


Common problems and solution strategies for network security in Python
Common problems and solution strategies for network security in Python
Network security is one of the important issues that cannot be ignored in today's information age. With the popularity and widespread application of the Python language, network security has also become a challenge that Python developers need to face and solve. This article will introduce common network security issues in Python and provide corresponding solution strategies and code examples.
1. Network security issues
- SQL injection attack
SQL injection attack means that the attacker inserts malicious SQL code into the parameters entered by the user, thereby destroying the database Integrity and Confidentiality. In order to prevent SQL injection attacks, Python developers need to use parameter binding or use an ORM framework to build SQL query statements.
Sample code:
import MySQLdb def login(username, password): conn = MySQLdb.connect(host='localhost', user='root', passwd='password', db='mydb') cursor = conn.cursor() # 使用?占位符替代用户输入的参数 cursor.execute("SELECT * FROM users WHERE username = ? AND password = ?", (username, password)) result = cursor.fetchone() cursor.close() conn.close() if result: return True else: return False
- XSS attack
Sensitive information. In order to prevent XSS attacks, Python developers need to filter and escape user-entered data.
Sample code:
from flask import Flask, request, escape app = Flask(__name__) @app.route('/search') def search(): keyword = request.args.get('keyword') # 使用escape函数对用户输入进行转义 keyword = escape(keyword) # 对转义后的关键词进行进一步处理 # ... return "Search results" if __name__ == '__main__': app.run()
- CSRF attack
CSRF (Cross-Site Request Forgery) attack means that the attacker forces the user to Perform certain actions without knowing it. In order to prevent CSRF attacks, Python developers can verify the legitimacy of requests by generating and verifying Tokens.
Sample code:
from flask import Flask, request, session import hashlib import random app = Flask(__name__) @app.route('/transfer', methods=['POST']) def transfer(): csrf_token = request.form.get('csrf_token') # 验证Token的合法性 if csrf_token == session.get('csrf_token'): # 转账操作 amount = request.form.get('amount') # ... return 'Transfer successful' else: return 'Invalid request' @app.route('/transfer_form') def transfer_form(): # 生成和存储Token csrf_token = hashlib.sha256(str(random.getrandbits(256)).encode()).hexdigest() session['csrf_token'] = csrf_token return f""" <form action="/transfer" method="POST"> <input type="hidden" name="csrf_token" value="{csrf_token}"> <input type="text" name="amount"> <input type="submit" value="Transfer"> </form> """ if __name__ == '__main__': app.secret_key = 'secret' app.run()
2. Network security solution strategy
- Input verification
For all user-entered data, whether from the web page Forms, URL parameters, or those obtained from API requests all need to be verified. The verification process should include checks of data type, length, format, etc. to ensure the legality of the input. - Output Escape
Before outputting the data input by the user to the web page for display, it must be escaped to prevent XSS attacks. Escapes include HTML entity escaping, JavaScript escaping, etc. - Strong Password Policy
User passwords should be required to have a certain level of complexity, and users should be required to change their passwords regularly. At the same time, passwords should be stored using encryption algorithms to prevent risks caused by password leaks. - Firewall and Network Monitoring
At the network architecture level, it is recommended to configure a firewall to restrict unauthorized access to the server and use network monitoring tools to detect and block potentially malicious network activities.
To sum up, Python developers need to establish a correct network security awareness and adopt corresponding solution strategies when developing network security. These policies include input validation, output escaping, strong password policies, firewalls, and network monitoring, among others. It is true that these measures cannot completely eliminate security risks, but they can greatly improve the security of the system and its ability to resist external attacks.
The above is the detailed content of Common problems and solution strategies for network security in Python. For more information, please follow other related articles on the PHP Chinese website!
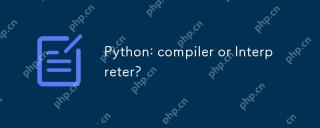
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
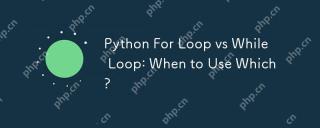
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
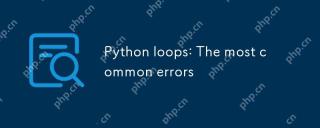
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i
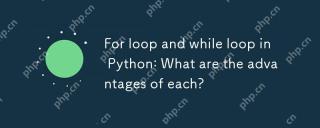
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
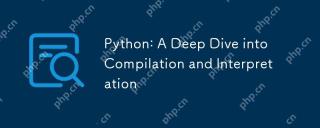
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
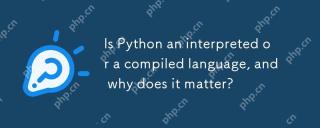
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
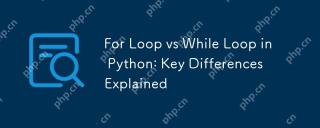
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
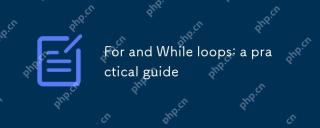
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
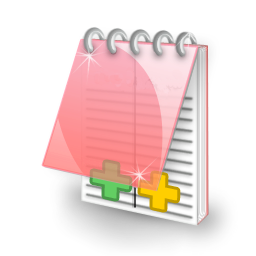
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
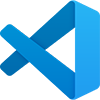
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
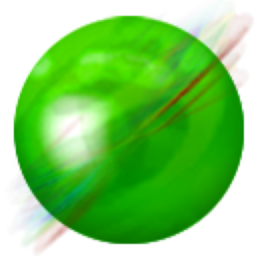
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
