


How to optimize concurrent operations and thread safety in PHP development
Concurrent operations refer to the ability to handle multiple requests or tasks at the same time. In PHP development, thread safety needs to be taken into consideration when handling concurrent operations to ensure the correctness of data sharing and state management between multiple threads. This article will introduce some techniques for optimizing concurrent operations and ensuring thread safety, with specific code examples.
1. Use lock mechanism to ensure thread safety
- Mutex Lock
Mutex lock is the most basic thread synchronization mechanism, which can ensure that only A thread can access protected resources. In PHP, you can use the Mutex extension to implement a mutex lock.
Sample code:
$mutex = new Mutex(); // 请求加锁 $mutex->lock(); // 执行需要保护的代码段 // 释放锁 $mutex->unlock();
- ReadWrite Lock (ReadWrite Lock)
ReadWrite Lock allows multiple threads to read shared resources at the same time, but only allows one thread Perform write operations. In PHP, you can use the Synchronized extension to implement read-write locks.
Sample code:
$rwlock = new ReadWriteLock(); // 只读操作请求加锁 $rwlock->rlock(); // 执行只读操作的代码段 // 释放只读操作锁 $rwlock->runlock(); // 写操作请求加锁 $rwlock->wlock(); // 执行写操作的代码段 // 释放写操作锁 $rwlock->wunlock();
2. Use connection pool to improve the concurrency of database operations
Database operations are one of the common bottlenecks in web development, especially in Performance problems are prone to occur in high concurrency scenarios. Using a connection pool can effectively improve the concurrency of database operations.
Sample code:
// 初始化连接池 $pool = new SwooleCoroutineChannel(10); // 添加数据库连接到连接池 $pool->push(new PDO('mysql:host=localhost;port=3306;dbname=test', 'username', 'password')); // 从连接池获取连接对象 $db = $pool->pop(); // 执行数据库操作 $stmt = $db->prepare('SELECT * FROM users'); $stmt->execute(); $results = $stmt->fetchAll(); // 释放连接对象 $pool->push($db);
3. Use process queue to implement concurrent task processing
Concurrent task processing refers to processing multiple tasks at the same time, and tasks can be distributed to multiple processes to improve processing efficiency.
Sample code:
// 创建进程池 $pool = new SwooleProcessPool(4); // 设置任务回调函数 $pool->on('WorkerStart', function($pool, $workerId) { // 从任务队列中获取任务 while(true) { $task = $pool->pop(); // 处理任务 // ... // 完成任务 $pool->ack(); } }); // 启动进程池 $pool->start(); // 添加任务到队列 $pool->push($task); // 等待任务完成 $pool->wait();
Summary:
In PHP development, optimizing concurrent operations and ensuring thread safety are important aspects to improve system performance and reliability. This article introduces the use of lock mechanisms to ensure thread safety, the use of connection pools to improve the concurrency of database operations, and the use of process queues to handle concurrent tasks, and gives specific code examples. By rationally using these technical means, the concurrent processing capabilities and thread safety of PHP applications can be effectively improved.
The above is the detailed content of How to optimize concurrent operations and thread safety in PHP development. For more information, please follow other related articles on the PHP Chinese website!
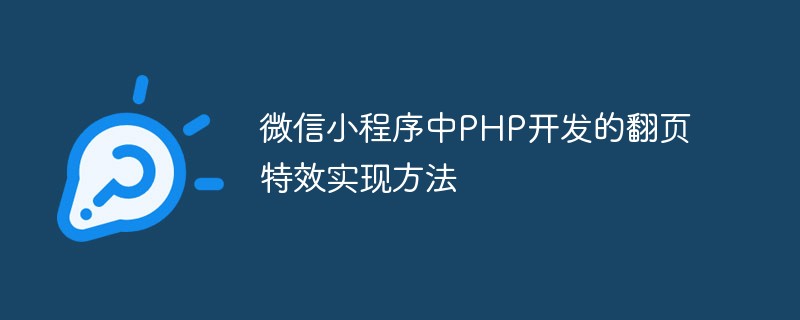
在微信小程序中,PHP开发的翻页特效是非常常见的功能。通过这种特效,用户可以轻松地在不同的页面之间进行切换,浏览更多的内容。在本文中,我们将介绍如何使用PHP来实现微信小程序中的翻页特效。我们将会讲解一些基本的PHP知识和技巧,以及一些实际的代码示例。理解基本的PHP语言知识在PHP中,我们经常会用到IF/ELSE语句、循环结构,以及函数等一些基本语言知识。
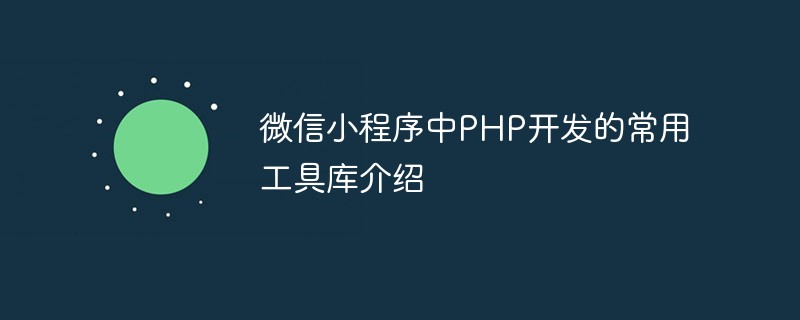
随着微信小程序的普及和发展,越来越多的开发者开始涉足其中。而PHP作为一种后端技术的代表,也在小程序中得到了广泛的运用。在小程序的开发中,PHP常用工具库也是很重要的一个部分。本文将介绍几款比较实用的PHP常用工具库,供大家参考。一、EasyWeChatEasyWeChat是一个开源的微信开发工具库,用于快速开发微信应用。它提供了一些常用的微信接口,如微信公
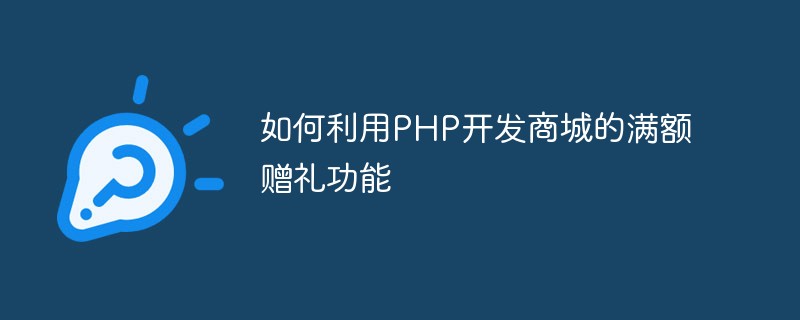
网上购物已经成为人们日常生活中不可或缺的一部分,因此,越来越多的企业开始关注电商领域。开发一款实用、易用的商城网站也成为了企业提高销售额、拓展市场的必要手段之一。在商城网站中,满额赠礼功能是提高用户购买欲望和促进销售增长的重要功能之一。本文将探讨如何利用PHP开发商城的满额赠礼功能。一、满额赠礼功能的实现思路在商城开发中,如何实现满额赠礼功能呢?简单来说就是
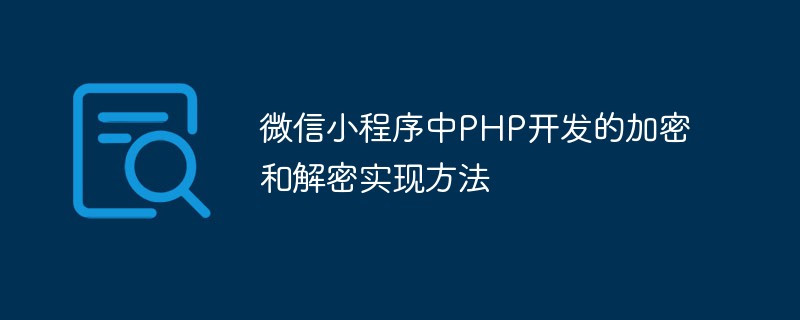
随着微信小程序在移动应用市场中越来越流行,它的开发也受到越来越多的关注。在小程序中,PHP作为一种常用的后端语言,经常用于处理敏感数据的加密和解密。本文将介绍在微信小程序中如何使用PHP实现加密和解密。一、什么是加密和解密?加密是将敏感数据转换为不可读的形式,以确保数据在传输过程中不被窃取或篡改。解密是将加密数据还原为原始数据。在小程序中,加密和解密通常包括
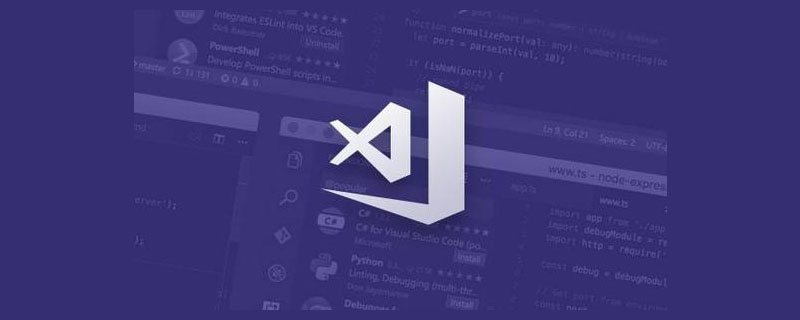
本篇文章给大家推荐一些VSCode+PHP开发中实用的插件。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。
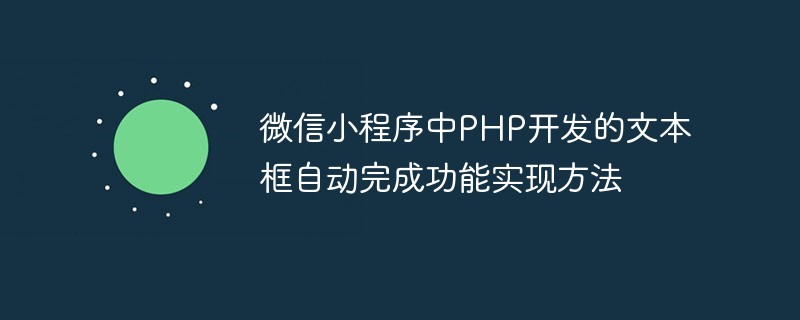
随着微信小程序的普及,各类开发需求也日渐增多。其中,文本框自动完成功能是小程序中常用的功能之一。虽然微信小程序提供了一些原生的组件,但是有一些特殊需求还是需要进行二次开发。本文将介绍如何使用PHP语言实现微信小程序中文本框自动完成功能。准备工作在开始开发之前,需要准备一些基本的环境和工具。首先,需要安装好PHP环境。其次,需要在微信小程序后台获取到自己的Ap
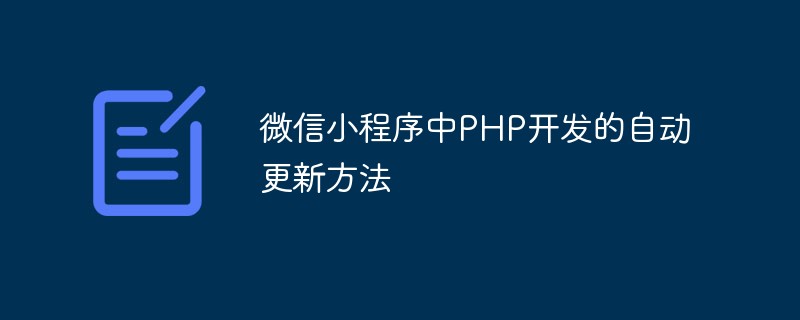
近年来,移动互联网的快速发展和移动终端的普及,让微信应用程序成为了人们生活中不可或缺的一部分。而在微信应用程序中,小程序更是以其轻量、快速、便捷的特点受到了广泛的欢迎。但是,对于小程序中的数据更新问题,却成为了一个比较头疼的问题。为了解决这一问题,我们可以使用PHP开发的自动更新方法来实现自动化数据更新。本篇文章就来探讨一下微信小程序中PHP开发的自动更新方
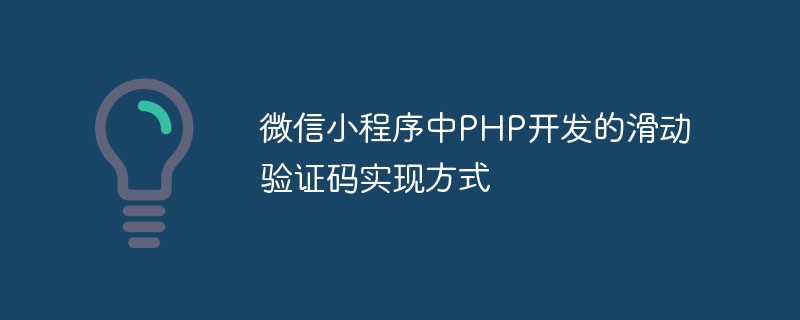
随着互联网的快速发展,网络安全问题也变得越来越严峻。针对恶意攻击、刷单等安全威胁,很多网站和应用程序都使用了验证码来保护用户信息和系统安全。在微信小程序中,如何实现一个安全可靠的滑动验证码呢?本文将介绍使用PHP开发的滑动验证码实现方式。一、滑动验证码的原理滑动验证码是指在验证用户身份时,通过用户在滑块上滑动完成验证过程。其原理是将一张图片分成两部分,一部分


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
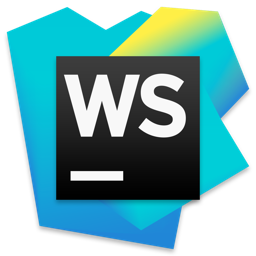
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
