How to solve the multi-thread synchronization problem in Java requires specific code examples
Introduction: With the continuous development of computer technology, multi-thread programming has become a modern software development basic requirements. However, synchronization issues in multi-threaded programming often lead to program errors and instability. For Java, a commonly used programming language, this article will explore the causes and solutions to multi-thread synchronization problems, and elaborate on them through code examples.
1. Causes of multi-thread synchronization problems
In multi-thread programming, synchronization problems mainly come from access and modification of shared data. Conflicts occur when multiple threads access or modify the same shared data at the same time. Such conflicts can lead to data consistency errors, deadlocks, and performance degradation.
2. Solutions to multi-thread synchronization problems in Java
In Java, there are many methods to solve multi-thread synchronization problems. Commonly used methods include using the synchronized keyword, Lock interface, Atomic class and Use thread-safe collection classes, etc.
- Use the synchronized keyword
The synchronized keyword is the most basic synchronization mechanism provided by the Java language and is used to modify methods and code blocks. When multiple threads access a synchronized method or code block at the same time, only one thread can execute, and other threads need to wait. By using the synchronized keyword, you can ensure safe access to shared data.
Sample code:
public class SynchronizedExample { private int count = 0; public synchronized void increment() { count++; } public int getCount() { return count; } } public class Main { public static void main(String[] args) { SynchronizedExample example = new SynchronizedExample(); // 创建多个线程对共享数据进行操作 Thread thread1 = new Thread(() -> { for (int i = 0; i < 1000; i++) { example.increment(); } }); Thread thread2 = new Thread(() -> { for (int i = 0; i < 1000; i++) { example.increment(); } }); // 启动线程 thread1.start(); thread2.start(); // 等待线程执行完毕 try { thread1.join(); thread2.join(); } catch (InterruptedException e) { e.printStackTrace(); } // 输出结果 System.out.println(example.getCount()); // 应为2000 } }
- Using the Lock interface
The Lock interface is a synchronization mechanism provided by Java to replace the synchronized keyword. Compared with the synchronized keyword, the Lock interface provides a more flexible synchronization method and supports finer-grained control. For example, specific synchronization requirements such as reentrant locks and read-write locks can be implemented.
Sample code:
public class LockExample { private int count = 0; private Lock lock = new ReentrantLock(); public void increment() { lock.lock(); try { count++; } finally { lock.unlock(); } } public int getCount() { return count; } } public class Main { public static void main(String[] args) { LockExample example = new LockExample(); Thread thread1 = new Thread(() -> { for (int i = 0; i < 1000; i++) { example.increment(); } }); Thread thread2 = new Thread(() -> { for (int i = 0; i < 1000; i++) { example.increment(); } }); thread1.start(); thread2.start(); try { thread1.join(); thread2.join(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println(example.getCount()); // 应为2000 } }
- Using the Atomic class
The Atomic class is an atomic operation class provided by Java, which can guarantee atomic operations on shared data. The Atomic class provides a series of atomic operation methods, including get, set, compareAndSet, etc., which can avoid race conditions that occur when multiple threads concurrently access shared data.
Sample code:
public class AtomicExample { private AtomicInteger count = new AtomicInteger(0); public void increment() { count.incrementAndGet(); } public int getCount() { return count.get(); } } public class Main { public static void main(String[] args) { AtomicExample example = new AtomicExample(); Thread thread1 = new Thread(() -> { for (int i = 0; i < 1000; i++) { example.increment(); } }); Thread thread2 = new Thread(() -> { for (int i = 0; i < 1000; i++) { example.increment(); } }); thread1.start(); thread2.start(); try { thread1.join(); thread2.join(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println(example.getCount()); // 应为2000 } }
3. Summary
Multi-thread synchronization problem is one of the common difficulties in multi-thread programming. For Java, a commonly used programming language, you can Use the synchronized keyword, Lock interface, Atomic class and thread-safe collection class to solve multi-thread synchronization problems. In actual development, appropriate synchronization methods should be selected according to specific needs to ensure multi-thread security and performance.
The above is the detailed content of How to solve multi-thread synchronization issues in Java. For more information, please follow other related articles on the PHP Chinese website!
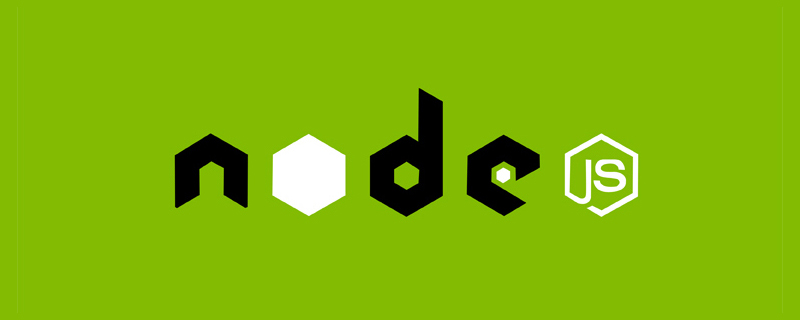
大家都知道 Node.js 是单线程的,却不知它也提供了多进(线)程模块来加速处理一些特殊任务,本文便带领大家了解下 Node.js 的多进(线)程,希望对大家有所帮助!
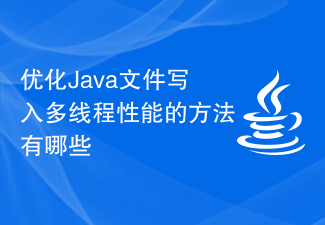
Java开发中如何优化文件写入多线程并发性能在大规模数据处理的场景中,文件的读写操作是不可避免的,而且在多线程并发的情况下,如何优化文件的写入性能变得尤为重要。本文将介绍一些在Java开发中优化文件写入多线程并发性能的方法。合理使用缓冲区在文件写入过程中,使用缓冲区可以大大提高写入性能。Java提供了多种缓冲区实现,如ByteBuffer、CharBuffe
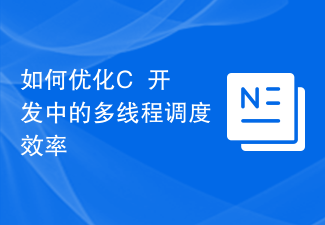
在当今的软件开发领域中,多线程编程已经成为了一种常见的开发模式。而在C++开发中,多线程调度的效率优化是开发者需要关注和解决的一个重要问题。本文将围绕如何优化C++开发中的多线程调度效率展开讨论。多线程编程的目的是为了充分利用计算机的多核处理能力,提高程序运行效率和响应速度。然而,在并行执行的同时,多线程之间的竞争条件和互斥操作可能导致线程调度的效率下降。为
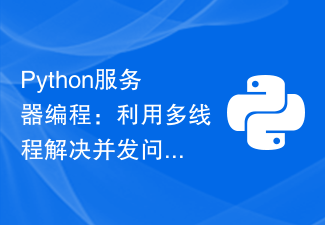
随着互联网的发展,越来越多的应用程序被开发出来,它们需要处理并发请求。例如,Web服务器需要处理多个客户端请求。在处理并发请求时,服务器需要同时处理多个请求。这时候,Python中的多线程技术就可以派上用场了。本文将介绍如何使用Python多线程技术解决并发问题。首先,我们将了解什么是多线程。然后,我们将讨论使用多线程的优点和缺点。最后,我们将演示一个实例,
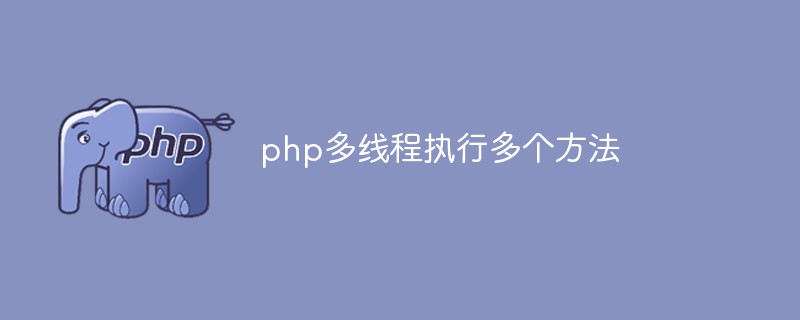
在PHP开发中,经常会遇到需要同时执行多个操作的情况。想要在一个进程中同时执行多个耗时操作,就需要使用PHP的多线程技术来实现。本文将介绍如何使用PHP多线程执行多个方法,提高程序的并发性能。
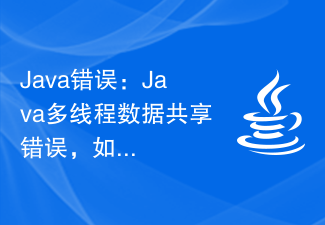
随着社会的发展和科技的进步,计算机程序已经渐渐成为我们生活中不可或缺的一部分。而Java作为一种流行的编程语言,以其可移植性、高效性和面向对象特性等而备受推崇。然而,Java程序开发过程中可能会出现一些错误,如Java多线程数据共享错误,这对于程序员们来说并不陌生。在Java程序中,多线程是非常常见的,开发者通常会使用多线程来优化程序的性能。多线程能够同时处
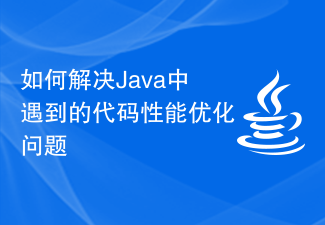
如何解决Java中遇到的代码性能优化问题随着现代软件应用的复杂性和数据量的增加,对于代码性能的需求也变得越来越高。在Java开发中,我们经常会遇到一些性能瓶颈,如何解决这些问题成为了开发者们关注的焦点。本文将介绍一些常见的Java代码性能优化问题,并提供一些解决方案。一、避免过多的对象创建和销毁在Java中,对象的创建和销毁是需要耗费资源的。因此,当一个方法
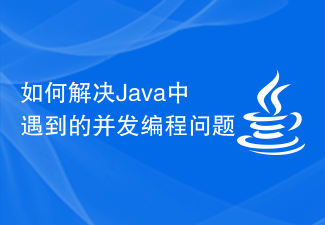
如何解决Java中遇到的并发编程问题随着计算机技术的发展和应用场景的扩大,多线程编程在软件开发中变得越来越重要。而Java作为一种常用的编程语言,也提供了强大的支持来进行并发编程。然而,并发编程也带来了一些挑战,如数据竞争、死锁、活锁等问题。本文将探讨在Java中如何解决这些并发编程的问题。数据竞争数据竞争是指当多个线程同时访问和修改共享数据时,由于执行顺序


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
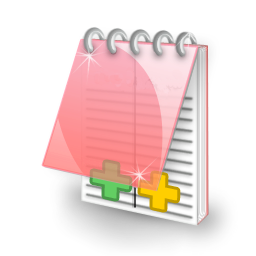
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool