How to solve thread pool and task scheduling problems in Java
How to solve the thread pool and task scheduling problems in Java
Introduction:
In Java development, using thread pools and task scheduling is a very common process way to improve application performance and concurrency. This article will introduce how to solve the problems of thread pool and task scheduling in Java, and provide specific code examples.
1. Use of thread pool
- Creating a thread pool
You can use the ThreadPoolExecutor class in Java to create a thread pool. Its construction method parameters include the number of core threads and the maximum number of threads. , Thread survival time, etc. The following is a sample code to create a thread pool:
ThreadPoolExecutor threadPool = new ThreadPoolExecutor( corePoolSize, maximumPoolSize, keepAliveTime, TimeUnit.MILLISECONDS, new LinkedBlockingQueue<Runnable>() );
- Submit a task
After creating a thread pool, you can use the submit method to submit a task to the thread pool. The sample code is as follows:
threadPool.submit(new Runnable() { public void run() { // 任务执行的逻辑代码 } });
- Close the thread pool
When the application exits, the thread pool needs to be closed and resources released. You can use the shutdown method to close the thread pool. The sample code is as follows:
threadPool.shutdown();
2. Use of task scheduling
- Delayed scheduling task
Java's ScheduledExecutorService interface provides The ability to delay scheduling tasks. You can use the schedule method to delay scheduling tasks. The sample code is as follows:
ScheduledExecutorService scheduledExecutor = Executors.newScheduledThreadPool(1); scheduledExecutor.schedule(new Runnable() { public void run() { // 在延时后执行的逻辑代码 } }, delay, TimeUnit.MILLISECONDS);
- Periodic scheduling tasks
In addition to delaying scheduling tasks, you can also use the scheduleAtFixedRate method to schedule periodically Task. The sample code is as follows:
ScheduledExecutorService scheduledExecutor = Executors.newScheduledThreadPool(1); scheduledExecutor.scheduleAtFixedRate(new Runnable() { public void run() { // 周期性执行的逻辑代码 } }, initialDelay, period, TimeUnit.MILLISECONDS);
- Close the task scheduler
Similarly, when the application exits, the task scheduler needs to be closed and resources released. You can use the shutdown method to shut down the task scheduler. The sample code is as follows:
scheduledExecutor.shutdown();
3. Integrate the thread pool and task scheduling
Sometimes we may need to use the thread pool and task scheduling at the same time To implement some complex business logic. The following is a sample code that demonstrates how to integrate thread pools and task scheduling to implement simple background data processing functions:
// 创建线程池 ThreadPoolExecutor threadPool = new ThreadPoolExecutor( corePoolSize, maximumPoolSize, keepAliveTime, TimeUnit.MILLISECONDS, new LinkedBlockingQueue<Runnable>() ); // 创建任务调度器 ScheduledExecutorService scheduledExecutor = Executors.newScheduledThreadPool(1); // 提交任务到线程池 threadPool.submit(new Runnable() { public void run() { // 后台数据处理的逻辑代码 } }); // 延时调度任务 scheduledExecutor.schedule(new Runnable() { public void run() { // 定时清理数据的逻辑代码 } }, delay, TimeUnit.MILLISECONDS); // 关闭线程池和任务调度器 threadPool.shutdown(); scheduledExecutor.shutdown();
Conclusion:
This article introduces how to solve the problems of thread pools and task scheduling in Java. And provide specific code examples. In actual development, reasonable use of thread pools and task scheduling can significantly improve application performance and efficiency. I hope readers can better understand the use of thread pools and task scheduling through this article.
The above is the detailed content of How to solve thread pool and task scheduling problems in Java. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
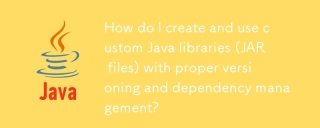
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
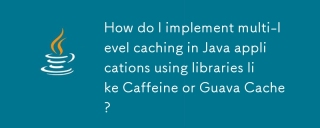
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
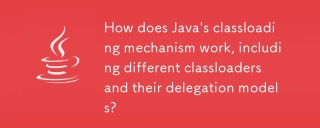
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
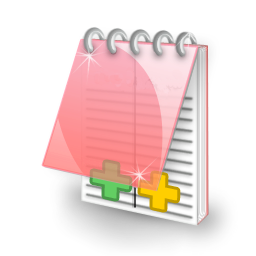
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
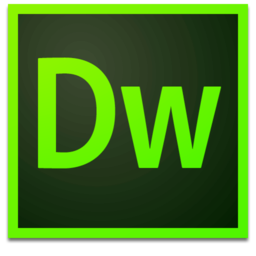
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor