How to implement a distributed file system in Java
The distributed file system is a network-based file system that allows users to access and manage distributed files over the network. Files in different physical locations. In a distributed system, file storage and management are dispersed across multiple servers, improving the reliability and performance of the file system.
Java is a programming language widely used in distributed system development. It provides a rich library and framework to implement distributed file systems. This article will introduce how to use Java to implement a simple distributed file system and provide specific code examples.
1. Overview
To implement a distributed file system, the following aspects mainly need to be considered:
- Storage distribution of files: files should be divided into different storage nodes to achieve load balancing and high availability. A consistent hashing algorithm can be used to determine the node where the file is stored.
- File index management: It is necessary to maintain a file index and record the location information of the file so that the file can be accessed and managed when needed. A distributed hash table or database can be used to store file indexes.
- File read and write operations: Basic read and write operations such as file upload, download, and deletion need to be implemented. You can use Java's network programming API to implement file transfer.
2. Implementation steps
The following is a simple implementation example of a distributed file system:
- Define the file node class
public class FileNode { private String nodeId; // 节点ID private String ipAddress; // 节点IP地址 private int port; // 节点端口号 // 构造方法和getter/setter方法省略 }
- Define file index class
public class FileIndex { private String fileName; // 文件名 private List<FileNode> nodes; // 存储文件的节点列表 // 构造方法和getter/setter方法省略 }
- Define distributed file system class
public class DistributedFileSystem { private Map<String, FileIndex> fileIndexMap; // 文件索引映射表 public DistributedFileSystem() { fileIndexMap = new HashMap<>(); } // 上传文件 public void uploadFile(String fileName, byte[] data) { // 根据文件名计算哈希值,决定文件存储的节点 String nodeId = calculateNodeId(fileName); // 假设有一个名为node的FileNode对象表示文件存储的节点 FileNode node = getNodeById(nodeId); // 将文件数据传输到节点上 uploadFileToNode(fileName, data, node); // 更新文件索引 updateFileIndex(fileName, node); } // 下载文件 public byte[] downloadFile(String fileName) { // 获取文件索引 FileIndex fileIndex = fileIndexMap.get(fileName); if (fileIndex == null) { throw new FileNotFoundException("File not found"); } // 获取存储文件的节点 FileNode node = fileIndex.getNodes().get(0); // 假设文件只存储在一个节点上 // 从节点上下载文件数据 return downloadFileFromNode(fileName, node); } // 删除文件 public void deleteFile(String fileName) { // 获取文件索引 FileIndex fileIndex = fileIndexMap.get(fileName); if (fileIndex == null) { throw new FileNotFoundException("File not found"); } // 获取存储文件的节点 FileNode node = fileIndex.getNodes().get(0); // 假设文件只存储在一个节点上 // 从节点上删除文件数据 deleteFileFromNode(fileName, node); // 更新文件索引 updateFileIndex(fileName, null); } // 计算文件存储的节点ID private String calculateNodeId(String fileName) { // 使用一致性哈希算法计算节点ID // ... } // 根据节点ID获取节点对象 private FileNode getNodeById(String nodeId) { // ... } // 将文件数据传输到节点上 private void uploadFileToNode(String fileName, byte[] data, FileNode node) { // 使用Java的网络编程API实现文件传输 // ... } // 从节点上下载文件数据 private byte[] downloadFileFromNode(String fileName, FileNode node) { // 使用Java的网络编程API实现文件传输 // ... } // 从节点上删除文件数据 private void deleteFileFromNode(String fileName, FileNode node) { // ... } // 更新文件索引 private void updateFileIndex(String fileName, FileNode node) { // ... } }
3. Summary
This article describes how to implement a simple distributed file system using Java and provides specific code examples. Actual distributed file systems also need to consider more details and functions, such as fault tolerance mechanisms, concurrency control, etc. I hope this article can help readers understand the basic principles and implementation of distributed file systems.
The above is the detailed content of How to implement a distributed file system in Java. For more information, please follow other related articles on the PHP Chinese website!
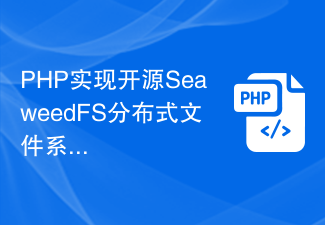
在分布式系统的架构中,文件管理和存储是非常重要的一部分。然而,传统的文件系统在应对大规模的文件存储和管理时遇到了一些问题。为了解决这些问题,SeaweedFS分布式文件系统被开发出来。在本文中,我们将介绍如何使用PHP来实现开源SeaweedFS分布式文件系统。什么是SeaweedFS?SeaweedFS是一个开源的分布式文件系统,它用于解决大规模文件存储和
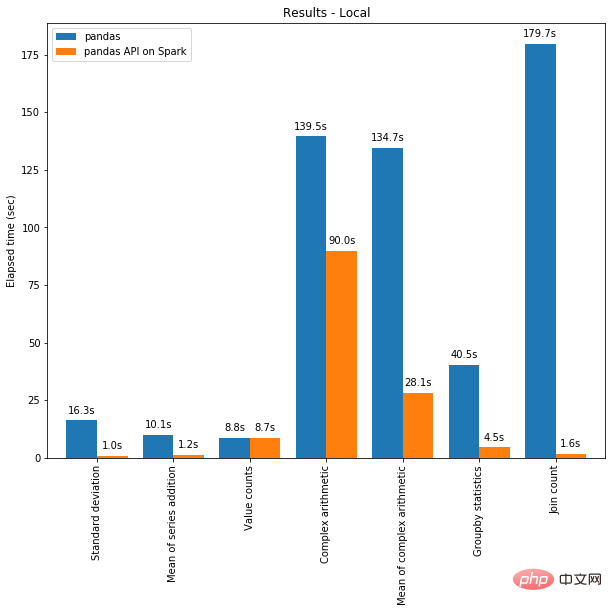
使用Python做数据处理的数据科学家或数据从业者,对数据科学包pandas并不陌生,也不乏像云朵君一样的pandas重度使用者,项目开始写的第一行代码,大多是importpandasaspd。pandas做数据处理可以说是yyds!而他的缺点也是非常明显,pandas只能单机处理,它不能随数据量线性伸缩。例如,如果pandas试图读取的数据集大于一台机器的可用内存,则会因内存不足而失败。另外pandas在处理大型数据方面非常慢,虽然有像Dask或Vaex等其他库来优化提升数
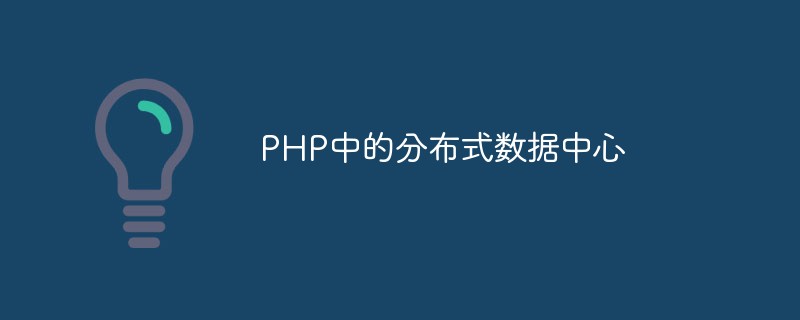
随着互联网的快速发展,网站的访问量也在不断增长。为了满足这一需求,我们需要构建高可用性的系统。分布式数据中心就是这样一个系统,它将各个数据中心的负载分散到不同的服务器上,增加系统的稳定性和可扩展性。在PHP开发中,我们也可以通过一些技术实现分布式数据中心。分布式缓存分布式缓存是互联网分布式应用中最常用的技术之一。它将数据缓存在多个节点上,提高数据的访问速度和
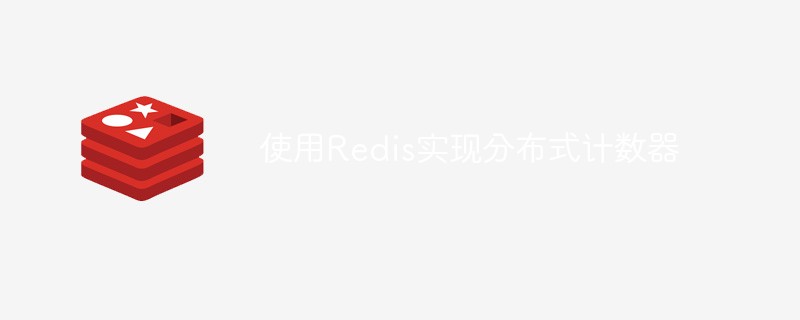
什么是分布式计数器?在分布式系统中,多个节点之间需要对共同的状态进行更新和读取,而计数器是其中一种应用最广泛的状态之一。通俗地讲,计数器就是一个变量,每次被访问时其值就会加1或减1,用于跟踪某个系统进展的指标。而分布式计数器则指的是在分布式环境下对计数器进行操作和管理。为什么要使用Redis实现分布式计数器?随着分布式计算的普及,分布式系统中的许多细节问题也
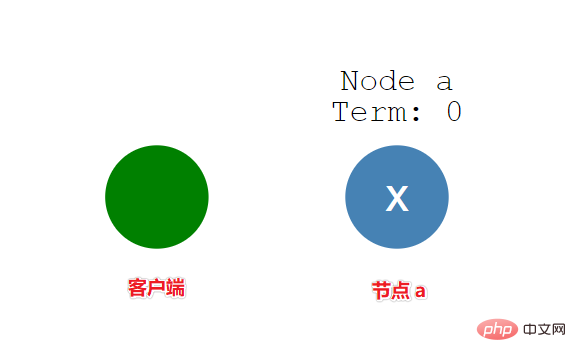
一、Raft 概述Raft 算法是分布式系统开发首选的共识算法。比如现在流行 Etcd、Consul。如果掌握了这个算法,就可以较容易地处理绝大部分场景的容错和一致性需求。比如分布式配置系统、分布式 NoSQL 存储等等,轻松突破系统的单机限制。Raft 算法是通过一切以领导者为准的方式,实现一系列值的共识和各节点日志的一致。二、Raft 角色2.1 角色跟随者(Follower):普通群众,默默接收和来自领导者的消息,当领导者心跳信息超时的
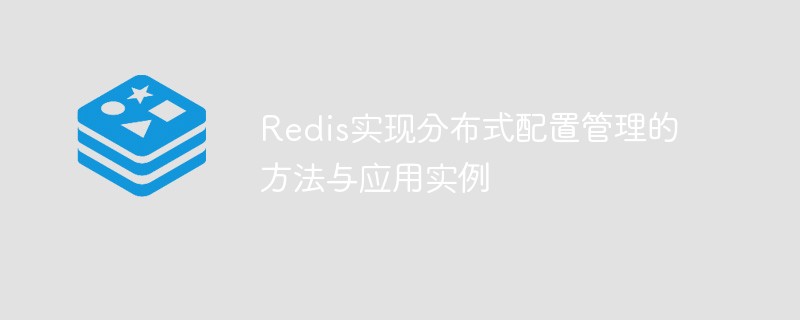
Redis实现分布式配置管理的方法与应用实例随着业务的发展,配置管理对于一个系统而言变得越来越重要。一些通用的应用配置(如数据库连接信息,缓存配置等),以及一些需要动态控制的开关配置,都需要进行统一管理和更新。在传统架构中,通常是通过在每台服务器上通过单独的配置文件进行管理,但这种方式会导致配置文件的管理和同步变得十分复杂。因此,在分布式架构下,采用一个可靠
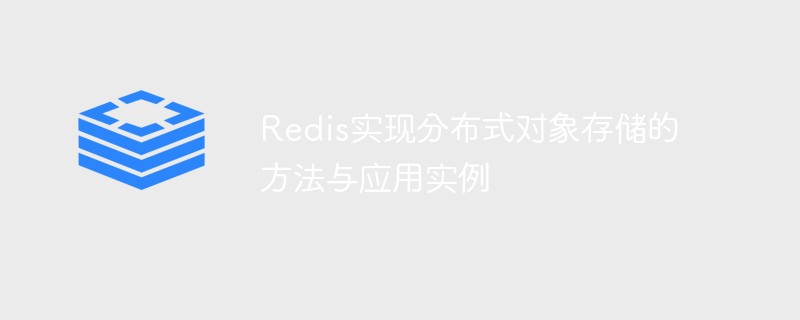
Redis实现分布式对象存储的方法与应用实例随着互联网的快速发展和数据量的快速增长,传统的单机存储已经无法满足业务的需求,因此分布式存储成为了当前业界的热门话题。Redis是一个高性能的键值对数据库,它不仅支持丰富的数据结构,而且支持分布式存储,因此具有极高的应用价值。本文将介绍Redis实现分布式对象存储的方法,并结合应用实例进行说明。一、Redis实现分
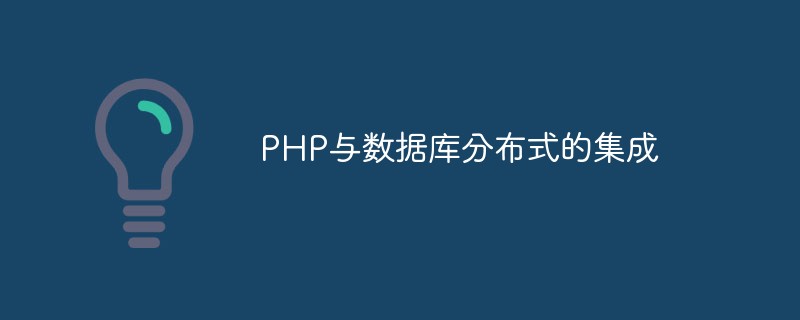
随着互联网技术的发展,对于一个网络应用而言,对数据库的操作非常频繁。特别是对于动态网站,甚至有可能出现每秒数百次的数据库请求,当数据库处理能力不能满足需求时,我们可以考虑使用数据库分布式。而分布式数据库的实现离不开与编程语言的集成。PHP作为一门非常流行的编程语言,具有较好的适用性和灵活性,这篇文章将着重介绍PHP与数据库分布式集成的实践。分布式的概念分布式


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
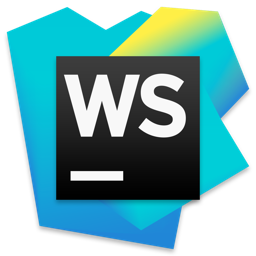
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
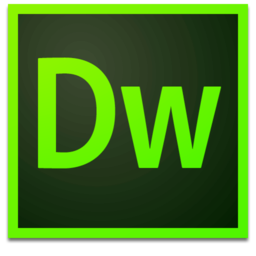
Dreamweaver Mac version
Visual web development tools