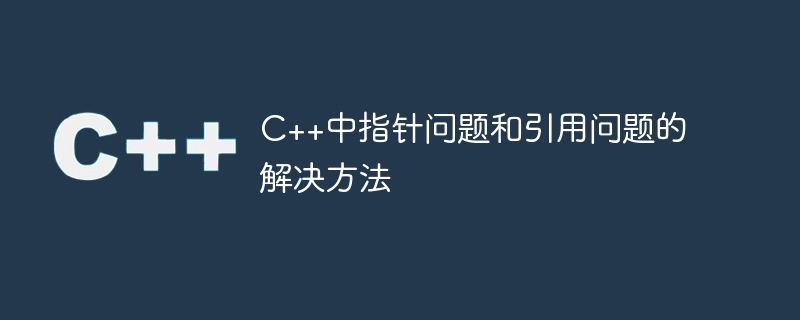
Solutions to pointer problems and reference problems in C
Introduction:
In the process of C programming, pointer problems and reference problems are common problems that trouble programmers difficult problem. This article will introduce some methods to solve these problems and illustrate them through specific code examples to help readers better understand and apply them.
1. Solution to the pointer problem
- Null pointer detection
Before using a pointer, be sure to perform a null pointer detection to prevent program crashes caused by a null pointer. The following is a sample code:
int main()
{
int* ptr = nullptr; // 初始化为nullptr
if (ptr != nullptr) // 空指针检测
{
*ptr = 10; // 使用指针前先进行检测
}
return 0;
}
- Avoid wild pointers
Wild pointers refer to pointers pointing to unknown memory addresses or freed memory addresses. In order to avoid wild pointers, you can use the following methods:
- Set the pointer to nullptr after it is used to avoid using it again;
- Do not use uninitialized pointers to avoid unknown pointers. ;
- Avoid using released memory, and promptly release and delete the memory pointed to by the pointer.
- Memory leak problem
Memory leak means that the memory allocated during the running of the program cannot be released, resulting in continuous occupation of system resources. In order to avoid memory leaks, you can use the following methods:
- Release memory in time after allocating memory;
- Use smart pointers (such as std::shared_ptr and std::unique_ptr) to manage dynamic memory to avoid The tedious operation of manually freeing memory.
2. Solution to the reference problem
- Initialization of the reference
The reference must be initialized when it is declared, and the point of the reference cannot be changed. If the reference points to an uninitialized variable, undefined behavior will result. The following is a sample code:
int main()
{
int a = 10;
int& ref = a; // 引用初始化为a
int& ref2; // 错误,引用必须进行初始化
return 0;
}
- Reference as function parameter
When the function is defined, the reference can be passed to the function as a parameter, which can avoid copying data when the function is called. . The following is a sample code:
void swap(int& a, int& b)
{
int temp = a;
a = b;
b = temp;
}
int main()
{
int x = 10, y = 20;
swap(x, y); // 使用引用作为函数参数进行值的交换
return 0;
}
- Conversion of references and pointers
There is a difference between references and pointers, but sometimes it is necessary to convert references to pointers or pointers to references. . In C, you can use the &
and *
operators for conversion. The following is a sample code:
int main()
{
int a = 10;
int* ptr = &a; // 将a的地址赋给指针ptr
int& ref = *ptr; // 将指针ptr所指向的值赋给引用ref
return 0;
}
Conclusion:
Pointer problems and reference problems are common problems in the C programming process. Pointer problems can be effectively solved by performing null pointer detection and avoiding wild pointers and memory leaks. Reference problems can be solved by properly initializing references, using references as function parameters, and converting references to pointers. I hope this article will be helpful to readers when solving pointer problems and reference problems in C, and will deepen their understanding of these problems.
The above is the detailed content of Solutions to pointer and reference problems in C++. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn