How to implement image uploading and cropping in Vue technology development requires specific code examples
In modern Web development, image uploading and image cropping are one of the common requirements. . As a popular front-end framework, Vue.js provides a wealth of tools and plug-ins to help us achieve these functions. This article will introduce how to implement image uploading and cropping in Vue technology development, and provide specific code examples.
The implementation of image upload can be divided into two steps: selecting images and uploading images. In Vue, third-party plugins can be used to simplify this process. One of the commonly used plug-ins is vue-upload-component. This plugin provides a simple and easy-to-use component to handle image uploads.
First, we need to install the vue-upload-component plug-in. It can be installed through npm:
npm install vue-upload-component
Then, introduce and register this plug-in in the project:
import vueUploadComponent from 'vue-upload-component' Vue.component('file-upload', vueUploadComponent)
Now, we can use this plug-in in the Vue component. For example, let's say we have a form component that contains a form item for image upload. You can use the following code:
<template> <div> <FileUpload v-model="image" name="image" accept="image/*" @input-filter="inputFilter" > <button>选择图片</button> </FileUpload> <button @click="uploadImage" :disabled="!image">上传图片</button> </div> </template> <script> export default { data() { return { image: null } }, methods: { uploadImage() { // 执行上传图片的操作 }, inputFilter(newFile, oldFile, prevent) { if (newFile && !oldFile) { // 检查文件类型和大小等限制 if (newFile.type !== 'image/jpeg') { alert('只允许上传JPEG格式的图片') return prevent() } if (newFile.size > 1024 * 1024) { alert('图片大小不能超过1MB') return prevent() } } } } } </script>
In the above code, we use the FileUpload component to implement the image upload form item. This component binds a v-model to track the selected image. The user can click the button to select a picture, and after the picture selection is completed, the @input-filter event is triggered to verify the file type and size. In the uploadImage method, we can perform the actual operation of uploading images.
Next, let’s look at how to implement image cropping. In Vue, you can use the third-party plug-in vue-cropper to simplify this process.
First, we need to install the vue-cropper plug-in. It can be installed through npm:
npm install vue-cropper
Then, introduce and register this plug-in in the project:
import vueCropper from 'vue-cropper' Vue.component('vue-cropper', vueCropper)
Now, we can use this plug-in in the Vue component. For example, let's say we have an image cropping component. You can use the following code:
<template> <div> <vue-cropper ref="cropper" v-model="croppedImage" :width="300" :height="300" :autoCrop="true" :responsive="true" src="path/to/image.jpg" ></vue-cropper> <button @click="cropImage">裁剪图片</button> </div> </template> <script> export default { data() { return { croppedImage: null } }, methods: { cropImage() { const cropper = this.$refs.cropper if (cropper) { const result = cropper.getCroppedCanvas() this.croppedImage = result.toDataURL('image/jpeg') } } } } </script>
In the above code, we use the vue-cropper component to crop the image. This component binds a v-model to track the cropped image. The user can adjust the position and size of the cropping box, and click the button to execute the cropImage method to obtain the cropped image.
To sum up, this article introduces the method of image uploading and cropping in Vue technology development, and provides specific code examples. By using third-party plug-ins, we can simplify the development process and improve development efficiency. Hope this article helps you!
The above is the detailed content of How to implement image uploading and cropping in Vue technology development. For more information, please follow other related articles on the PHP Chinese website!
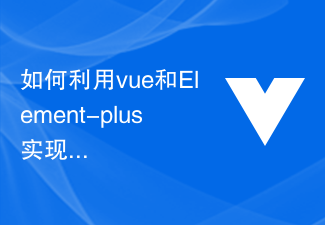
如何利用Vue和ElementPlus实现图片的裁剪和旋转功能引言:随着Web应用对于用户体验的要求越来越高,图片的处理成为了不可忽视的一部分。Vue作为一种流行的JavaScript框架,结合ElementPlus这一优秀的UI组件库,为我们提供了丰富的工具和功能,方便快捷地实现图片的裁剪和旋转。本文将以Vue和ElementPlus为基础,为大家介
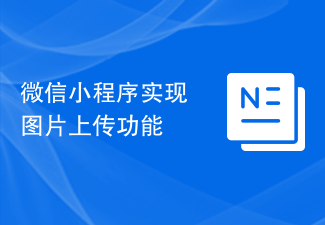
微信小程序实现图片上传功能随着移动互联网的发展,微信小程序已经成为了人们生活中不可或缺的一部分。微信小程序不仅提供了丰富的应用场景,还支持开发者自定义功能,其中包括图片上传功能。本文将介绍如何在微信小程序中实现图片上传功能,并提供具体的代码示例。一、前期准备工作在开始编写代码之前,我们需要先下载并安装微信开发者工具,并注册成为微信开发者。同时,还需要了解微信
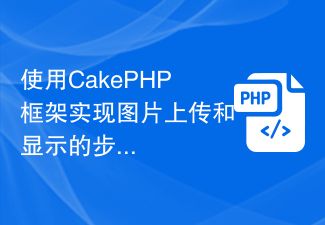
使用CakePHP框架实现图片上传和显示的步骤引言:在现代Web应用程序中,图片上传和显示是常见的功能需求。CakePHP框架为开发者提供了强大的功能和便捷的工具,使得实现图片上传和显示变得简单高效。本文将向您介绍如何使用CakePHP框架来实现图片上传和显示。步骤1:创建文件上传表单首先,我们需要在视图文件中创建一个表单,用于用户上传图片。以下是一个示例的
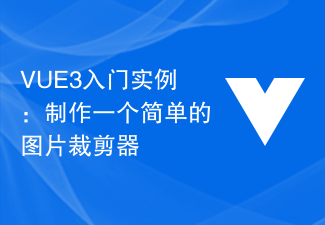
Vue.js是一款流行的JavaScript前端框架,目前已经推出了最新的版本——Vue3,新版Vue在性能、体积以及开发体验上均有所提升,受到越来越多的开发者欢迎。本文将介绍如何使用Vue3制作一个简单的图片裁剪器。首先,我们需要创建一个Vue项目并安装所需的插件。可以使用VueCLI来创建项目,也可以手动搭建。这里我们以使用VueCLI的方式为例:#
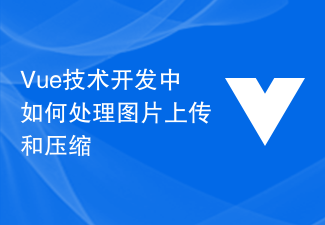
Vue技术开发中如何处理图片上传和压缩在现代web应用中,图片上传是一个非常常见的需求。然而,由于网络传输和存储等方面的原因,直接上传原始的高分辨率图片可能会导致上传速度慢和存储空间的大量浪费。因此,对于图片的上传和压缩是非常重要的。在Vue技术开发中,我们可以使用一些现成的解决方案来处理图片上传和压缩。下面将介绍如何使用vue-upload-compone
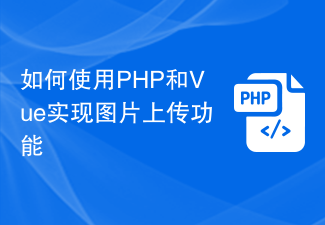
如何使用PHP和Vue实现图片上传功能在现代网页开发中,图片上传功能是非常常见的需求。本文将详细介绍如何使用PHP和Vue来实现图片上传功能,并提供具体的代码示例。一、前端部分(Vue)首先需要在前端创建一个用于上传图片的表单。具体代码如下:<template><div><inputtype="fil
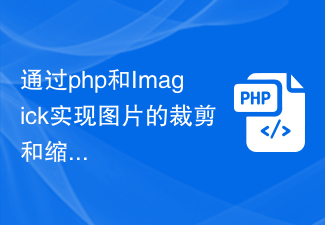
通过PHP和Imagick实现图片的裁剪和缩放摘要:在Web开发中,经常需要对图片进行裁剪和缩放以适应各种需求。本文将介绍如何使用PHP和Imagick库来实现图片的裁剪和缩放,并提供代码示例供读者参考。引言:随着互联网的快速发展,图片在网页中扮演着越来越重要的角色。然而,由于每个网页都有各自的布局和尺寸要求,往往需要对图片进行裁剪和缩放来适应不同的场景。P
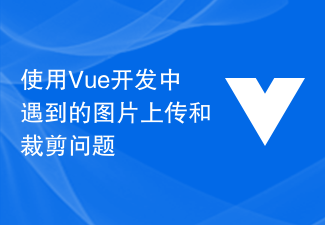
标题:Vue开发中图片上传和裁剪问题及解决方法引言:在Vue开发中,图片上传和裁剪是常见的需求。本文将介绍在Vue开发中遇到的图片上传和裁剪问题,并给出解决方法和具体的代码示例。一、图片上传问题:选择图片上传按钮无法触发文件选择框:这个问题通常是因为没有正确绑定事件或者绑定的事件不生效。可以通过在模板中绑定点击事件,并在对应的方法中触发文件选择框。代码示例:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
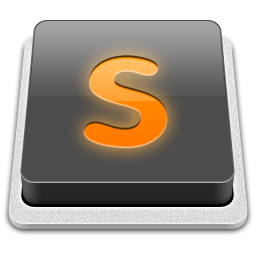
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
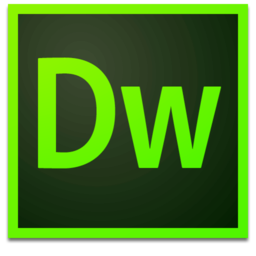
Dreamweaver Mac version
Visual web development tools