


How to solve the problem of request rate limit and flow control of concurrent network requests in Go language?
Go language is a language that is very suitable for concurrent programming. It provides a wealth of concurrency primitives and tools, which can easily implement request rate limiting and flow control. This article will introduce how to use Go language to solve the problem of request rate limiting and flow control of concurrent network requests, and provide specific code examples.
First of all, we need to clarify the concepts of request rate limiting and flow control. Request rate limiting refers to limiting the number of requests sent within a certain period of time to avoid excessive server pressure or being banned due to too many requests. Flow control limits the amount of data sent within a certain period of time to prevent excessive data traffic from causing network congestion or bandwidth overload.
To implement request rate limiting, we can use several key components such as goroutine, channel and time packages of the Go language. First, we can create a channel to control the number of concurrent requests. Before each request, we can indicate the start of a request by sending a token to the channel. If the channel is full, it means that the current number of concurrent requests has reached the limit, and we can control the issuance of the next request by blocking and waiting. When the request is completed, we can indicate the end of a request by receiving a token from the channel. The following is a simple sample code:
package main import ( "fmt" "sync" "time" ) func request(url string, token chan struct{}, wg *sync.WaitGroup) { defer wg.Done() // 发送一个token表示开始请求 token <- struct{}{} // 模拟请求耗时 time.Sleep(1 * time.Second) // 完成请求后接收一个token <-token fmt.Println("Request completed:", url) } func main() { urls := []string{"http://example.com", "http://example.org", "http://example.net"} maxConcurrentRequests := 2 token := make(chan struct{}, maxConcurrentRequests) var wg sync.WaitGroup for _, url := range urls { wg.Add(1) go request(url, token, &wg) } wg.Wait() }
In this example, we create a channel token
and set its capacity to maxConcurrentRequests
to limit concurrency The requested quantity. At the beginning and end of each request, we send and receive a token to token
respectively. If the capacity of token
is full, the sending operation will be blocked to achieve request rate limiting.
Next, let’s introduce how to implement flow control. Flow control requires controlling the amount of data requested. We can control the frequency of sending requests by calculating the size of the data and matching the time interval and rate. Specifically, we can use the time.Ticker
and time.Sleep
of the Go language to implement the function of sending requests regularly. The following is a sample code:
package main import ( "fmt" "io/ioutil" "net/http" "time" ) func sendRequest(url string) { resp, err := http.Get(url) if err != nil { fmt.Println("Failed to send request:", err) return } defer resp.Body.Close() // 读取响应数据 data, _ := ioutil.ReadAll(resp.Body) fmt.Println("Response:", string(data)) } func main() { urls := []string{"http://example.com", "http://example.org", "http://example.net"} rate := time.Second / 2 // 控制请求速率为每秒2次 ticker := time.NewTicker(rate) for { select { case <-ticker.C: for _, url := range urls { go sendRequest(url) } } } }
In this example, we use time.Ticker
to trigger the operation of sending requests regularly. Whenever the ticker.C
channel generates a time event, we traverse the urls
slices and send requests respectively. By adjusting the value of rate
, we can control the number of requests sent per second to achieve flow control.
The above are methods and code examples to solve the problem of request speed limit and flow control of concurrent network requests in Go language. By rationally using Go language primitives and tools such as goroutine, channel, time.Ticker, etc., we can easily implement rate limiting and flow control functions for concurrent requests.
The above is the detailed content of How to solve the problem of request rate limit and flow control of concurrent network requests in Go language?. For more information, please follow other related articles on the PHP Chinese website!
