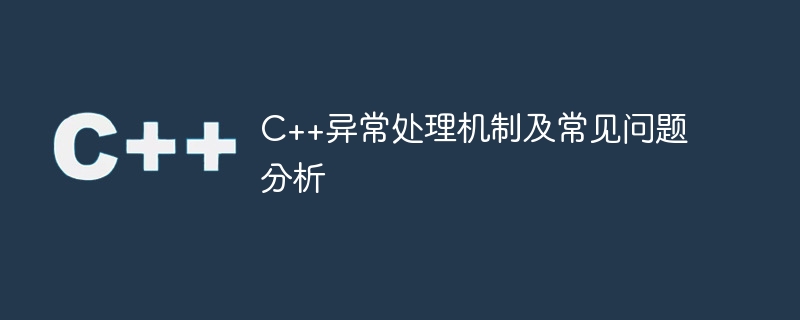
C exception handling mechanism and analysis of common problems
Introduction:
C is a powerful programming language that provides an exception handling mechanism to handle program execution Errors and anomalies in the process. Exception handling is a control flow mechanism that is used to transfer control from the current execution point to another processing point under specific conditions. This article will introduce the exception handling mechanism in C and analyze some common problems.
1. Exception handling mechanism
- Basic concept of exception: Exception is an error or abnormal situation during program running, such as division-by-zero error, insufficient memory, etc. When an exception occurs, the program interrupts the current execution flow and jumps to the exception handling code block.
- Exception handling process: C's exception handling mechanism consists of three keywords: try, catch and throw. try is used to identify code blocks, catch is used to catch and handle exceptions, and throw is used to throw exceptions.
- Exception type: The exception type in C can be a built-in type (such as int, char, etc.) or a custom type. If the exception type is not caught by the catch block, the program will terminate and call the terminate function.
- Exception catching: The catch block is used to catch and handle exceptions. Multiple catch blocks can be used to catch different types of exceptions so that they can be handled accordingly. The order of catch blocks is important. Exceptions of derived types should be caught first, and then exceptions of base class types should be caught.
- Exception throwing: The throw keyword is used to throw exceptions. The exception thrown can be of any type, including basic types and custom types. When an exception is thrown, the program will interrupt the current execution process and transfer to the catch block for processing.
2. Analysis of common problems
- Not properly catching exceptions: There may be uncaught exceptions in the code, which will cause the program to terminate. To avoid this, it is recommended to use appropriate try-catch blocks in your code to catch exceptions and handle them accordingly.
Sample code:
try {
// 可能抛出异常的代码
} catch (const std::exception& e) {
// 处理异常的代码
}
- Exceptions not handled correctly: Sometimes even if an exception is caught, it is not handled correctly. Reasonable exception handling should include printing error information, repairing error status or rolling back operations, etc.
Sample code:
try {
// 调用可能抛出异常的函数
function();
} catch (const std::exception& e) {
std::cout << "发生了异常: " << e.what() << std::endl;
// 其他异常处理代码
// 修复错误状态或回滚操作
}
- Improper exception handling leads to resource leakage: During exception handling, attention needs to be paid to the correct release of resources. When an exception occurs, the program jumps to the catch block, and the unexecuted code portion may cause resource leaks.
Sample code:
try {
// 动态分配内存
int* data = new int[100];
// 执行其他可能抛出异常的操作
// ...
delete[] data;
} catch (const std::exception& e) {
// 异常处理代码
// 未执行的代码部分可能导致资源泄漏
}
Conclusion:
C’s exception handling mechanism is a powerful control flow mechanism that can effectively handle errors and exceptions during program running. Reasonable use of exception handling mechanisms can improve the reliability and robustness of programs. However, in actual development, you need to pay attention to the correctness of catching and handling exceptions to avoid uncaught exceptions that cause the program to terminate. At the same time, you must also consider the correct release of resources during exception handling to avoid resource leaks.
The above is an analysis of the C exception handling mechanism and common problems. Through reasonable exception capture and processing, we can better handle abnormal situations during program operation, improve the reliability and stability of the program, and make the program more reliable and stable. The code is more robust.
The above is the detailed content of C++ exception handling mechanism and analysis of common problems. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn