


How to handle the challenges of operation, maintenance and deployment in PHP development requires specific code examples
In PHP development, operation, maintenance and deployment are important links that cannot be ignored. Good operation and maintenance practices can ensure the stability and reliability of the system, and efficient deployment processes can improve development efficiency. This article describes some common methods for handling operational and deployment challenges, and provides specific code examples.
1. Implementation of automated deployment
Automated deployment is the key to solving deployment challenges. Through automated deployment, manual errors can be reduced, deployment efficiency can be improved, and consistency of each deployment can be ensured. The following is an example of using a Shell script to implement automated deployment:
#!/bin/bash # 1. 进入项目目录 cd /path/to/project # 2. 更新代码 git pull # 3. 安装依赖 composer install # 4. 编译前端资源 npm run build # 5. 清理缓存 php artisan optimize:clear # 6. 重启服务 php artisan serve
The above code can implement a series of steps from code update to deployment completion. Adjustments will be made based on project needs.
2. Selection of configuration management tools
Configuration management tools can help us manage configuration files in different environments and switch easily. Common configuration management tools include Ansible, Chef, Puppet, etc. The following is an example of using Ansible for configuration management:
- name: Deploy PHP project hosts: web tasks: - name: Copy configuration file copy: src: "{{ env }}.ini" dest: "/etc/php.ini"
The above code will copy the corresponding configuration files to the specified directory according to the configuration files of different environments (env). In this way, we can easily switch configurations for different environments.
3. Log management and monitoring
Log management and monitoring are the keys to ensuring system stability. We need to record the operation of the system and find and solve problems in a timely manner. The following is an example of using the Monolog library to record logs:
use MonologLogger; use MonologHandlerStreamHandler; // 创建日志对象 $log = new Logger('name'); $log->pushHandler(new StreamHandler('path/to/logs.log', Logger::DEBUG)); // 记录日志 $log->info('This is an informational message'); $log->error('This is an error message');
The above code will use the Monolog library to create a log object and write the log to the specified log file. We can customize the log level and output method according to our needs.
4. Security Management
In PHP development, security management is particularly important. We need to protect users' private data and prevent hacker attacks, etc. Here is a simple example that shows how to encrypt and verify user passwords using PHP's password hash function (password_hash):
// 加密密码 $hashedPassword = password_hash($password, PASSWORD_DEFAULT); // 验证密码 if (password_verify($userInput, $hashedPassword)) { // 密码验证通过 } else { // 密码验证失败 }
The above code will use the password_hash function to encrypt the password and then use the password_verify function Verify that the password entered by the user matches the encrypted password.
5. Containerized deployment
Containerized deployment is a popular deployment method that can provide better isolation and portability. Docker is a commonly used containerization tool. The following is an example of containerized deployment using Docker-compose:
version: '3' services: app: build: context: . dockerfile: Dockerfile ports: - "80:80" volumes: - "./app:/app" networks: - frontend - backend db: image: mysql environment: MYSQL_ROOT_PASSWORD: password networks: - backend networks: frontend: backend:
The above code will define an application container and a database container through Docker-compose, and specify their configuration items. We can make adjustments based on project needs.
To sum up, dealing with the operation, maintenance and deployment challenges in PHP development requires rational selection of tools and technologies, and configuration and optimization according to project needs. Through automated deployment, configuration management, log management and monitoring, security management, and containerized deployment, the stability and reliability of the system can be improved, and development efficiency can be improved. I hope the above content is helpful to readers.
The above is the detailed content of How to deal with operation, maintenance and deployment challenges in PHP development. For more information, please follow other related articles on the PHP Chinese website!
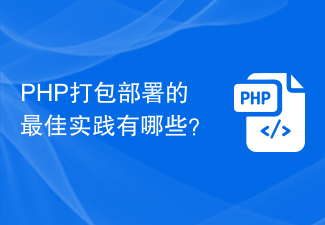
PHP打包部署的最佳实践有哪些?随着互联网技术的快速发展,PHP作为一种广泛应用于网站开发的开源编程语言,越来越多的开发者需求在项目部署上提高效率和稳定性。本文将介绍几种PHP打包部署的最佳实践,并提供相关的代码示例。使用版本控制工具版本控制工具如Git、SVN等,可以帮助开发者有效地管理代码的变更。使用版本控制工具可以轻松地跟踪和回滚代码,确保每次部署都是
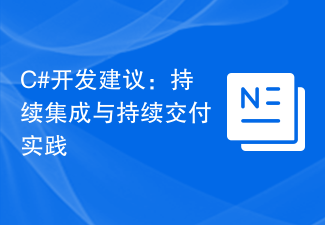
在当前的软件开发过程中,持续集成(ContinuousIntegration)和持续交付(ContinuousDelivery)已经成为了开发团队提高产品质量和加快交付速度的关键实践。无论是大型软件企业还是小型团队,都可以从这两个领域中受益。本文将为C#开发人员提供一些关于持续集成与持续交付实践的建议。自动化构建和测试自动化构建和测试是持续集成的基础。使
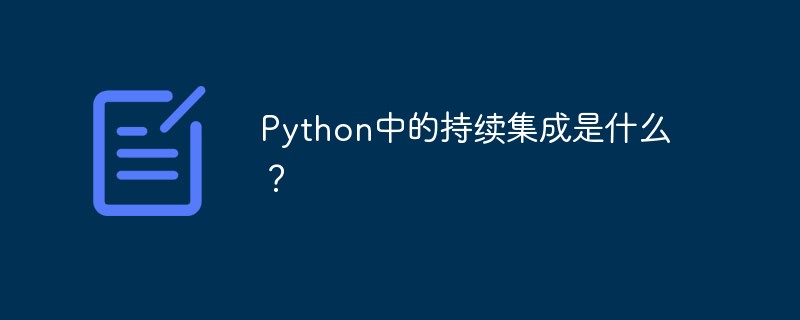
Python语言已经成为了现代软件开发中不可或缺的一部分,而其中持续集成(CI)则是高度集成及持续交付过程中的一部分,可以大大提升开发过程的效率和质量。CI的目的是通过将代码集成到一个公共的代码库,并持续运行自动化测试和静态分析工具,以最大程度地减少不必要的错误。本文将讨论Python中持续集成的原理,以及它对软件开发过程的影响。持续集成的原理CI在软件开发
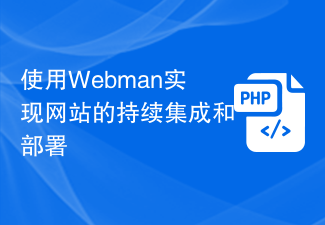
使用Webman实现网站的持续集成和部署随着互联网的迅猛发展,网站开发和维护的工作也变得越来越复杂。为了提高开发效率和保证网站的质量,采用持续集成和部署的方式成为了一个重要的选择。在这篇文章中,我将介绍如何使用Webman工具来实现网站的持续集成和部署,并附上一些代码示例。一、什么是WebmanWebman是一个基于Java的开源持续集成和部署工具,它提供了
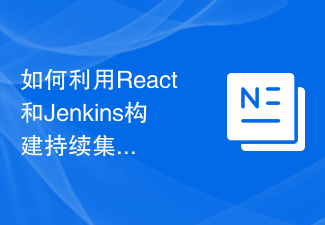
如何利用React和Jenkins构建持续集成和持续部署的前端应用引言:在当今的互联网开发中,持续集成和持续部署已经成为了开发团队提升效率、保障产品质量的重要手段。而React作为流行的前端框架,结合Jenkins这一强大的持续集成工具,能够为我们构建持续集成和持续部署的前端应用提供便捷和高效的解决方案。本文将详细介绍如何利用React和Jenkins进行持
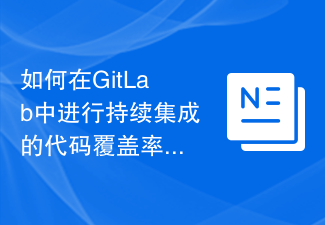
标题:GitLab持续集成中的代码覆盖率分析及实例引言:随着软件开发变得越来越复杂,代码覆盖率分析成为了评估软件测试质量的重要指标之一。而采用持续集成来进行代码覆盖率分析可以帮助开发团队实时监控自己的代码质量,提高软件开发效率。本文将介绍如何在GitLab中进行持续集成的代码覆盖率分析,并提供具体的代码示例。一、GitLab中的代码覆盖率分析1.1代码覆盖
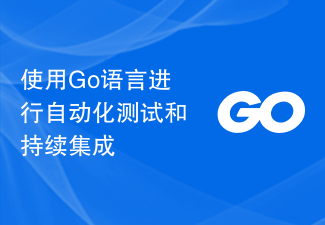
随着软件开发的不断发展,自动化测试和持续集成变得越来越重要。它们可以提高效率、减少错误,并且可以更快地推出新功能。在本文中,我们将介绍如何使用Go语言进行自动化测试和持续集成。Go语言是一种快速、高效和功能丰富的编程语言。它最初由Google所开发,旨在提供一种简单易学的语言。Go的语法简洁,并且具有并发编程的优势,这使得它成为进行自动化测试和持续集成的理想
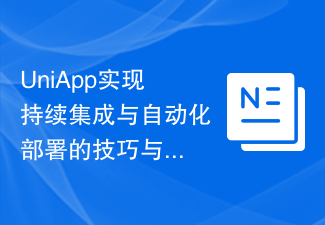
UniApp实现持续集成与自动化部署的技巧与实践随着移动应用的快速发展,我们编写和发布应用的方式也在不断演进。持续集成(ContinuousIntegration,简称CI)和自动化部署(AutomatedDeployment)成为了开发者们提高效率和降低错误风险的关键工具。本文将介绍如何在UniApp中实现持续集成与自动化部署的技巧与实践,并给出相应的


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
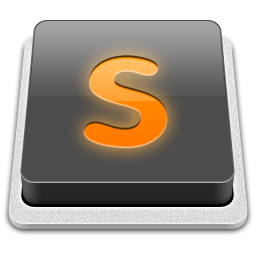
SublimeText3 Mac version
God-level code editing software (SublimeText3)
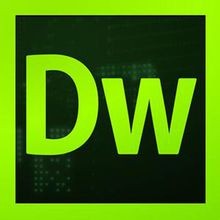
Dreamweaver CS6
Visual web development tools
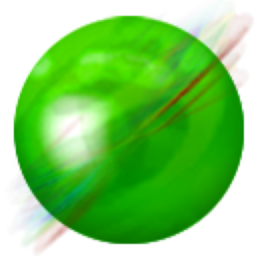
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
