How to solve thread scheduling and optimization problems in Java
How to solve thread scheduling and optimization problems in Java
Introduction:
In Java development, using multi-threads can improve the concurrency performance of the program, but at the same time It also brings some problems, such as scheduling and optimization between threads. This article will introduce how to solve thread scheduling and optimization problems in Java and provide some specific code examples.
1. Thread scheduling issues:
1.1 Setting thread priority:
In Java, you can use the setPriority() method to set the priority of a thread. The priority range is 1-10, and the default is 5. Higher priority threads are more likely to be executed first when CPU is scheduled. The sample code is as follows:
Thread thread = new Thread(); // 设置为最高优先级 thread.setPriority(Thread.MAX_PRIORITY); // 设置为较低优先级 thread.setPriority(Thread.MIN_PRIORITY);
1.2 Thread sleep:
Use the Thread.sleep() method to pause the thread for a period of time to give other threads a chance to execute. The sample code is as follows:
try { // 线程暂停1秒 Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); }
1.3 Thread waiting:
Using the wait() method of the Object class can make the thread wait until a certain condition is met before continuing execution. The sample code is as follows:
Object lock = new Object(); synchronized (lock) { lock.wait(); }
2. Thread optimization issues:
2.1 Use of thread pool:
Thread pool can effectively manage and reuse threads and reduce thread creation and destruction overhead. You can use the Executor framework provided by Java to create and manage thread pools. The sample code is as follows:
ExecutorService executor = Executors.newFixedThreadPool(10); // 创建容量为10的线程池 executor.execute(new Runnable() { @Override public void run() { // 线程执行的代码 } });
2.2 Use of synchronization locks:
In a multi-threaded environment, access to shared data needs to be synchronized to prevent race conditions between threads. You can use the synchronized keyword to use synchronization locks. The sample code is as follows:
synchronized (this) { // 访问共享资源的代码 }
2.3 Use of thread local variables:
Thread local variables are unique to each thread and will not be affected by other threads. Thread local variables can be created using the ThreadLocal class. The sample code is as follows:
ThreadLocal<Integer> threadLocal = new ThreadLocal<>(); threadLocal.set(1); // 设置线程局部变量的值 int value = threadLocal.get(); // 获取线程局部变量的值
Summary:
This article introduces some methods to solve thread scheduling and optimization problems in Java development, and provides some specific code examples. By properly setting thread priorities, using thread sleeping and waiting, using thread pools, synchronization locks and thread local variables, etc., you can better manage and optimize thread execution and improve program concurrency performance.
The above is the detailed content of How to solve thread scheduling and optimization problems in Java. For more information, please follow other related articles on the PHP Chinese website!
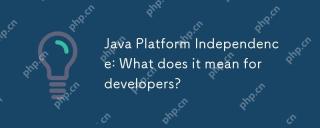
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
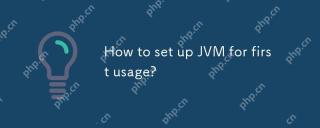
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
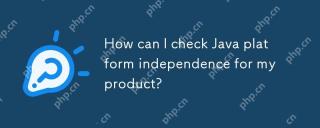
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss
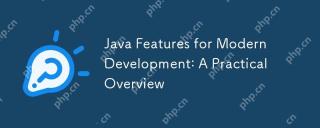
Javastandsoutinmoderndevelopmentduetoitsrobustfeatureslikelambdaexpressions,streams,andenhancedconcurrencysupport.1)Lambdaexpressionssimplifyfunctionalprogramming,makingcodemoreconciseandreadable.2)Streamsenableefficientdataprocessingwithoperationsli
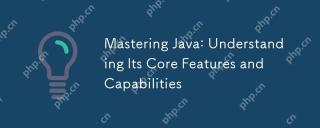
The core features of Java include platform independence, object-oriented design and a rich standard library. 1) Object-oriented design makes the code more flexible and maintainable through polymorphic features. 2) The garbage collection mechanism liberates the memory management burden of developers, but it needs to be optimized to avoid performance problems. 3) The standard library provides powerful tools from collections to networks, but data structures should be selected carefully to keep the code concise.
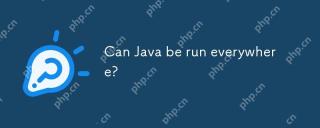
Yes,Javacanruneverywhereduetoits"WriteOnce,RunAnywhere"philosophy.1)Javacodeiscompiledintoplatform-independentbytecode.2)TheJavaVirtualMachine(JVM)interpretsorcompilesthisbytecodeintomachine-specificinstructionsatruntime,allowingthesameJava
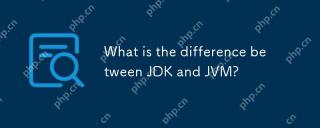
JDKincludestoolsfordevelopingandcompilingJavacode,whileJVMrunsthecompiledbytecode.1)JDKcontainsJRE,compiler,andutilities.2)JVMmanagesbytecodeexecutionandsupports"writeonce,runanywhere."3)UseJDKfordevelopmentandJREforrunningapplications.
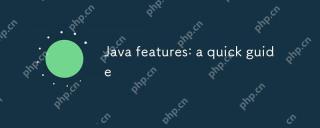
Key features of Java include: 1) object-oriented design, 2) platform independence, 3) garbage collection mechanism, 4) rich libraries and frameworks, 5) concurrency support, 6) exception handling, 7) continuous evolution. These features of Java make it a powerful tool for developing efficient and maintainable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
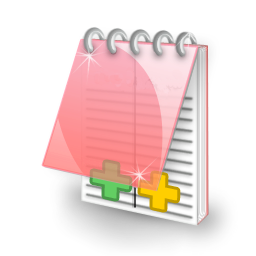
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Notepad++7.3.1
Easy-to-use and free code editor
