How to solve the problem of concurrency competition in Java requires specific code examples
Introduction: In multi-thread programming, a problem we often encounter is concurrency competition. . When multiple threads access shared resources at the same time, data inconsistency or deadlock may occur. In Java, some mechanisms and tools are provided to solve these problems. This article will introduce in detail how to solve the concurrency competition problem in Java and give specific code examples.
1. Use the synchronized keyword
The synchronized keyword is one of the most basic methods provided by Java to solve the problem of concurrency competition. The synchronized keyword can be used to mark a method or code block as synchronized. Only one thread can access the method or code block at the same time, and other threads need to wait.
Code example:
public class Example { private int count = 0; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } }
In the above code, the increment() method and getCount() method are marked as synchronized, ensuring that only one thread can access these two at the same time. method. This solves the problem of concurrency competition.
2. Use the ReentrantLock class
In addition to using the synchronized keyword, Java also provides the ReentrantLock class to resolve concurrency competition. The ReentrantLock class is a reentrant mutex lock that can replace the synchronized keyword to synchronize access to shared resources.
Code example:
import java.util.concurrent.locks.ReentrantLock; public class Example { private int count = 0; private ReentrantLock lock = new ReentrantLock(); public void increment() { lock.lock(); try { count++; } finally { lock.unlock(); } } public int getCount() { lock.lock(); try { return count; } finally { lock.unlock(); } } }
In the above code, use the ReentrantLock class to achieve synchronous access to count. In the increment() method and getCount() method, the lock is acquired and released by calling the lock() method and unlock() method. This ensures that only one thread can access these methods at the same time, solving the problem of concurrency competition.
3. Use Atomic classes
In addition to using locks to achieve synchronous access to shared resources, Java also provides some atomic classes, such as AtomicInteger, AtomicLong, etc., which can directly operate the underlying memory , implement atomic operations on shared resources and avoid race conditions.
Code example:
import java.util.concurrent.atomic.AtomicInteger; public class Example { private AtomicInteger count = new AtomicInteger(0); public void increment() { count.incrementAndGet(); } public int getCount() { return count.get(); } }
In the above code, use the AtomicInteger class to replace the int type count, and atomically increase and get the count through the incrementAndGet() method and get() method value. This avoids race conditions and solves the problem of concurrency competition.
Summary: In Java, we can use the synchronized keyword, ReentrantLock class and Atomic class to solve the problem of concurrency competition. The specific choice depends on the actual needs and scenarios. This article gives specific code examples, hoping to help readers better understand and solve concurrency competition problems in Java.
The above is the detailed content of How to solve concurrency race issues in Java. For more information, please follow other related articles on the PHP Chinese website!
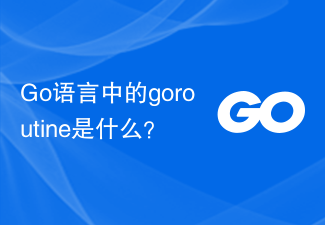
Go语言是一种开源编程语言,由Google开发并于2009年面世。这种语言在近年来越发受到关注,并被广泛用于开发网络服务、云计算等领域。Go语言最具特色的特点之一是它内置了goroutine(协程),这是一种轻量级的线程,可以在代码中方便地实现并发和并行计算。那么goroutine到底是什么呢?简单来说,goroutine就是Go语言中的
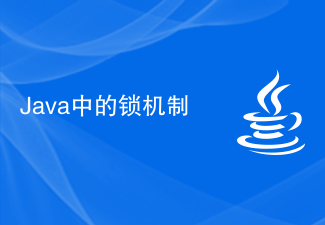
Java作为一种高级编程语言,在并发编程中有着广泛的应用。在多线程环境下,为了保证数据的正确性和一致性,Java采用了锁机制。本文将从锁的概念、类型、实现方式和使用场景等方面对Java中的锁机制进行探讨。一、锁的概念锁是一种同步机制,用于控制多个线程之间对共享资源的访问。在多线程环境下,线程的执行是并发的,多个线程可能会同时修改同一数据,这就会导致数
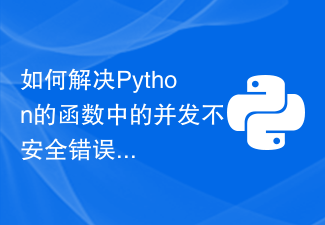
Python是一门流行的高级编程语言,它具有简单易懂的语法、丰富的标准库和开源社区的支持,而且还支持多种编程范式,例如面向对象编程、函数式编程等。尤其是Python在数据处理、机器学习、科学计算等领域有着广泛的应用。然而,在多线程或多进程编程中,Python也存在一些问题。其中之一就是并发不安全。本文将从以下几个方面介绍如何解决Python的函数中的并发不安
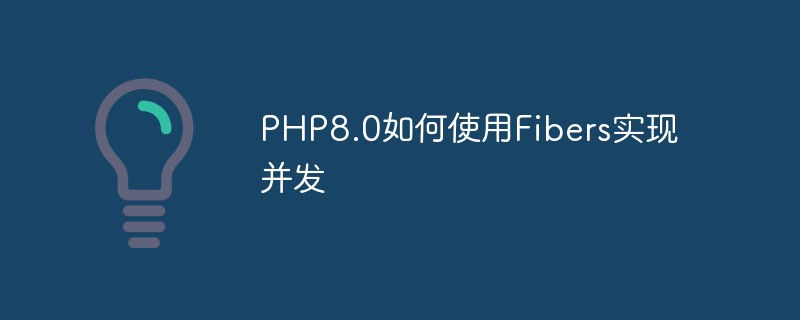
随着现代互联网技术的不断发展,网站访问量越来越大,对于服务器的并发处理能力也提出了更高的要求。如何提高服务器的并发处理能力是每个开发者需要面对的问题。在这个背景下,PHP8.0引入了Fibers这一全新的特性,让PHP开发者掌握一种全新的并发处理方式。Fibers是什么?首先,我们需要了解什么是Fibers。Fibers是一种轻量级的线程,可以高效地支持PH
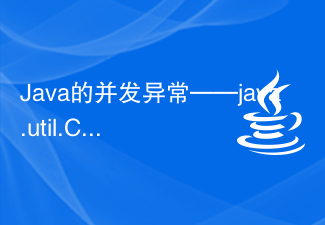
Java作为一种高级语言,在编程语言中使用广泛。在Java的应用程序和框架的开发中,我们经常会碰到并发的问题。并发问题是指当多个线程同时对同一个对象进行操作时,会产生一些意想不到的结果,这些问题称为并发问题。其中的一个常见的异常就是java.util.ConcurrentModificationException异常,那么我们在开发过程中如何有效地解决这个异
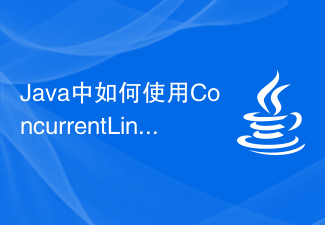
Java中的ConcurrentLinkedQueue函数为开发者提供了一种线程安全的、高效的队列实现方式,它支持并发读写操作,并且执行效率较高。在本文中,我们将介绍Java中如何使用ConcurrentLinkedQueue函数进行并发队列操作,帮助开发者更好地利用其优势。ConcurrentLinkedQueue是Java中的一个线程安全、非阻塞的队列实
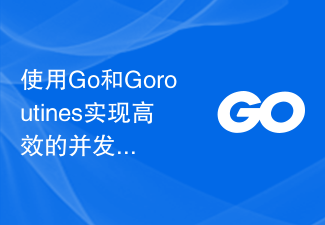
使用Go和Goroutines实现高效的并发图计算引言:随着大数据时代的到来,图计算问题也成为了一个热门的研究领域。在图计算中,图的顶点和边之间的关系非常复杂,因此如果采用传统的串行方法进行计算,往往会遇到性能瓶颈。为了提高计算效率,我们可以利用并发编程的方法使用多个线程同时进行计算。今天我将向大家介绍使用Go和Goroutines实现高效的并发图计算的方法
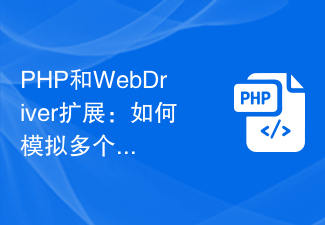
PHP和WebDriver扩展:如何模拟多个用户的并发访问随着互联网的快速发展,网站的访问量也越来越大,很多场景下需要测试网站在高并发情况下的表现。本文将介绍如何使用PHP和WebDriver扩展来模拟多个用户的并发访问,并提供相应的代码示例。首先,我们需要安装并配置PHP和WebDriver扩展。PHP是一种流行的服务器端脚本语言,而WebDriver是一


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
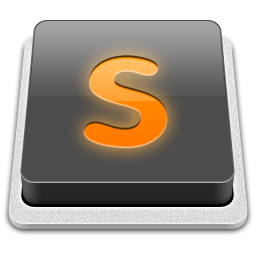
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
