


How to solve the problem of request authentication and authorization processing of concurrent network requests in Go language?
How to solve the problem of request authentication and authorization processing of concurrent network requests in Go language?
With the rapid development of the Internet, network requests play a very important role in our daily development. However, as the system scale expands and concurrency increases, request authentication and authorization issues gradually become more complex. In this article, we will explore how to solve the problem of request authentication and authorization processing of concurrent network requests in the Go language.
1. Request authentication
Network request authentication is to confirm whether the identity of the request initiator is legal. In a multi-user system, we need to control user access rights through authentication and authorization. The following is a sample code that demonstrates how to perform request authentication in Go language:
package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/hello", authMiddleware(helloHandler)) http.ListenAndServe(":8080", nil) } func authMiddleware(next http.HandlerFunc) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { // 在认证中添加具体的认证逻辑 valid := true // 暂时假设认证通过 if !valid { w.WriteHeader(http.StatusUnauthorized) return } next.ServeHTTP(w, r) } } func helloHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World") }
In the above example, we defined the authMiddleware function as the middleware for request authentication. In it, we can add specific authentication logic. If the authentication passes, we continue processing the next middleware or request processing function; if the authentication fails, we return an unauthorized HTTP status code.
2. Request authorization
After request authentication, we need to authorize the user to confirm whether he or she has the requested permissions. The following is a sample code that demonstrates how to request authorization in Go language:
package main import ( "fmt" "net/http" ) type role int const ( Admin role = iota // 管理员角色 Editor // 编辑角色 ) func main() { http.HandleFunc("/edit", authorizeMiddleware([]role{Admin, Editor}, editHandler)) http.ListenAndServe(":8080", nil) } func authorizeMiddleware(roles []role, next http.HandlerFunc) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { // 在授权中添加具体的授权逻辑 userRole := role(0) // 假设当前用户为管理员 authorized := false for _, r := range roles { if r == userRole { authorized = true break } } if !authorized { w.WriteHeader(http.StatusForbidden) return } next.ServeHTTP(w, r) } } func editHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Edit page") }
In the above example, we defined the authorizeMiddleware function as the middleware for request authorization. In it, we can add specific authorization logic. If the authorization passes, we continue to process the next middleware or request processing function; if the authorization fails, we return an access-denied HTTP status code.
Summary:
Through the above example code, we can see that it is very simple to solve the problem of request authentication and authorization processing of concurrent network requests in the Go language. We can implement the logic of request authentication and request authorization by writing middleware. As the system expands and the business develops, we can expand the functions of these middleware according to actual needs. At the same time, we can also combine different middleware to meet the scenario requirements of different roles and permissions.
Of course, the above code is just a simple example. In actual development, we also need to consider issues such as the security of request authentication, the flexibility of request authorization, and the performance of concurrent requests. At the same time, we can also use third-party libraries to simplify development work, such as gin, gorilla/mux, etc. Through careful design and reasonable planning, we can better solve the problem of request authentication and authorization processing of concurrent network requests.
The above is the detailed content of How to solve the problem of request authentication and authorization processing of concurrent network requests in Go language?. For more information, please follow other related articles on the PHP Chinese website!
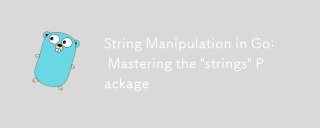
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
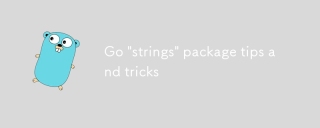
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
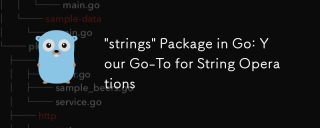
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
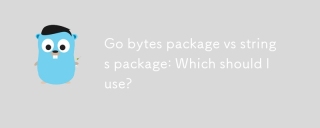
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
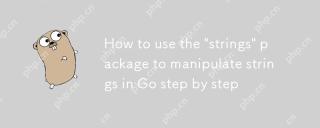
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
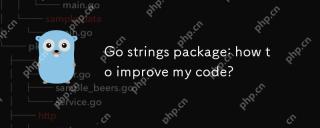
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
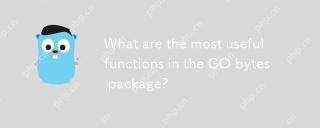
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
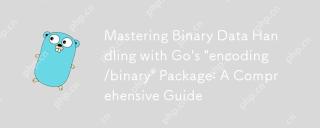
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
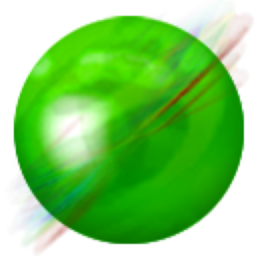
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
