How to store and manage data locally in Vue projects
Local storage and management of data in the Vue project is very important. You can use the local storage API provided by the browser to achieve persistent storage of data. This article will introduce how to use localStorage in Vue projects for local storage and management of data, and provide specific code examples.
- Initializing data
In the Vue project, you first need to initialize the data that needs to be stored locally. You can define initial data in the data option of the Vue component and check whether locally stored data already exists through the created hook function. If it exists, assign the local data to the component's data.
data() { return { myData: '' } }, created() { const localData = localStorage.getItem('myData') if (localData) { this.myData = JSON.parse(localData) } }
- Save data
When the data changes, the new data needs to be saved to local storage. You can monitor data changes through Vue's watch option, and call the setItem method of localStorage in the callback function to save the data to local storage.
watch: { myData: { handler(newData) { localStorage.setItem('myData', JSON.stringify(newData)) }, deep: true } }
- Clear data
If you need to clear locally stored data, you can do so by calling the removeItem method of localStorage.
methods: { clearData() { localStorage.removeItem('myData') this.myData = '' } }
- Other operations
In addition to saving and clearing data, you can also perform some other operations, such as obtaining the amount of locally stored data.
methods: { getDataCount() { return localStorage.length } }
- Notes
When using localStorage for local storage of data, you need to pay attention to the following points:
- localStorage can only store String type data, so when saving and loading data, you need to use JSON.stringify and JSON.parse for conversion.
- In order to avoid conflicts caused by multiple components modifying the same data at the same time, you can use Vue's deep monitoring option (deep: true) to monitor changes in objects or arrays.
- If you need to retain data after the user closes the browser, you can use sessionStorage instead of localStorage.
Summary:
In the Vue project, it is very convenient to use localStorage for local storage and management of data. By initializing data, saving data, clearing data and other operations, you can achieve persistent storage of data and ensure data consistency and integrity. The code examples provided above can help you quickly apply them in actual projects.
The above is the detailed content of How to store and manage data locally in Vue projects. For more information, please follow other related articles on the PHP Chinese website!

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
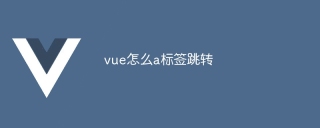
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
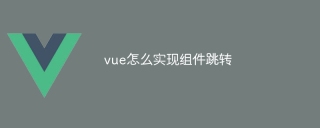
There are the following methods to implement component jump in Vue: use router-link and <router-view> components to perform hyperlink jump, and specify the :to attribute as the target path. Use the <router-view> component directly to display the currently routed rendered components. Use the router.push() and router.replace() methods for programmatic navigation. The former saves history and the latter replaces the current route without leaving records.
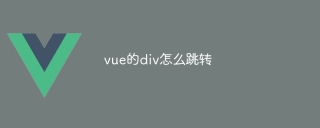
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
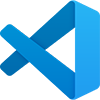
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
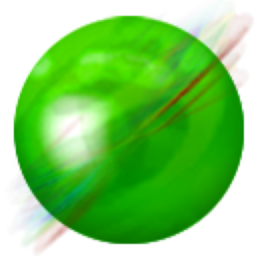
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
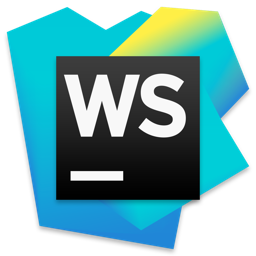
WebStorm Mac version
Useful JavaScript development tools