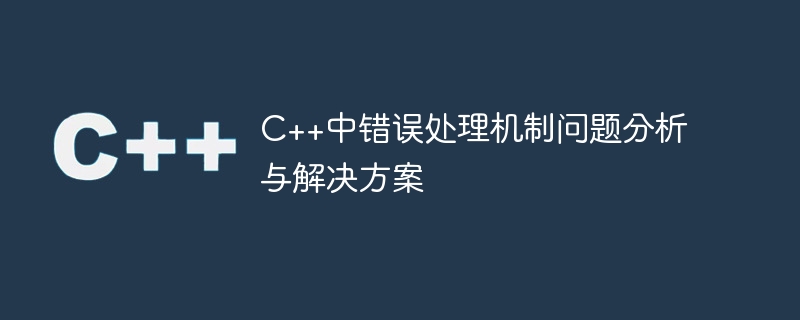
Analysis and solutions to error handling mechanism problems in C
Introduction:
Error handling is a very important part of the software development process and can help developers diagnose , debug and fix bugs in software. In C, the error handling mechanism is mainly implemented through exception handling. However, exception handling may also cause some problems. This article will analyze the error handling mechanism problems in C and provide some solutions.
1. Disadvantages of exception handling
Exception handling is a very powerful error handling mechanism, but it is not without its shortcomings. The following are some common problems:
- Performance overhead caused by exception handling
Exception handling will bring a certain performance overhead because it requires additional resources to maintain the exception context and perform stack unwinding . In performance-sensitive applications, excessive exception handling may cause the program to slow down.
- Conflict between exceptions and destructors
The exception handling mechanism in C relies on the automatic calling of the destructor, but in some cases the destructor may cause an exception, leading to unpredictable behavior of the program.
- Irregular design and use of exception handling
Irregular design and use of exception handling may lead to reduced readability and maintainability of the code. For example, abusing exception handling to control program flow, ignoring exceptions, etc. is not recommended.
2. Solutions
In view of the above problems, the following are some common solutions:
- Reasonable use of exception handling
Exception handling should be used reasonably Use it only to handle real exceptions. For normal control flow, other means should be used to avoid using exceptions, such as returning error codes or using conditional statements.
- Optimize exception handling
In order to reduce the performance overhead caused by exception handling, you can consider using lightweight alternatives to exception handling, such as error code return.
- Avoid throwing exceptions in the destructor
In order to avoid uncertain behavior caused by exceptions thrown in the destructor, you can capture and record the exception in the destructor instead of throwing it again out. In addition, you can also consider using RAII (resource acquisition i.e. initialization) technology such as smart pointers to ensure the correct release of resources.
The following are some specific sample codes:
[Code sample 1]
try {
// 执行可能引发异常的代码
} catch (const std::exception& e) {
// 处理异常
std::cerr << "Exception: " << e.what() << std::endl;
} catch (...) {
// 处理未知异常
std::cerr << "Unknown exception!" << std::endl;
}
[Code sample 2]
class Resource {
public:
Resource() {
// 打开资源
}
~Resource() noexcept {
try {
// 关闭资源
} catch (...) {
// 处理关闭资源时可能引发的异常
// 记录日志或进行其他处理
}
}
// ...
};
Conclusion: ## The exception handling mechanism in #C is a powerful error handling method, but there are some issues that need to be paid attention to when using it. By rationally using exception handling, optimizing exception handling and avoiding throwing exceptions in destructors, the error handling mechanism problem in C can be effectively solved.
In short, error handling is an indispensable part of the development process. Correct and standardized use of the exception handling mechanism can help us write more stable and reliable C programs.
The above is the detailed content of Analysis and solutions to error handling mechanism problems in C++. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn