


How to solve the problem of task monitoring and alarm handling of concurrent tasks in Go language?
How to solve the problem of task monitoring and alarm processing of concurrent tasks in Go language?
When using Go language for concurrent programming, we often encounter problems with task monitoring and alarm handling. The monitoring of concurrent tasks is to understand the execution status of the tasks in a timely manner, while the alarm processing is to promptly notify when an abnormality occurs in the tasks so that timely measures can be taken. This article will introduce how to solve the problem of task monitoring and alarm handling of concurrent tasks in the Go language, and provide specific code examples.
1. Task monitoring
The Go language provides a variety of ways to monitor the execution of tasks. Several common methods will be introduced below.
- Monitor the startup and completion of tasks through counters
When using the Go language for concurrent programming, you can monitor the startup and completion of tasks by using counters. Whenever a task is started, the counter is incremented by 1; whenever a task is completed, the counter is decremented by 1. By monitoring the value of the counter, you can understand the execution status of the task in real time.
The following is a sample code that uses counters to monitor tasks:
package main import ( "fmt" "sync" ) var wg sync.WaitGroup func main() { numTasks := 5 // 设置计数器的值为任务的数量 wg.Add(numTasks) // 启动多个任务 for i := 0; i < numTasks; i++ { go processTask(i) } // 等待所有任务完成 wg.Wait() fmt.Println("All tasks completed!") } func processTask(taskNum int) { fmt.Println("Task", taskNum, "started") // 模拟任务的耗时操作 // ... fmt.Println("Task", taskNum, "completed") // 任务完成,计数器减1 wg.Done() }
- Monitoring the startup and completion of tasks through channels
In addition to using counters to monitor In addition to the start and completion of tasks, channels can also be used to monitor. When a task starts, a signal is sent to the channel; when the task completes, a signal is received from the channel. By monitoring the signal of the channel, the execution status of the task can be understood in real time.
The following is a sample code for using channel monitoring tasks:
package main import ( "fmt" "sync" ) var wg sync.WaitGroup func main() { numTasks := 5 // 创建一个通道用于监控任务的完成情况 doneCh := make(chan struct{}) // 启动多个任务 for i := 0; i < numTasks; i++ { wg.Add(1) go processTask(i, doneCh) } // 等待所有任务完成 wg.Wait() fmt.Println("All tasks completed!") } func processTask(taskNum int, doneCh chan struct{}) { fmt.Println("Task", taskNum, "started") // 模拟任务的耗时操作 // ... fmt.Println("Task", taskNum, "completed") // 任务完成,向通道发送信号 doneCh <- struct{}{} // 任务完成,计数器减1 wg.Done() }
2. Alarm processing
When an exception occurs in a task, alarm processing needs to be carried out in a timely manner to effectively solve the problem question. The following will introduce how to use channels and select statements in the Go language to solve the problem of alarm processing.
- Use the channel to transmit error information
During the execution of the task, if an abnormal situation is encountered, the error information can be transmitted through the channel for alarm processing. Error information can be encapsulated into a structure, containing information such as task number and error description.
The following is a sample code that uses channels to transmit error information:
package main import ( "fmt" "sync" ) type ErrorInfo struct { TaskNum int Message string } var wg sync.WaitGroup func main() { numTasks := 5 // 创建一个通道用于传递错误信息 errorCh := make(chan ErrorInfo) // 启动多个任务 for i := 0; i < numTasks; i++ { wg.Add(1) go processTask(i, errorCh) } // 等待所有任务完成 wg.Wait() // 关闭通道,防止死锁 close(errorCh) // 处理错误信息 for err := range errorCh { fmt.Printf("Task %d error: %s ", err.TaskNum, err.Message) // 进行报警处理 // ... } fmt.Println("All tasks completed!") } func processTask(taskNum int, errorCh chan ErrorInfo) { fmt.Println("Task", taskNum, "started") // 模拟任务的耗时操作 // ... // 任务出现异常,向通道发送错误信息 errorCh <- ErrorInfo{ TaskNum: taskNum, Message: "Task encountered an error", } fmt.Println("Task", taskNum, "completed") // 任务完成,计数器减1 wg.Done() }
- Use the select statement to monitor multiple channels
During the alarm processing process, You may need to listen to multiple channels at the same time to handle different events in a timely manner. You can use the select statement of the Go language to monitor multiple channels. Once an event occurs, handle it accordingly.
The following is a sample code that uses the select statement to monitor multiple channels:
package main import ( "fmt" "sync" "time" ) var wg sync.WaitGroup func main() { numTasks := 5 // 创建一个通道用于传递错误信息 errorCh := make(chan int) // 创建一个通道用于定时器事件 ticker := time.NewTicker(time.Second) // 启动多个任务 for i := 0; i < numTasks; i++ { wg.Add(1) go processTask(i, errorCh) } // 启动报警处理协程 go alertHandler(errorCh, ticker) // 等待所有任务完成 wg.Wait() fmt.Println("All tasks completed!") } func processTask(taskNum int, errorCh chan int) { fmt.Println("Task", taskNum, "started") // 模拟任务的耗时操作 // ... // 任务出现异常,向通道发送错误信息 if taskNum == 3 { errorCh <- taskNum } fmt.Println("Task", taskNum, "completed") // 任务完成,计数器减1 wg.Done() } func alertHandler(errorCh chan int, ticker *time.Ticker) { for { select { case taskNum := <-errorCh: fmt.Printf("Task %d encountered an error! ", taskNum) // 进行报警处理 // ... case <-ticker.C: fmt.Println("Tick") // 定时器事件处理 // ... } } }
The above is a method and code example on how to solve the problem of task monitoring and alarm processing of concurrent tasks in the Go language. By properly setting up task monitoring and alarm processing, the reliability and stability of concurrent tasks can be improved. Hope this article helps you!
The above is the detailed content of How to solve the problem of task monitoring and alarm handling of concurrent tasks in Go language?. For more information, please follow other related articles on the PHP Chinese website!
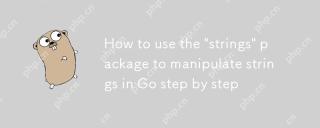
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
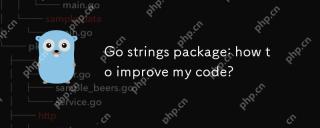
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
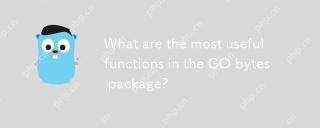
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
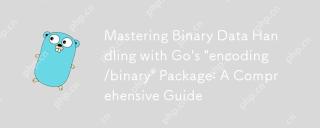
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
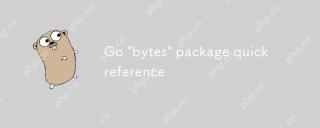
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
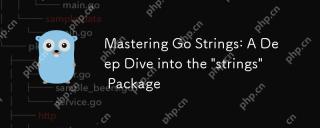
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
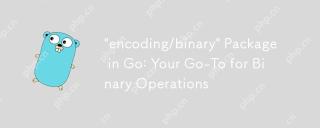
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
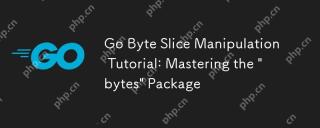
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
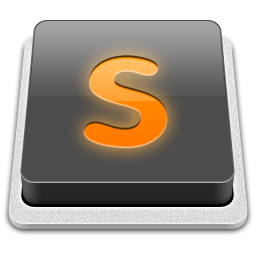
SublimeText3 Mac version
God-level code editing software (SublimeText3)
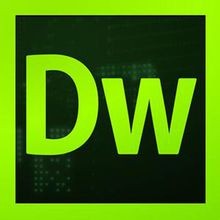
Dreamweaver CS6
Visual web development tools
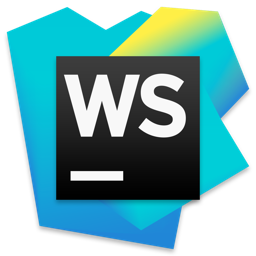
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
