


How to handle asynchronous requests and concurrent processing in PHP development
How to handle asynchronous requests and concurrent processing in PHP development requires specific code examples
In web development, we often encounter the need to handle a large number of concurrent requests and asynchronous requests Case. Taking PHP as an example, we can use some technologies and tools to handle these requirements and improve the performance and responsiveness of the system. This article explains how to handle asynchronous requests and concurrent processing, and provides some concrete code examples.
1. Processing of asynchronous requests
- Using Ajax technology
In Web development, Ajax (Asynchronous JavaScript and XML) is a method used to Front-end technology for creating asynchronous requests. Through Ajax, the browser can send an asynchronous request to the server and process it in the background without refreshing the entire page. In PHP development, you can handle asynchronous requests through the following steps:
(1) In the front-end part, use JavaScript to send asynchronous requests to the back-end PHP file, for example:
var xhr = new XMLHttpRequest(); xhr.open('GET', 'example.php', true); xhr.onreadystatechange = function() { if (xhr.readyState === 4 && xhr.status === 200) { var response = xhr.responseText; // 处理返回的数据 } }; xhr.send();
(2) In the back-end PHP file, perform corresponding processing as needed and return data, for example:
$data = array('name' => 'John', 'age' => 30); echo json_encode($data);
Through the above code, the front-end page can obtain the data returned by the back-end and process it accordingly.
- Multi-threaded processing using PHP
In PHP, asynchronous requests can be processed through multi-threads to improve the processing efficiency of the system. You can use PHP's extension library pthreads to implement multi-threading. The following is a simple multi-threaded processing code example:
class WorkerThread extends Thread { private $data; public function __construct($data) { $this->data = $data; } public function run() { // 进行相应的处理 // ... // 返回结果 return $result; } } $threads = array(); $data = array('name' => 'John', 'age' => 30); for ($i = 0; $i < 10; $i++) { $thread = new WorkerThread($data); $threads[] = $thread; $thread->start(); } foreach ($threads as $thread) { $result = $thread->join(); // 处理返回的结果 }
Through the above code, multiple threads can be created to process asynchronous requests. Each thread independently executes the specified task and returns the results for subsequent processing.
2. Implementation of concurrent processing
- Multi-process processing using PHP
In PHP, concurrent processing can be achieved through multiple processes to improve System processing capabilities. You can use PHP's extension library pcntl to implement multi-process processing. The following is a simple code example of multi-process processing:
$processes = array(); for ($i = 0; $i < 10; $i++) { $pid = pcntl_fork(); if ($pid == -1) { die('Fork failed'); } elseif ($pid) { $processes[] = $pid; } else { // 进行相应的处理 // ... exit(); } } foreach ($processes as $pid) { pcntl_waitpid($pid, $status); // 处理返回的结果 }
Through the above code, multiple processes can be created to achieve concurrent processing. Each process independently performs specified tasks and returns results for subsequent processing.
- Use PHP's concurrent HTTP request library
In addition to using multi-threads and multi-processes to achieve concurrent processing, you can also use PHP's concurrent HTTP request library to achieve it. For example, you can use libraries such as Guzzle to send concurrent HTTP requests. The following is a simple code example:
use GuzzleHttpClient; use GuzzleHttpPromise; $client = new Client(); $promises = []; for ($i = 0; $i < 10; $i++) { $promises[] = $client->getAsync('http://example.com'); } $results = Promiseunwrap($promises);
Through the above code, you can send multiple HTTP requests concurrently and obtain the returned results.
In summary, PHP development can handle asynchronous requests and concurrent processing needs through the above methods. Based on specific business scenarios and needs, appropriate methods and tools can be selected to optimize system performance and responsiveness. I hope the above content is helpful to everyone.
The above is the detailed content of How to handle asynchronous requests and concurrent processing in PHP development. For more information, please follow other related articles on the PHP Chinese website!
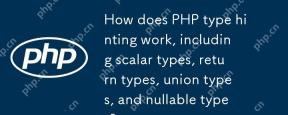
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
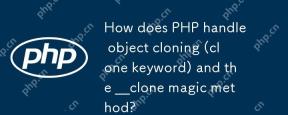
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
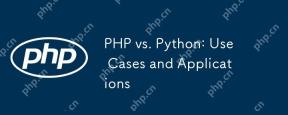
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
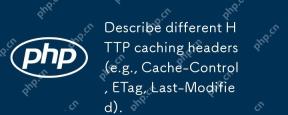
Key players in HTTP cache headers include Cache-Control, ETag, and Last-Modified. 1.Cache-Control is used to control caching policies. Example: Cache-Control:max-age=3600,public. 2. ETag verifies resource changes through unique identifiers, example: ETag: "686897696a7c876b7e". 3.Last-Modified indicates the resource's last modification time, example: Last-Modified:Wed,21Oct201507:28:00GMT.
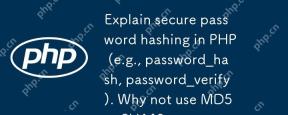
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
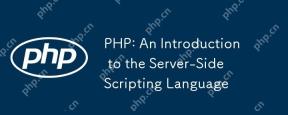
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
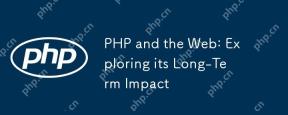
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
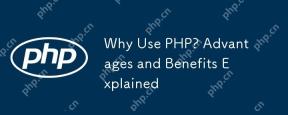
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
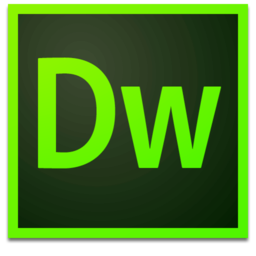
Dreamweaver Mac version
Visual web development tools
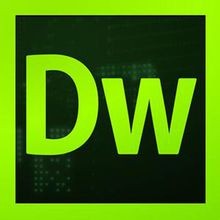
Dreamweaver CS6
Visual web development tools