Detailed analysis of common memory management issues in C++
C is a powerful programming language, but it is also a language that requires careful handling of memory management. When writing programs in C, you often encounter memory management problems. This article will analyze common memory management problems in C in detail and provide specific code examples to help readers understand and solve these problems.
1. Memory Leak
Memory leak refers to the fact that the dynamically allocated memory in the program is not released correctly, resulting in a waste of memory resources. This is a common problem, especially in large or long-running programs. The following is an example of a memory leak:
void func() { int* ptr = new int; // ... // do some operations // ... return; // 未释放内存 }
In this example, ptr
points to a dynamically allocated int
type variable, but is not passed # at the end of the function ##deleteKeyword to release this memory. When this function is called repeatedly, it causes a memory leak.
delete keyword to release this memory when it is no longer needed:
void func() { int* ptr = new int; // ... // do some operations // ... delete ptr; // 释放内存 return; }It should be noted that it should be ensured that all possible Dynamically allocated memory is released before the end of the path to avoid memory leaks. In addition, you can consider using smart pointers (such as
std::shared_ptr,
std::unique_ptr) to avoid manual memory management, thereby reducing the risk of memory leaks.
Dangling Pointer refers to a pointer to freed or invalid memory. Accessing wild pointers can cause undefined behavior, such as program crashes or unpredictable results. The following is an example of a wild pointer:
int* createInt() { int x = 10; return &x; } void func() { int* ptr = createInt(); // ... // do some operations // ... delete ptr; // 错误:野指针 return; }In this example, the
createInt() function returns the address of a local variable
x, but when the function returns, # The life cycle of ##x
ends, its memory is released, and ptr
points to invalid memory. The solution is to ensure that the pointer points to valid memory before creating it, or to set the pointer to
when it is no longer needed: <pre class='brush:php;toolbar:false;'>void func() {
int* ptr = nullptr; // 初始化指针
// ...
// create dynamic memory
ptr = new int;
// do some operations
// ...
delete ptr; // 释放内存
ptr = nullptr; // 置空指针
return;
}</pre>
When using pointers Be extra careful to ensure that a pointer is no longer used at the end of its lifetime to avoid wild pointer problems.
3. Repeated release (Double Free)
Repeated release refers to releasing the same piece of memory multiple times. Such behavior can also lead to undefined behavior, such as program crashes or data corruption. The following is an example of repeated release:void func() { int* ptr = new int; // ... // do some operations // ... delete ptr; // ... // do more operations // ... delete ptr; // 错误:重复释放 return; }
In this example, ptr
points to a dynamically allocated int
type variable. The first delete
releases the memory pointed to by ptr
, but the second delete
tries to release the memory again, causing a repeated release problem. The solution is to set the pointer to
after each release of memory to prevent repeated releases: <pre class='brush:php;toolbar:false;'>void func() {
int* ptr = new int;
// ...
// do some operations
// ...
delete ptr;
ptr = nullptr; // 置空指针
// ...
// do more operations
// ...
if (ptr != nullptr) {
delete ptr; // 多次检查指针是否为空
ptr = nullptr;
}
return;
}</pre>
Use smart pointers to avoid the problem of repeated releases. Because smart pointers automatically manage the release of memory.
The above is a detailed analysis of common memory management problems and solutions in C. When writing C programs, be sure to pay attention to the correct allocation and release of memory to avoid problems such as memory leaks, wild pointers, and repeated releases. At the same time, it is recommended to use modern C features such as smart pointers to simplify memory management and improve the security and reliability of the code.
The above is the detailed content of Detailed analysis of common memory management issues in C++. For more information, please follow other related articles on the PHP Chinese website!
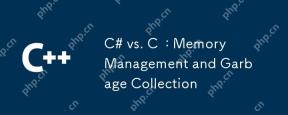
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
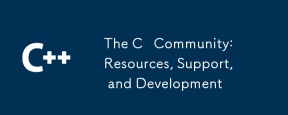
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
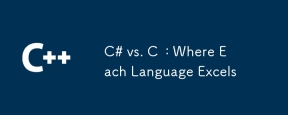
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
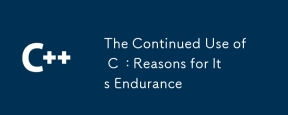
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
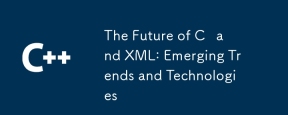
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
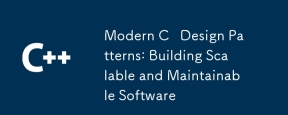
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
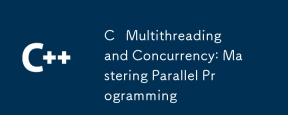
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
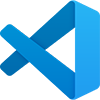
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
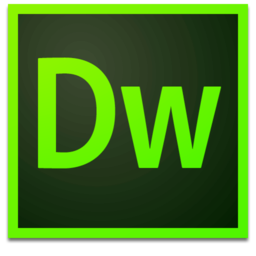
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor