How to solve network latency issues in Java
How to solve the network delay problem in Java
Network delay refers to the delay that occurs between the sending and receiving of data due to various reasons during the data transmission process. time delay. When conducting network communications or developing network applications, we often encounter network latency problems. This article will introduce some methods to solve network latency problems in Java and provide specific code examples.
1. Use multi-threading
Network delay is usually caused by the blocking of network requests. In order to avoid network requests blocking the main thread, we can use multi-threads to handle network requests. The main thread is responsible for displaying the user interface, while network requests are performed in sub-threads. This allows multiple network requests to be made at the same time and improves the response speed of the program.
The following is a sample code that uses multi-threading to process network requests:
public class NetworkRequestThread extends Thread { private String url; public NetworkRequestThread(String url) { this.url = url; } @Override public void run() { // 发送网络请求 HttpURLConnection connection = null; try { URL urlObj = new URL(url); connection = (HttpURLConnection) urlObj.openConnection(); // 设置请求超时时间 connection.setConnectTimeout(5000); connection.setReadTimeout(5000); // 请求数据并处理结果 int responseCode = connection.getResponseCode(); if (responseCode == HttpURLConnection.HTTP_OK) { // 读取数据 BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream())); String line; StringBuilder response = new StringBuilder(); while ((line = reader.readLine()) != null) { response.append(line); } reader.close(); // 处理数据 handleResponse(response.toString()); } } catch (Exception e) { e.printStackTrace(); } finally { if (connection != null) { connection.disconnect(); } } } private void handleResponse(String response) { // 处理网络请求返回的数据 // ... } } public class MainThread { public static void main(String[] args) { String url1 = "http://example.com/api1"; String url2 = "http://example.com/api2"; String url3 = "http://example.com/api3"; // 创建并启动多个线程 NetworkRequestThread thread1 = new NetworkRequestThread(url1); NetworkRequestThread thread2 = new NetworkRequestThread(url2); NetworkRequestThread thread3 = new NetworkRequestThread(url3); thread1.start(); thread2.start(); thread3.start(); } }
In the above sample code, we created a NetworkRequestThread class that inherits from the Thread class, and in its run method Make a network request and process the result of the network request in the handleResponse method. In the main thread, we create multiple NetworkRequestThread objects and start these threads so that multiple network requests can be made at the same time.
2. Use connection pool
Network delay is usually related to the establishment and release of network connections. In order to avoid delays caused by frequent establishment and release of network connections, we can use connection pools to manage network connections.
The connection pool maintains a set of reusable network connections. When a network request needs to be sent, the connection is obtained from the connection pool and used. After the request is completed, the connection is returned to the connection pool, which can reduce the establishment and The number of times the connection is released to improve the response speed of the program.
The following is a sample code that uses a connection pool to handle network requests:
public class NetworkRequest { private static final int MAX_CONNECTIONS = 10; private static final int CONNECTION_TIMEOUT = 5000; private static final int SO_TIMEOUT = 5000; private static HttpClient httpClient; static { PoolingHttpClientConnectionManager connectionManager = new PoolingHttpClientConnectionManager(); connectionManager.setMaxTotal(MAX_CONNECTIONS); connectionManager.setDefaultMaxPerRoute(MAX_CONNECTIONS); RequestConfig requestConfig = RequestConfig.custom() .setConnectTimeout(CONNECTION_TIMEOUT) .setSocketTimeout(SO_TIMEOUT) .build(); httpClient = HttpClientBuilder.create() .setConnectionManager(connectionManager) .setDefaultRequestConfig(requestConfig) .build(); } public static String sendHttpRequest(String url) throws IOException { HttpGet get = new HttpGet(url); try (CloseableHttpResponse response = httpClient.execute(get)) { HttpEntity entity = response.getEntity(); return EntityUtils.toString(entity); } } public static void main(String[] args) { String url1 = "http://example.com/api1"; String url2 = "http://example.com/api2"; String url3 = "http://example.com/api3"; try { String response1 = sendHttpRequest(url1); String response2 = sendHttpRequest(url2); String response3 = sendHttpRequest(url3); // 处理网络请求返回的数据 // ... } catch (IOException e) { e.printStackTrace(); } } }
In the above sample code, we use the HttpClient provided by the Apache HttpComponents component library to send network requests. In the static code block, we created a connection pool and HttpClient object, and set the maximum number of connections in the connection pool, the connection timeout and the Socket timeout.
In the sendHttpRequest method, we use HttpGet to send the network request and close the connection after the request is completed. In the main method, we directly call the sendHttpRequest method to send the network request and process the returned data.
Conclusion:
This article introduces how to solve the network delay problem in Java, including using multi-threading to process network requests and using connection pools to manage network connections. These methods can effectively improve the response speed and performance of the program. Hope this article is helpful to you.
The above is the detailed content of How to solve network latency issues in Java. For more information, please follow other related articles on the PHP Chinese website!
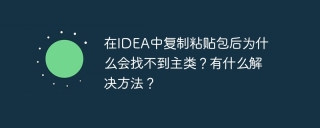
Why can't the main class be found after copying and pasting the package in IDEA? Using IntelliJIDEA...
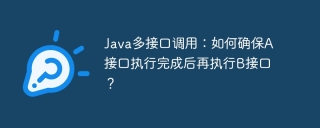
State synchronization between Java multi-interface calls: How to ensure that interface A is called after it is executed? In Java development, you often encounter multiple calls...
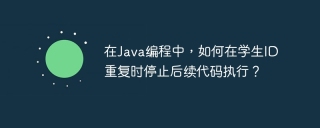
How to stop subsequent code execution when ID is repeated in Java programming. When learning Java programming, you often encounter such a requirement: when a certain condition is met,...
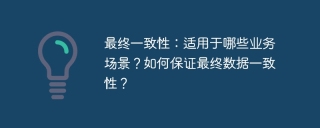
In-depth discussion of final consistency: In the distributed system of application scenarios and implementation methods, ensuring data consistency has always been a major challenge for developers. This article...
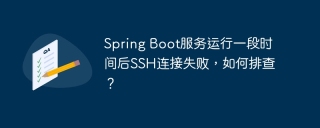
The troubleshooting idea of SSH connection failure after SpringBoot service has been running for a period of time has recently encountered a problem: a Spring...
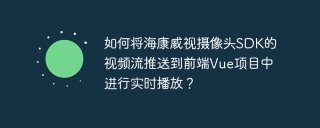
How to push video streams from Hikvision camera SDK to front-end Vue project? During the development process, you often encounter videos that need to be captured by the camera to be circulated...
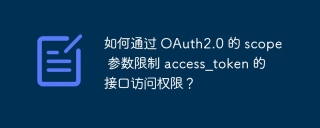
How to use access_token of OAuth2.0 to restrict interface access permissions How to ensure access_token when authorizing using OAuth2.0...
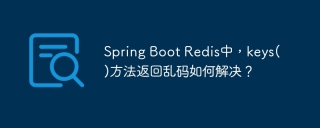
SpringBootRedis gets the key garbled problem analysis using Spring...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
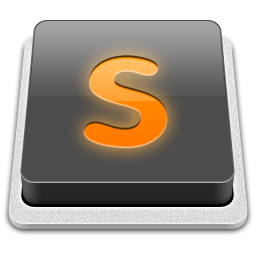
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.