Context generation issues in chatbots
Context generation issues and code examples in chatbots
Abstract: With the rapid development of artificial intelligence, chatbots, as an important application scenario, have been widely s concern. However, chatbots often lack contextual understanding when engaging in conversations with users, resulting in poor conversation quality. This article explores the problem of context generation in chatbots and addresses it with concrete code examples.
1. Introduction
Chat robot has important research and application value in the field of artificial intelligence. It can simulate conversations between people and realize natural language interaction. However, traditional chatbots often simply respond based on user input, lacking context understanding and memory capabilities. This makes the chatbot’s conversations seem incoherent and humane, and the user experience is relatively poor.
2. Cause of context generation problem
- Lack of context information. Traditional chatbot conversations only rely on the user's current input, cannot use previous conversation history as a reference, and lack contextual information about the conversation.
- Broken dialogue flow. Traditional chatbot responses only respond to the user's current input and are unable to carry out a conversation coherently, resulting in a broken conversation process.
3. Solutions to context generation
In order to solve the context generation problem in chatbots, we can use some technologies and algorithms to improve the conversational capabilities of chatbots.
- Use Recurrent Neural Network (RNN).
Recurrent neural network is a neural network structure that can process sequence data. By using the previous sentence as part of the current input, the RNN can remember contextual information and use it when generating answers. The following is a code example that uses RNN to handle conversation context:
import tensorflow as tf import numpy as np # 定义RNN模型 class ChatRNN(tf.keras.Model): def __init__(self): super(ChatRNN, self).__init__() self.embedding = tf.keras.layers.Embedding(VOCAB_SIZE, EMBEDDING_DIM) self.rnn = tf.keras.layers.GRU(EMBEDDING_DIM, return_sequences=True, return_state=True) self.fc = tf.keras.layers.Dense(VOCAB_SIZE) def call(self, inputs, training=False): x = self.embedding(inputs) x, state = self.rnn(x) output = self.fc(x) return output, state # 训练模型 model = ChatRNN() model.compile(optimizer='adam', loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True), metrics=['accuracy']) model.fit(x_train, y_train, epochs=10)
- Using the attention mechanism.
The attention mechanism allows the model to weight key information in the context when generating answers, improving the accuracy and coherence of answers. The following is a code example that uses the attention mechanism to process conversation context:
import tensorflow as tf import numpy as np # 定义注意力模型 class AttentionModel(tf.keras.Model): def __init__(self): super(AttentionModel, self).__init__() self.embedding = tf.keras.layers.Embedding(VOCAB_SIZE, EMBEDDING_DIM) self.attention = tf.keras.layers.Attention() self.fc = tf.keras.layers.Dense(VOCAB_SIZE) def call(self, inputs, training=False): x = self.embedding(inputs) x, attention_weights = self.attention(x, x) output = self.fc(x) return output, attention_weights # 训练模型 model = AttentionModel() model.compile(optimizer='adam', loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True), metrics=['accuracy']) model.fit(x_train, y_train, epochs=10)
IV. Summary
In practical applications, chat robots often need to have the ability to generate context to achieve a more natural, Smooth conversation experience. This article introduces the problem of context generation in chatbots and provides code examples that use RNN and attention mechanisms to solve the problem. By adding reference and weighting to conversation history, chatbots can better understand contextual information and generate coherent responses. These methods provide important ideas and methods for improving the conversational capabilities of chatbots.
References:
- Sutskever, I., Vinyals, O., & Le, Q. V. (2014). Sequence to sequence learning with neural networks. In Advances in neural information processing systems (pp. 3104-3112).
- Vaswani, A., Shazeer, N., Parmar, N., Uszkoreit, J., Jones, L., Gomez, A. N., ... & Polosukhin, I. (2017). Attention is all you need. In Advances in neural information processing systems (pp. 5998-6008).
- Zhou, Y., Zhang, H., & Wang, H. (2017 ). Emotional chatting machine: Emotional conversation generation with internal and external memory. In Proceedings of the 55th Annual Meeting of the Association for Computational Linguistics (Volume 1: Long Papers) (pp. 1318-1327).
The above is the detailed content of Context generation issues in chatbots. For more information, please follow other related articles on the PHP Chinese website!
![Can't use ChatGPT! Explaining the causes and solutions that can be tested immediately [Latest 2025]](https://img.php.cn/upload/article/001/242/473/174717025174979.jpg?x-oss-process=image/resize,p_40)
ChatGPT is not accessible? This article provides a variety of practical solutions! Many users may encounter problems such as inaccessibility or slow response when using ChatGPT on a daily basis. This article will guide you to solve these problems step by step based on different situations. Causes of ChatGPT's inaccessibility and preliminary troubleshooting First, we need to determine whether the problem lies in the OpenAI server side, or the user's own network or device problems. Please follow the steps below to troubleshoot: Step 1: Check the official status of OpenAI Visit the OpenAI Status page (status.openai.com) to see if the ChatGPT service is running normally. If a red or yellow alarm is displayed, it means Open
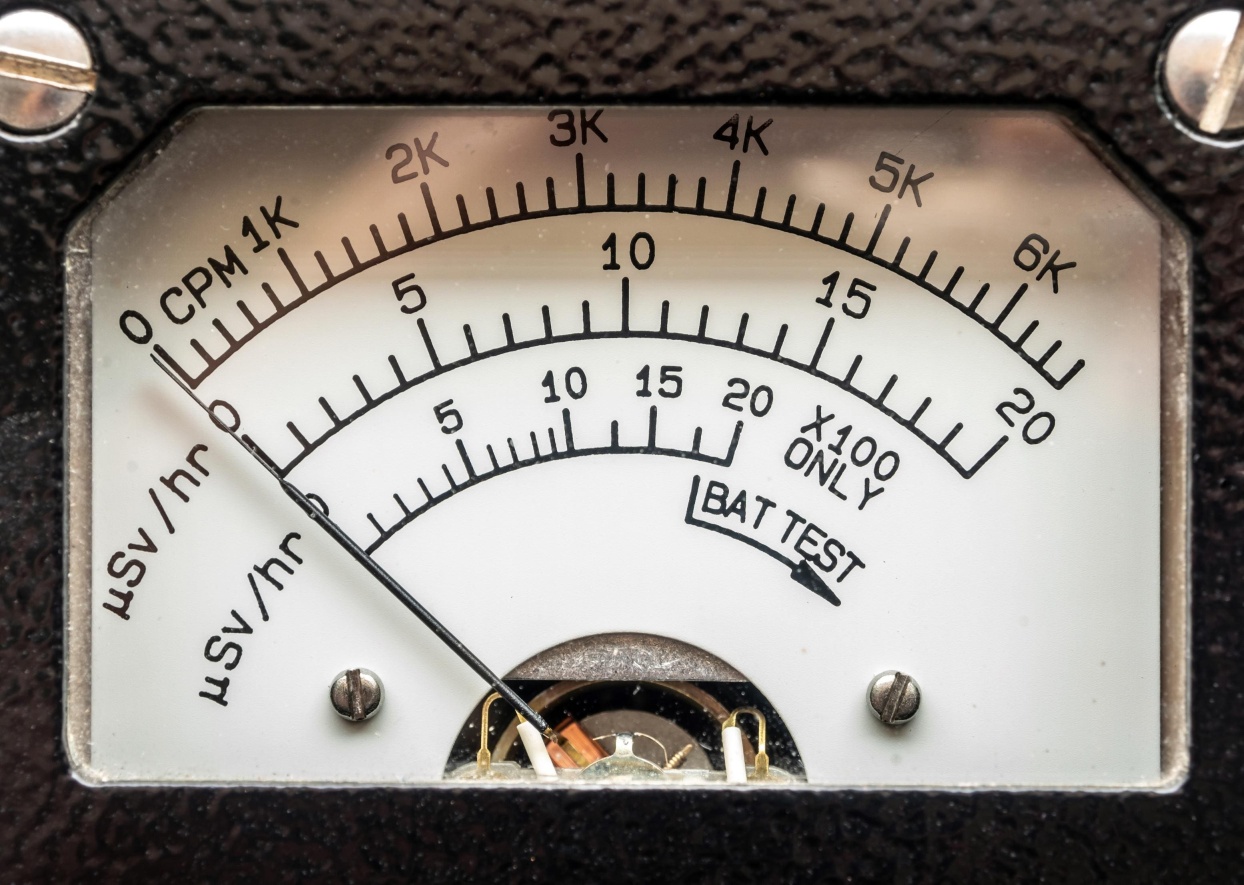
On 10 May 2025, MIT physicist Max Tegmark told The Guardian that AI labs should emulate Oppenheimer’s Trinity-test calculus before releasing Artificial Super-Intelligence. “My assessment is that the 'Compton constant', the probability that a race to

AI music creation technology is changing with each passing day. This article will use AI models such as ChatGPT as an example to explain in detail how to use AI to assist music creation, and explain it with actual cases. We will introduce how to create music through SunoAI, AI jukebox on Hugging Face, and Python's Music21 library. Through these technologies, everyone can easily create original music. However, it should be noted that the copyright issue of AI-generated content cannot be ignored, and you must be cautious when using it. Let’s explore the infinite possibilities of AI in the music field together! OpenAI's latest AI agent "OpenAI Deep Research" introduces: [ChatGPT]Ope

The emergence of ChatGPT-4 has greatly expanded the possibility of AI applications. Compared with GPT-3.5, ChatGPT-4 has significantly improved. It has powerful context comprehension capabilities and can also recognize and generate images. It is a universal AI assistant. It has shown great potential in many fields such as improving business efficiency and assisting creation. However, at the same time, we must also pay attention to the precautions in its use. This article will explain the characteristics of ChatGPT-4 in detail and introduce effective usage methods for different scenarios. The article contains skills to make full use of the latest AI technologies, please refer to it. OpenAI's latest AI agent, please click the link below for details of "OpenAI Deep Research"
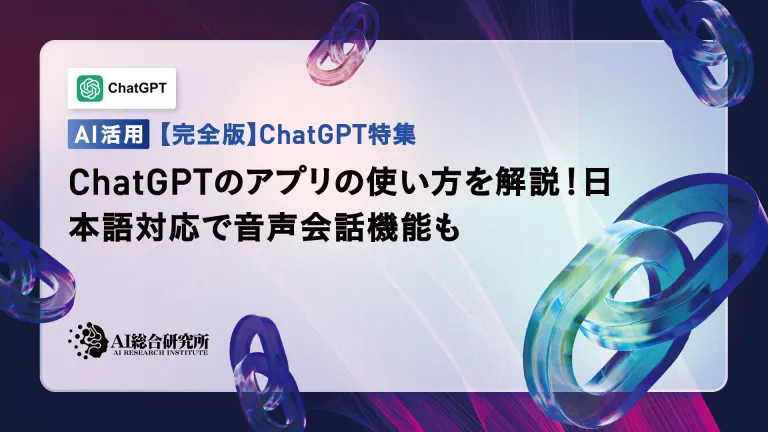
ChatGPT App: Unleash your creativity with the AI assistant! Beginner's Guide The ChatGPT app is an innovative AI assistant that handles a wide range of tasks, including writing, translation, and question answering. It is a tool with endless possibilities that is useful for creative activities and information gathering. In this article, we will explain in an easy-to-understand way for beginners, from how to install the ChatGPT smartphone app, to the features unique to apps such as voice input functions and plugins, as well as the points to keep in mind when using the app. We'll also be taking a closer look at plugin restrictions and device-to-device configuration synchronization
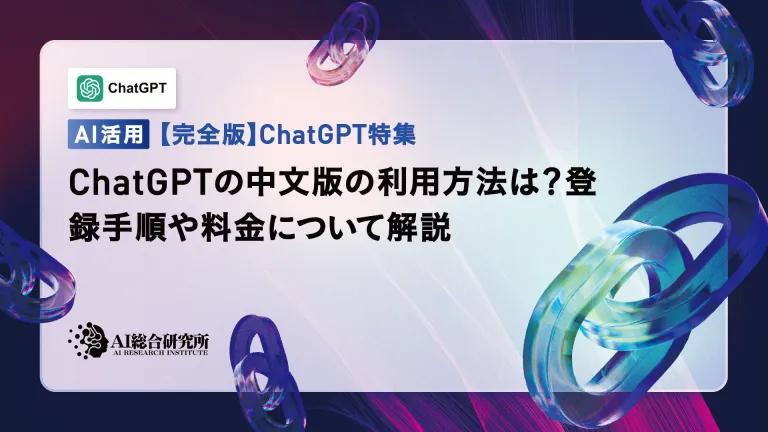
ChatGPT Chinese version: Unlock new experience of Chinese AI dialogue ChatGPT is popular all over the world, did you know it also offers a Chinese version? This powerful AI tool not only supports daily conversations, but also handles professional content and is compatible with Simplified and Traditional Chinese. Whether it is a user in China or a friend who is learning Chinese, you can benefit from it. This article will introduce in detail how to use ChatGPT Chinese version, including account settings, Chinese prompt word input, filter use, and selection of different packages, and analyze potential risks and response strategies. In addition, we will also compare ChatGPT Chinese version with other Chinese AI tools to help you better understand its advantages and application scenarios. OpenAI's latest AI intelligence
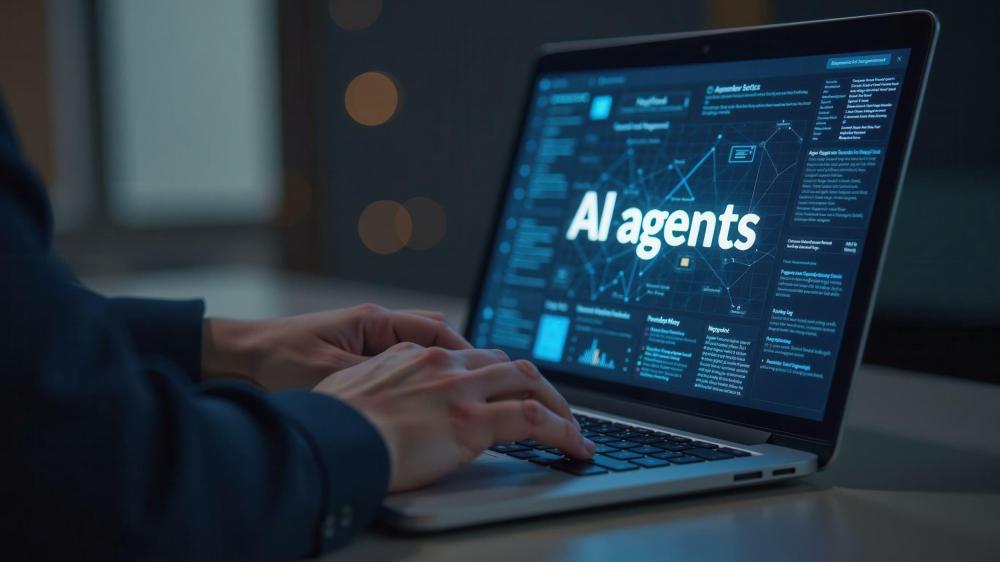
These can be thought of as the next leap forward in the field of generative AI, which gave us ChatGPT and other large-language-model chatbots. Rather than simply answering questions or generating information, they can take action on our behalf, inter
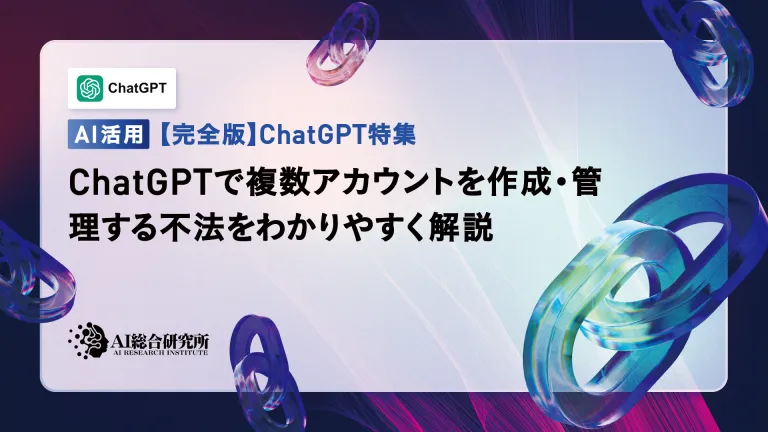
Efficient multiple account management techniques using ChatGPT | A thorough explanation of how to use business and private life! ChatGPT is used in a variety of situations, but some people may be worried about managing multiple accounts. This article will explain in detail how to create multiple accounts for ChatGPT, what to do when using it, and how to operate it safely and efficiently. We also cover important points such as the difference in business and private use, and complying with OpenAI's terms of use, and provide a guide to help you safely utilize multiple accounts. OpenAI


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
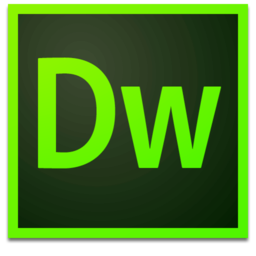
Dreamweaver Mac version
Visual web development tools
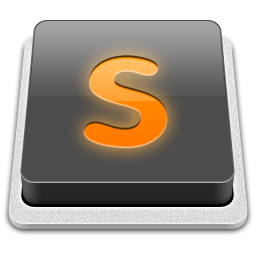
SublimeText3 Mac version
God-level code editing software (SublimeText3)
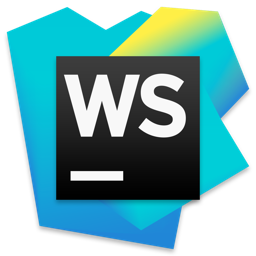
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
