


How to solve security authentication and authorization issues in PHP development
In PHP development, security authentication and authorization issues are very important, especially when it comes to users Login, access control and rights management. This article will introduce some methods to solve security authentication and authorization problems in PHP development, and provide specific code examples.
1. Security Authentication (Authentication)
Security authentication is the process of verifying the user's identity to ensure that the user is a legal visitor. In PHP development, we can use the session mechanism to implement security authentication. The following is a simple sample code:
// 启动会话 session_start(); // 用户登录验证 function authenticate($username, $password) { // 根据用户名和密码进行验证,比如从数据库查询用户信息 // 如果验证成功,设置会话变量 $_SESSION['username'] = $username; } // 用户注销 function logout() { // 清除会话变量 session_unset(); // 销毁会话 session_destroy(); } // 验证用户是否登录 function isLoggedin() { // 判断会话变量是否存在,即判断用户是否登录 return isset($_SESSION['username']); }
The above code demonstrates how to perform user authentication and logout, and determine whether the user is logged in. After successful login verification, user information is recorded by setting session variables.
2. Access Control
Access control restricts users’ access to resources based on user roles and permissions. In PHP development, we can implement access control through role and permission management. The following is a simple sample code:
// 用户角色定义 define('ROLE_ADMIN', 1); define('ROLE_USER', 2); // 资源访问权限定义 define('PERMISSION_VIEW', 1); define('PERMISSION_EDIT', 2); // 用户角色和权限关系定义 $rolePermissions = array( ROLE_ADMIN => array(PERMISSION_VIEW, PERMISSION_EDIT), ROLE_USER => array(PERMISSION_VIEW) ); // 检查用户是否有权限访问资源 function hasPermission($userRole, $requiredPermission) { global $rolePermissions; // 判断用户角色是否存在 if (!array_key_exists($userRole, $rolePermissions)) { return false; } // 判断用户角色是否拥有所需权限 return in_array($requiredPermission, $rolePermissions[$userRole]); } // 示例用法 if (hasPermission(ROLE_ADMIN, PERMISSION_VIEW)) { // 允许管理员查看资源 // 执行相关操作 } else { // 没有权限,给出提示或执行其他操作 }
The above code demonstrates how to control resource access based on user roles and permissions. By defining user roles and permissions, you can flexibly control different users' access to resources.
To sum up, security authentication and authorization are important issues that cannot be ignored in PHP development. Through reasonable security authentication and access control mechanisms, the security of users and resources can be protected. We hope that the code examples provided in this article will be helpful in solving security authentication and authorization issues in PHP development.
The above is the detailed content of How to solve security authentication and authorization issues in PHP development. For more information, please follow other related articles on the PHP Chinese website!
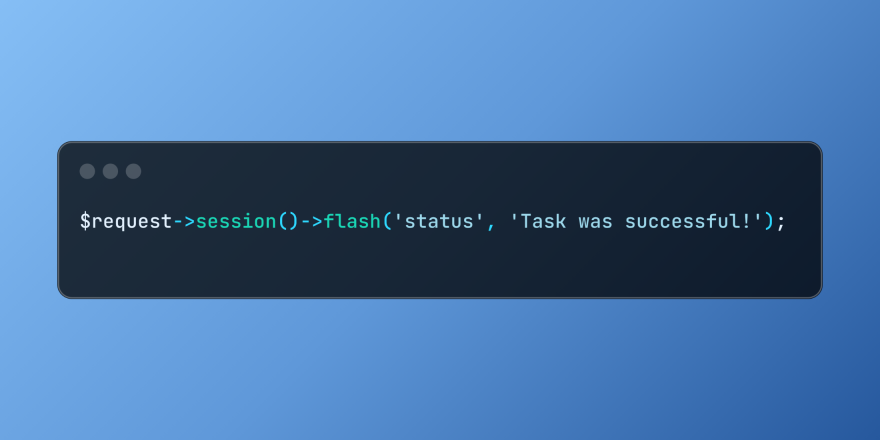
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
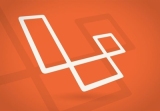
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
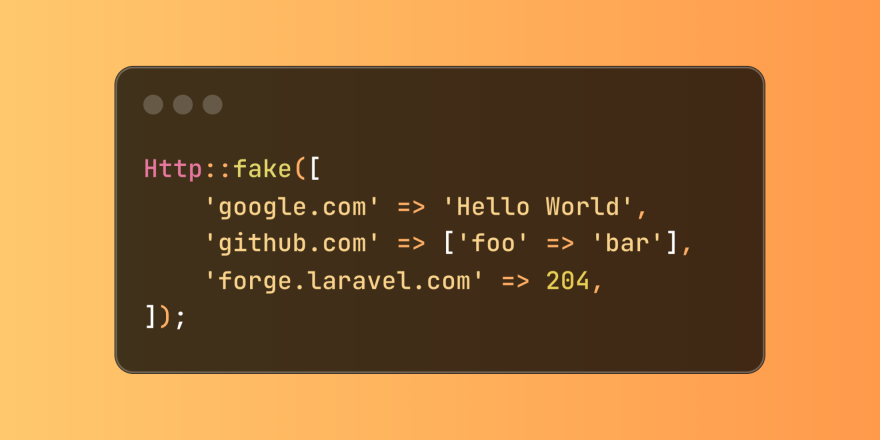
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
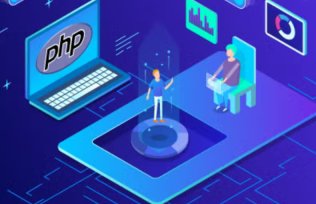
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
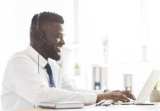
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
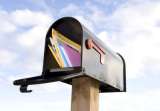
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov
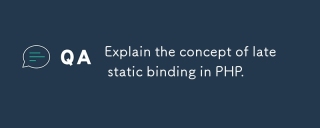
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
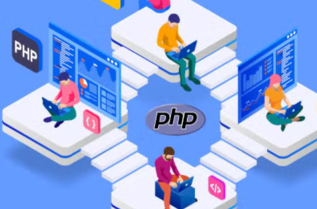
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
