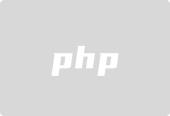
Solutions to common null pointer exception problems in C
Introduction:
In C programming, null pointer exception is a common type of error. A Null Pointer Exception occurs when a program attempts to access a pointer pointing to a null address. In large projects, null pointer exceptions may cause the program to crash or produce unpredictable behavior. Therefore, developers need to know how to avoid and handle these exceptions. This article will introduce some common null pointer exception problems and give corresponding solutions and code examples.
- Initializing pointer variables
Pointer variables should be explicitly initialized before using them. Dereferencing an uninitialized pointer causes a NullPointerException. To avoid this, it's better to initialize the pointer to nullptr.
Sample code:
int* ptr = nullptr;
- Check if the pointer is null
Before using the pointer, you need to check if it is null. You can use conditional statements such as if to determine whether the pointer is null to avoid the occurrence of null pointer exception.
Sample code:
if (ptr != nullptr) {
// 执行操作
}
- Determine whether the pointer is valid
In addition to checking whether the pointer is empty, you also need to confirm whether the pointer points to a valid memory address. Dereferencing with an invalid pointer also causes a NullPointerException. You can determine whether a pointer is valid by checking whether its value falls within the expected range.
Sample code:
if (ptr >= start && ptr < end) {
// 执行操作
}
- Using conditional operators
Conditional operators can simplify checking whether a pointer is null. You can use conditional operators to determine whether the pointer is null and perform appropriate operations.
Sample code:
ptr ? *ptr : 0;
- Exception handling
In some cases, even if the validity check of the pointer is performed, a null pointer exception may still occur. Null pointer exceptions can be caught and handled using exception handling mechanisms. You can use try-catch blocks to handle exceptions and take appropriate actions, such as restoring program state or reporting error messages.
Sample code:
try {
// 执行可能引发异常的操作
} catch(std::exception& e) {
// 处理异常
}
- Using smart pointers
Smart pointers are a way of safely managing memory resources provided by C. They help avoid null pointer exceptions and provide automatic resource release. You can use smart pointer classes provided by the C standard library such as std::shared_ptr and std::unique_ptr to manage pointers.
Sample code:
std::shared_ptr<int> ptr = std::make_shared<int>(42);
Summary:
Null pointer exception is one of the common problems in C programming. To avoid and handle null pointer exceptions, developers should always initialize pointer variables and check whether they are null before using them. In addition, you can use conditional operators, exception handling mechanisms, and smart pointers to improve the robustness and reliability of your code. With these solutions, we can reduce the occurrence of program crashes and unpredictable behavior.
The above is the detailed content of Solutions to common null pointer exception problems in C++. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn