How to handle concurrent task retries in Go language?
In concurrent programming, task retry is a common problem. When a task fails, we may want to re-execute the task until it succeeds. The concurrency model of the Go language makes it relatively simple to deal with concurrent task retries. This article will introduce how to handle concurrent task retries in the Go language and provide specific code examples.
1. Use goroutine and channel for concurrent task execution
In the Go language, we can use goroutine and channel to implement concurrent task execution. Goroutine is a lightweight thread that can create multiple goroutines in the code to perform tasks. Channel is a mechanism used for communication between goroutines. By putting tasks into a channel, different goroutines can execute tasks concurrently.
The following is a simple sample code that shows how to use goroutine and channel to execute tasks concurrently:
func worker(tasks <-chan int, results chan<- int) { for task := range tasks { // 执行任务的逻辑,省略具体实现 result := executeTask(task) results <- result } } func main() { tasks := make(chan int, 100) results := make(chan int, 100) // 创建多个goroutine来执行任务 for i := 0; i < 10; i++ { go worker(tasks, results) } // 初始化任务队列 for i := 0; i < 100; i++ { tasks <- i } close(tasks) // 获取任务执行结果 for i := 0; i < 100; i++ { result := <-results // 处理任务结果的逻辑,省略具体实现 handleResult(result) } close(results) // 其他后续操作 }
In the above code, we use two channels: tasks and results. tasks are used to pass the tasks to be executed, and results are used to pass the execution results of the tasks. By placing tasks into tasks, executing tasks concurrently through multiple goroutines, and finally obtaining the execution results of the tasks through results.
2. Handling task retry issues
When dealing with concurrent task retry issues, you can use the features of goroutine and channel to achieve this. When a task fails to execute, we can put the task back into the task queue and execute it again. The following is a sample code that shows how to handle concurrent task retry issues:
func worker(tasks <-chan int, results chan<- int) { for task := range tasks { // 执行任务的逻辑,省略具体实现 result := executeTask(task) if result < 0 { // 任务执行失败,需要进行重试 tasks <- task } else { results <- result } } } func main() { tasks := make(chan int, 100) results := make(chan int, 100) // 创建多个goroutine来执行任务 for i := 0; i < 10; i++ { go worker(tasks, results) } // 初始化任务队列 for i := 0; i < 100; i++ { tasks <- i } close(tasks) // 获取任务执行结果 for i := 0; i < 100; i++ { result := <-results if result < 0 { // 任务执行失败,需要进行重试 tasks <- i } else { // 处理任务结果的逻辑,省略具体实现 handleResult(result) } } close(results) // 其他后续操作 }
In the above code, when a task fails to execute, we put the task back into the task queue and execute it again. This achieves retry of concurrent tasks. Note that we need to choose the right time to put the task back into the task queue to avoid an infinite loop.
Summary:
This article introduces how to handle concurrent task retry issues in the Go language and provides specific code examples. By leveraging the features of goroutine and channel, we can implement retry of concurrent tasks relatively simply. This is very helpful for improving the fault tolerance and reliability of the program. In actual development, we can adjust the code according to specific needs to adapt to different scenarios.
The above is the detailed content of How to deal with concurrent task retries in Go language?. For more information, please follow other related articles on the PHP Chinese website!
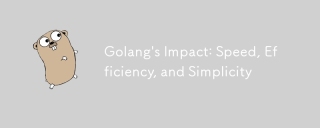
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
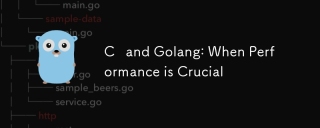
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
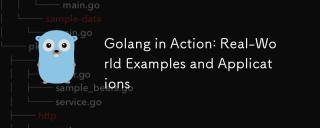
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
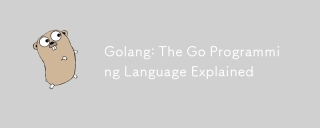
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
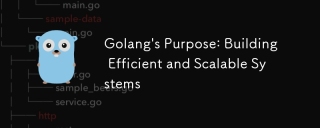
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
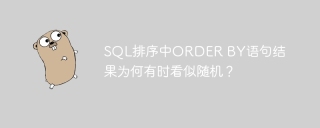
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
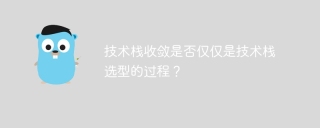
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
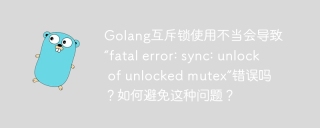
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
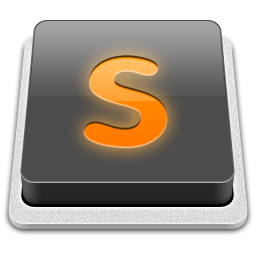
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
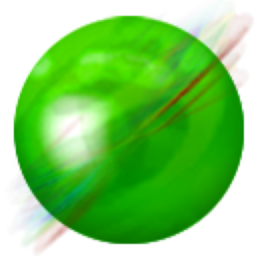
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment