Introduction to multiple inheritance problems and solutions in C++
Introduction to multiple inheritance problems and solutions in C
In C, multiple inheritance is a powerful feature that allows a class to be derived from multiple parent classes Come. However, multiple inheritance also brings some problems and challenges, the most common of which is the Diamond Inheritance Problem.
Diamond inheritance means that when a class inherits from two different parent classes at the same time, and the two parent classes jointly inherit from the same base class, the derived class will have two identical base classes. Class instance. As a result, when a derived class calls a member function of the base class or accesses a member variable of the base class, ambiguity occurs, and the compiler cannot determine which member of the parent class is being called.
The following is a specific example to demonstrate the diamond inheritance problem:
#include <iostream> class Base { public: void display() { std::cout << "Base class "; } }; class LeftDerived : public Base { }; class RightDerived : public Base { }; class DiamondDerived : public LeftDerived, public RightDerived { }; int main() { DiamondDerived d; d.display(); // 编译错误,二义性调用 return 0; }
In the above example, the DiamondDerived
class derives from LeftDerived
and ## The #RightDerived classes are inherited respectively, and both classes inherit from the
Base class. When we create an object
d of
DiamondDerived in the
main function and call the
display function, the compiler cannot determine which base should be called
display function of the class, therefore a compilation error will occur.
virtual keyword in the inheritance relationship, you can ensure that the derived class only inherits one instance of the base class, not two.
#include <iostream> class Base { public: void display() { std::cout << "Base class "; } }; class LeftDerived : virtual public Base { }; class RightDerived : virtual public Base { }; class DiamondDerived : public LeftDerived, public RightDerived { }; int main() { DiamondDerived d; d.display(); // 正确调用 Base class return 0; }In the above code, we have the
LeftDerived and
RightDerived classes The
virtual keyword is used in the inheritance declaration. In this way, the
DiamondDerived class will only inherit one instance of the
Base class, making the
display function call no longer ambiguous.
The above is the detailed content of Introduction to multiple inheritance problems and solutions in C++. For more information, please follow other related articles on the PHP Chinese website!
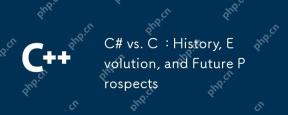
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
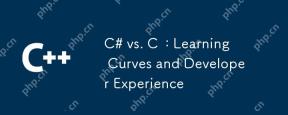
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
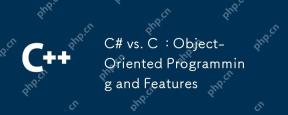
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
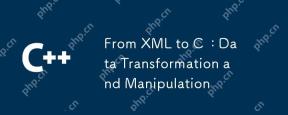
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
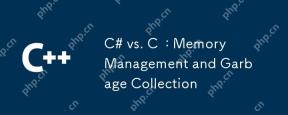
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
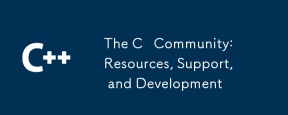
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
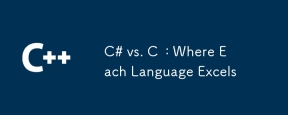
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
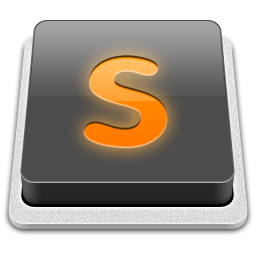
SublimeText3 Mac version
God-level code editing software (SublimeText3)
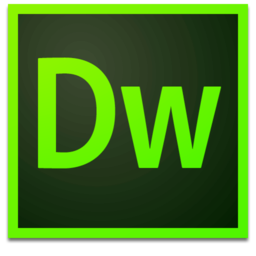
Dreamweaver Mac version
Visual web development tools
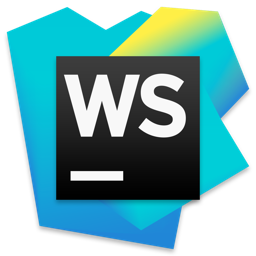
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment