


Implement distributed task scheduling using RPC services developed by ThinkPHP6 and Swoole
Title: Using RPC services developed by ThinkPHP6 and Swoole to implement distributed task scheduling
Introduction:
With the rapid development of the Internet, more and more Applications need to handle a large number of tasks, such as scheduled tasks, queue tasks, etc. The traditional single-machine task scheduling method can no longer meet the needs of high concurrency and high availability. This article will introduce how to use ThinkPHP6 and Swoole to develop an RPC service to implement distributed task scheduling and processing to improve task processing efficiency and reliability.
1. Environment preparation:
Before we start, we need to install and configure the following development environment:
- PHP environment (it is recommended to use PHP7.2 or above)
- Composer (used to install and manage ThinkPHP6 and Swoole libraries)
- MySQL database (used to store task information)
- Swoole extension library (used to implement RPC services)
2. Project creation and configuration:
-
Create a project:
Use Composer to create a ThinkPHP6 project and execute the following command:composer create-project topthink/think your_project_name
-
Configure database connection:
Edit the.env
file in the project directory and configure the database connection information, for example:DATABASE_CONNECTION=mysql DATABASE_HOST=127.0.0.1 DATABASE_PORT=3306 DATABASE_DATABASE=your_database_name DATABASE_USERNAME=your_username DATABASE_PASSWORD=your_password
-
Establish Database table:
Execute the database migration command of ThinkPHP6 to generate the migration file of the task table and scheduling log table:php think migrate:run
Edit the generated migration file and create the structure of the task table and scheduling log table. For example, the task table structure is as follows:
<?php namespace appmigration; use thinkmigrationMigrator; use thinkmigrationdbColumn; class CreateTaskTable extends Migrator { public function up() { $table = $this->table('task'); $table->addColumn('name', 'string', ['comment' => '任务名称']) ->addColumn('content', 'text', ['comment' => '任务内容']) ->addColumn('status', 'integer', ['default' => 0, 'comment' => '任务状态']) ->addColumn('create_time', 'timestamp', ['default' => 'CURRENT_TIMESTAMP', 'comment' => '创建时间']) ->addColumn('update_time', 'timestamp', ['default' => 'CURRENT_TIMESTAMP', 'update' => 'CURRENT_TIMESTAMP', 'comment' => '更新时间']) ->create(); } public function down() { $this->dropTable('task'); } }
Execute the
php think migrate:run
command to synchronize the task table structure to the database.
3. Write RPC service:
-
Install the Swoole extension library:
Execute the following command to install the Swoole extension library:pecl install swoole
-
Create RPC service:
Create aserver
folder in the project directory to store RPC service-related code. Create aRpcServer.php
file in this folder and write the code for the RPC service. The example is as follows:<?php namespace appserver; use SwooleHttpServer; use SwooleWebSocketServer as WebSocketServer; class RpcServer { private $httpServer; private $rpcServer; private $rpc; public function __construct() { $this->httpServer = new Server('0.0.0.0', 9501); $this->httpServer->on('request', [$this, 'handleRequest']); $this->rpcServer = new WebSocketServer('0.0.0.0', 9502); $this->rpcServer->on('open', [$this, 'handleOpen']); $this->rpcServer->on('message', [$this, 'handleMessage']); $this->rpcServer->on('close', [$this, 'handleClose']); $this->rpc = new ppcommonRpc(); } public function start() { $this->httpServer->start(); $this->rpcServer->start(); } public function handleRequest($request, $response) { $this->rpc->handleRequest($request, $response); } public function handleOpen($server, $request) { $this->rpc->handleOpen($server, $request); } public function handleMessage($server, $frame) { $this->rpc->handleMessage($server, $frame); } public function handleClose($server, $fd) { $this->rpc->handleClose($server, $fd); } }
-
Create the RPC class:
In the project directory Create acommon
folder to store public class library files. Create aRpc.php
file under this folder and write the code for RPC processing. The example is as follows:<?php namespace appcommon; use SwooleHttpRequest; use SwooleHttpResponse; use SwooleWebSocketServer; use SwooleWebSocketFrame; class Rpc { public function handleRequest(Request $request, Response $response) { // 处理HTTP请求的逻辑 } public function handleOpen(Server $server, Request $request) { // 处理WebSocket连接建立的逻辑 } public function handleMessage(Server $server, Frame $frame) { // 处理WebSocket消息的逻辑 } public function handleClose(Server $server, $fd) { // 处理WebSocket连接关闭的逻辑 } public function handleTask($frame) { // 处理任务的逻辑 } }
4. Implement task scheduling:
InRpc.php In the
handleRequestmethod in the
file, add task scheduling logic to the logic of processing HTTP requests. For example, the code for processing scheduled POST requests is as follows:public function handleRequest(Request $request, Response $response) { if ($request->server['request_method'] == 'POST') { // 解析请求参数 $data = json_decode($request->rawContent(), true); // 写入任务表 $task = new ppindexmodelTask(); $task->name = $data['name']; $task->content = $data['content']; $task->status = 0; $task->save(); $this->handleTask($data); // 返回调度成功的响应 $response->end(json_encode(['code' => 0, 'msg' => '任务调度成功'])); } else { // 返回不支持的请求方法响应 $response->end(json_encode(['code' => 1, 'msg' => '不支持的请求方法'])); } }
In the above code, we first parse the content of the request and write the task information into the task table. Then call the
handleTask
method to handle the logic of the task, such as RPC clients sent to other servers.
Summary:
This article introduces the steps and code examples for implementing distributed task scheduling using RPC services developed by ThinkPHP6 and Swoole. By using RPC services, we can implement distributed scheduling and processing of tasks and improve task processing efficiency and reliability. I hope this article can help you understand and practice distributed task scheduling.
The above is the detailed content of Implement distributed task scheduling using RPC services developed by ThinkPHP6 and Swoole. For more information, please follow other related articles on the PHP Chinese website!
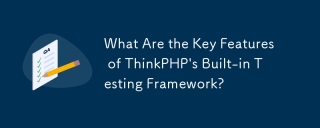
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
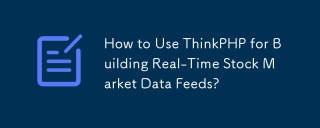
Article discusses using ThinkPHP for real-time stock market data feeds, focusing on setup, data accuracy, optimization, and security measures.

The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
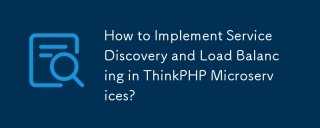
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
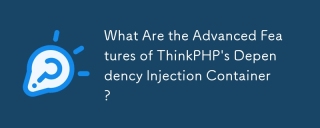
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
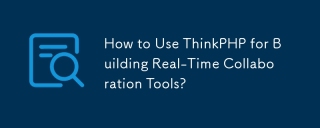
The article discusses using ThinkPHP to build real-time collaboration tools, focusing on setup, WebSocket integration, and security best practices.
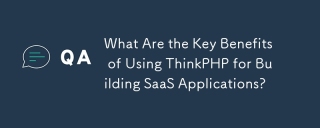
ThinkPHP benefits SaaS apps with its lightweight design, MVC architecture, and extensibility. It enhances scalability, speeds development, and improves security through various features.
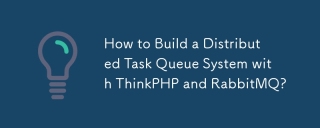
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
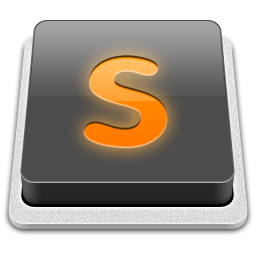
SublimeText3 Mac version
God-level code editing software (SublimeText3)