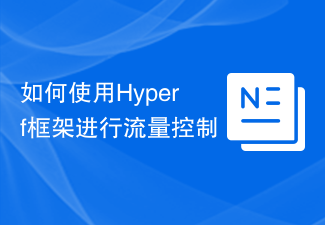
如何使用Hyperf框架进行流量控制引言:在实际开发中,对于高并发系统来说,合理的流量控制是非常重要的。流量控制可以帮助我们保护系统免受过载的风险,提高系统的稳定性和性能。在本文中,我们将介绍如何使用Hyperf框架进行流量控制,并提供具体的代码示例。一、什么是流量控制?流量控制是指对系统的访问流量进行管理和限制,以保证系统在处理大流量请求时能够正常工作。流
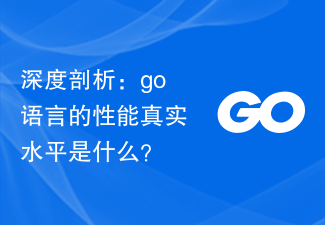
深入分析:Go语言的性能到底如何?引言:在当今的软件开发领域,性能是一个至关重要的因素。对于开发者而言,选择一个性能出色的编程语言可以提高软件应用的效率和质量。Go语言作为一种现代化的编程语言,被许多开发者认为是一种高性能的语言。本文将深入探讨Go语言的性能特点,并通过具体的代码示例进行分析。一、并发能力:Go语言作为一门基于并发的编程语言,具备出色的并发能
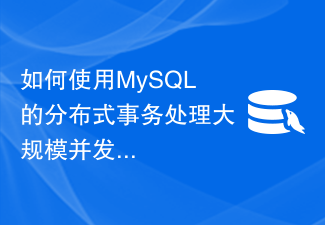
如何使用MySQL的分布式事务处理大规模并发请求引言:在当今互联网应用中,大规模并发请求是常见的挑战之一。为了保证数据的一致性和可靠性,正确处理并发请求变得至关重要。MySQL是广泛使用的关系型数据库之一,本文将介绍如何使用MySQL的分布式事务来处理大规模并发请求,并提供代码示例,帮助开发者解决这一问题。创建分布式数据库在处理大规模并发请求之前,首先需要创
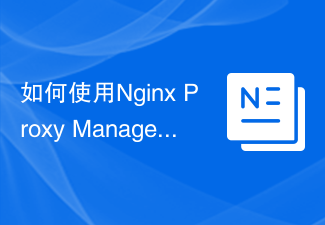
如何使用NginxProxyManager实现网络流量控制概述:网络流量控制是指通过对网络流量进行管理和控制,以达到优化网络性能,保障网络服务质量的目的。NginxProxyManager是一个基于Nginx的代理服务器管理工具,通过使用它,我们可以方便地实现网络流量控制。本文将介绍如何使用NginxProxyManager来实现网络流量控制,并
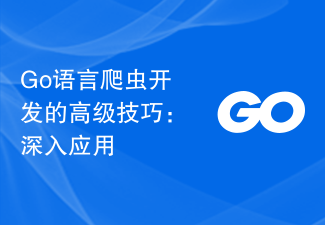
高级技巧:掌握Go语言在爬虫开发中的进阶应用引言:随着互联网的迅速发展,网页上的信息量日益庞大。而获取网页中的有用信息,就需要使用爬虫。Go语言作为一门高效、简洁的编程语言,在爬虫开发中广受欢迎。本文将介绍Go语言在爬虫开发中的一些高级技巧,并提供具体的代码示例。一、并发请求在进行爬虫开发时,我们经常需要同时请求多个页面,以提高数据的获取效率。Go语言中提供
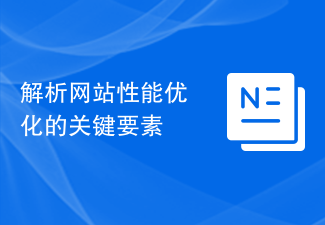
随着互联网技术的快速发展,网站已经成为了企业与用户之间沟通和交互的重要平台。然而,随着用户对网站性能要求的提高,网站性能优化越来越成为一个关键的问题。本文将分析网站性能优化的关键点,为网站管理员提供一些有用的指导。首先,优化服务器响应时间是网站性能优化的一个重要方面。服务器响应时间指的是从用户发送请求到服务器返回响应的时间间隔。一个快速响应的服务器能够提高用
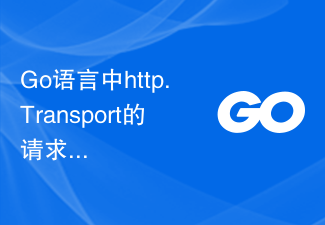
Go语言中http.Transport的请求流量控制配置与实践引言在当前的互联网环境下,高并发的请求是非常常见的情况。为了保证系统的稳定性和良好的性能,我们需要对请求进行适当的流量控制。在Go语言中,http.Transport是一个常用的HTTP客户端库,我们可以通过对其进行配置来实现请求流量控制。本文将介绍如何在Go语言中配置http.Transport
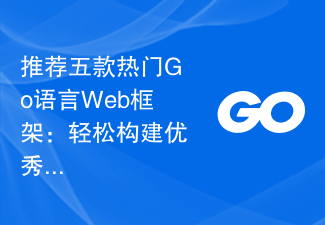
1.GinGonic:轻量级且高效GinGonic是一个轻量级且高效的Web框架,以其极快的速度和简单的API而闻名。它非常适合构建高性能的Web应用程序,尤其是那些需要处理大量并发请求的应用程序。优点:极快的速度:GinGonic是目前最快的Go语言Web框架之一,可以处理数百万个请求每秒。简单易用的API:GinGonic的API非常简单易用,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment
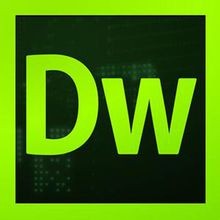
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
