routing resolution and dynamic controller loading implementation in PHP
As the complexity of web applications continues to increase, routing management and controller loading It has become an important link in the development process. The traditional route resolution and controller loading methods require manual configuration of routing rules and controller classes, and require frequent updates and maintenance, which can become very cumbersome and inefficient in large applications.
In order to solve this problem, annotations can be used to simplify route parsing and dynamic controller loading. Annotation is a technology that adds relevant metadata to the code. The mapping relationship between routing rules and controller classes can be written directly in the controller class, so that the corresponding controller class can be automatically loaded during the routing process.
1. Implement the route parsing function
To implement the annotation-based route parsing function in PHP, you need to use reflection to obtain the annotation information of the controller class. The following is a simple implementation example:
class Router { public function dispatch($url) { $controller = $this->parseUrl($url); // 根据控制器类名称实例化对象 $controllerObject = new $controller; // 执行控制器方法 $controllerObject->execute(); } private function parseUrl($url) { // 解析URL,获取控制器类名称 // 这里假设URL的格式为 /controller/method // 如 /user/save 表示UserController的save方法 $parts = explode('/', $url); $controller = ucfirst($parts[1]).'Controller'; return $controller; } } class UserController { /** * @Route("/user/save") */ public function save() { // 实现保存用户的逻辑 } } // 创建Router对象并分发路由 $router = new Router(); $router->dispatch('/user/save');
In the above example, the route resolution process is implemented by parsing the URL, assuming that the format of the URL is /controller/method. The annotation @Route is used in the controller class UserController to specify routing rules. When the dispatch method is called and '/user/save' is passed in, the resolved controller class name is UserController, and the object is instantiated and the save method is executed.
2. Implement dynamic controller loading function
In order to dynamically load controller classes, we need to use PHP's automatic loading mechanism. The following is a simple implementation example:
class Autoloader { public static function autoload($className) { // 将命名空间中的替换为目录分隔符/ $className = str_replace('\', DIRECTORY_SEPARATOR, $className); // 根据类名加载文件 require_once __DIR__ . '/controllers/' . $className . '.php'; } } // 注册自动加载函数 spl_autoload_register('Autoloader::autoload'); // 创建Router对象并分发路由 $router = new Router(); $router->dispatch('/user/save');
In the above example, we created an Autoloader class, in which the autoload method dynamically loads the corresponding file based on the class name. After this, use the spl_autoload_register function to register the autoload method as an autoload function. In this way, when the dispatch method is called, the corresponding controller class file will be automatically loaded based on the controller class name parsed from the annotation.
Through the above implementation, we can simplify the process of route parsing and controller loading, and reduce the maintenance work of configuration files. At the same time, annotation-based route parsing and dynamic controller loading also improve the readability and maintainability of the code, making the development process more efficient and flexible.
The above is the detailed content of Annotation-based route parsing and dynamic controller loading implementation in PHP. For more information, please follow other related articles on the PHP Chinese website!
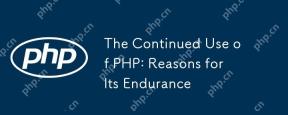
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
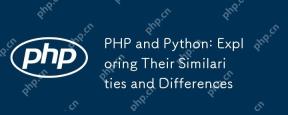
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
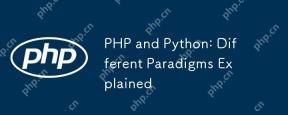
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
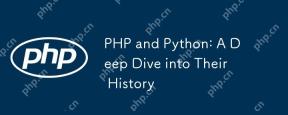
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
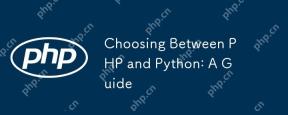
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
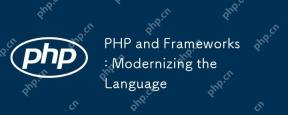
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
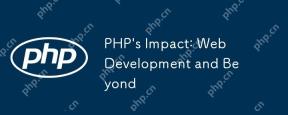
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
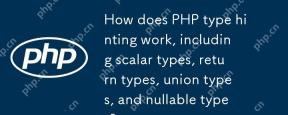
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
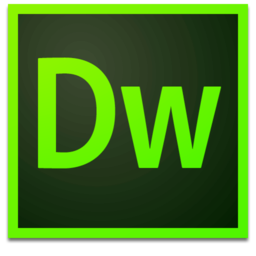
Dreamweaver Mac version
Visual web development tools
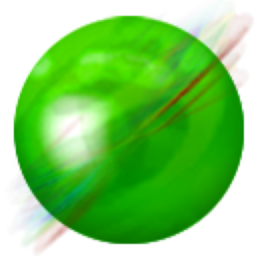
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
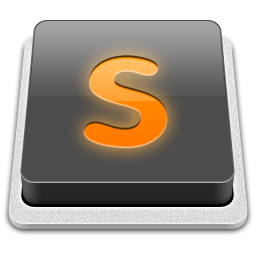
SublimeText3 Mac version
God-level code editing software (SublimeText3)