


How to solve the problem of failure recovery of concurrent tasks in Go language?
How to solve the problem of failure recovery of concurrent tasks in Go language?
In modern software development, the use of concurrent processing can significantly improve the performance of the program. In the Go language, we can achieve efficient concurrent task processing by using goroutine and channel. However, concurrent tasks also bring some new challenges, such as handling failure recovery. This article will introduce some methods to solve the problem of concurrent task failure recovery in Go language and provide specific code examples.
- Error handling in concurrent tasks
When dealing with concurrent tasks, we often want to be able to detect and handle errors that may occur during task execution. In Go language, you can use Go statements and anonymous functions to create goroutines, and you can use the defer keyword to capture and handle errors that occur in goroutines.
func main() { // 创建一个带有缓冲的channel,用于接收任务的执行结果和错误信息 results := make(chan string, 10) // 启动多个goroutine,并行执行任务 for i := 0; i < 10; i++ { go func() { result, err := doTask() if err != nil { fmt.Println("Error:", err) results <- "" return } results <- result }() } // 等待所有任务执行完毕 for i := 0; i < 10; i++ { <-results } }
In the above sample code, we use a buffered channel to receive the task execution results and error information. Each goroutine will send execution results or error information to this channel. The main goroutine uses a for loop and the channel's receive operation to wait for all tasks to be completed.
- Failure recovery of concurrent tasks
In real applications, no matter how much we pay attention to the correctness of the program, failures cannot be completely avoided. When an error occurs in a goroutine, we may need to perform fault recovery, such as retry, rollback, etc. In Go language, we can use the recover function to capture and handle panic exceptions in goroutine, and then perform corresponding failure recovery operations.
func main() { // 创建一个带有缓冲的channel,用于接收任务的执行结果和错误信息 results := make(chan string, 10) // 启动多个goroutine,并行执行任务 for i := 0; i < 10; i++ { go func() { defer func() { if err := recover(); err != nil { fmt.Println("Panic:", err) // 进行故障恢复,如重试、回滚等操作 time.Sleep(time.Second) } }() result, err := doTask() if err != nil { fmt.Println("Error:", err) results <- "" return } results <- result }() } // 等待所有任务执行完毕 for i := 0; i < 10; i++ { <-results } }
In the above sample code, we added an anonymous function after the main function using the defer keyword. This anonymous function uses the recover function to capture and handle panic exceptions in goroutine, and perform corresponding failure recovery operations. In this example, we simply sleep for one second when a panic exception occurs to simulate failure recovery operations.
Summary
By using goroutine and channel, we can easily implement concurrent task processing in the Go language. In order to solve the problem of failure recovery in concurrent tasks, we can use error handling and panic/recover mechanisms to detect and handle errors and exceptions that may occur during task execution. Compared with other programming languages, the Go language provides a concise and powerful concurrent programming model, making it more convenient and efficient to solve the failure recovery problem of concurrent tasks.
The above is the detailed content of How to solve the problem of failure recovery of concurrent tasks in Go language?. For more information, please follow other related articles on the PHP Chinese website!
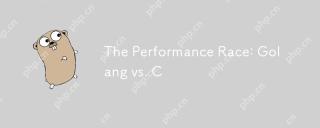
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
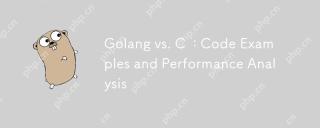
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
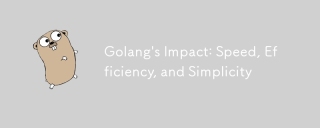
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
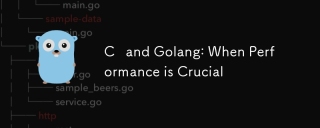
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
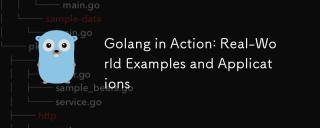
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
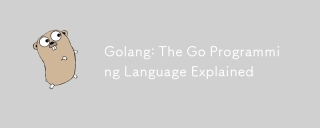
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
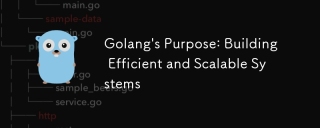
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
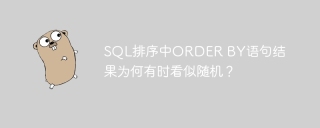
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
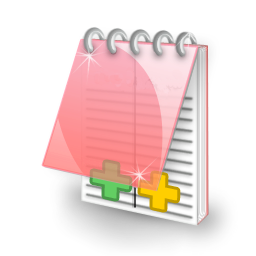
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor