


RPC service based on ThinkPHP6 and Swoole to implement service routing and load balancing
RPC service based on ThinkPHP6 and Swoole to implement service routing and load balancing
Introduction:
With the rapid development of the Internet, distributed systems have become increasingly important . When our system needs to scale horizontally, the RPC (Remote Procedure Call)-based approach is a good choice. RPC allows us to easily split services into independent modules and communicate over the network. This article will introduce how to use ThinkPHP6 and Swoole to implement RPC-based service routing and load balancing.
1. Environment setup
Before we start, we need to prepare the following environment:
- PHP: Make sure your system has PHP installed and the version is higher than 7.3.
- Composer: Composer is a dependency management tool for PHP. Please make sure you have Composer installed.
- Swoole extension: We need to install the Swoole extension, which can be installed through the
composer require swoole/swoole
command.
2. Overview
Our goal is to build an RPC service based on ThinkPHP6 and Swoole so that different modules can communicate through RPC. In order to achieve load balancing, we will use the HTTP Server provided by Swoole to act as a routing server to distribute requests to back-end service nodes.
3. Create HTTP Server
First, we need to create a Swoole HTTP Server to act as a routing server. Create a rpc_server.php
file in the root directory of your project and write the following code:
use SwooleHttpServer; use SwooleHttpRequest; use SwooleHttpResponse; $http = new Server("0.0.0.0", 9501); $http->on('request', function (Request $request, Response $response) { // 处理请求并分发到对应的服务节点 }); $http->start();
4. Implement the RPC service
Next, we need to create the RPC service. We use ThinkPHP6 as the framework and initiate RPC requests through Swoole's CoroutineHttpClient
.
First, create a Rpc
directory in the project, and create a Service
directory under this directory to store the code of the service node. Create a TestService.php
file in the Service
directory and write the following code:
namespace apppcservice; class TestService { public function test() { return 'Hello, World!'; } }
Next, create a Rpc
directory Server.php
file, and write the following code:
namespace apppc; class Server { public function handle($request) { // 解析请求,获取要调用的服务和方法 $service = $request['service']; $method = $request['method']; // 根据服务名调用对应的服务节点 $className = '\app\rpc\service\'.$service; $instance = new $className(); $result = $instance->$method(); // 返回结果 return $result; } }
5. Process the request in the routing server
Now we can go back to the rpc_server.php
file , write the code to handle the request in the handleRequest
function. We need to parse the service name and method name in the request and forward the request to the corresponding RPC service node. The code is as follows:
use SwooleHttpServer; use SwooleHttpRequest; use SwooleHttpResponse; $http = new Server("0.0.0.0", 9501); $http->on('request', function (Request $request, Response $response) { $requestData = json_decode($request->rawContent(), true); // 解析服务名和方法名 $service = $requestData['service']; $method = $requestData['method']; // 转发请求给对应的RPC服务节点 $client = new SwooleCoroutineHttpClient('127.0.0.1', 9502); $client->post('/rpc', json_encode($requestData)); $response->end($client->body); }); $http->start();
6. Configure routing and load balancing
Finally, we need to configure routing and load balancing. Write the following code in the rpc_server.php
file:
use SwooleHttpServer; use SwooleHttpRequest; use SwooleHttpResponse; use SwooleCoroutineHttpClient; $http = new Server("0.0.0.0", 9501); $http->on('request', function (Request $request, Response $response) { // 路由配置,可以根据请求的URL中的信息进行路由和负载均衡选择 $routes = [ '/test' => [ 'host' => '127.0.0.1', 'port' => 9502, ], ]; // 获取请求路径,并根据路径选择对应的服务节点 $path = $request->server['path_info']; $route = $routes[$path]; // 转发请求给对应的RPC服务节点 $client = new Client($route['host'], $route['port']); $client->post('/rpc', $request->rawContent()); $response->end($client->body); }); $http->start();
7. Test
Now, we can test. Start the routing server and RPC service node, and visit http://localhost:9501/test
in the browser. You will see that the returned result is "Hello, World!".
8. Summary
This article introduces how to use ThinkPHP6 and Swoole to implement RPC-based service routing and load balancing. Through Swoole's HTTP Server and CoroutineHttpClient, we can easily build a distributed system that supports RPC communication. Hope this article is helpful to you.
The above is the detailed content of RPC service based on ThinkPHP6 and Swoole to implement service routing and load balancing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
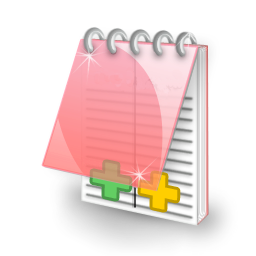
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use
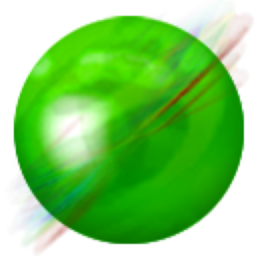
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
