Analysis and solutions to operator overloading problems in C
Overview:
In C, operator overloading is a powerful feature that allows users to modify existing The operators are redefined to suit a specific data type. However, when using operator overloading, you may encounter some problems, such as conflicts between multiple operator overloaded functions, operator overloaded functions failing to match the expected operand type, etc. This article will discuss these issues and provide solutions.
1. Conflict of operator overloading functions
When overloading an operator, we can define multiple different operator overloading functions for it (which can have different number of parameters or parameter types) . However, in some cases, a conflict between multiple operator overloaded functions may occur, causing the compiler to be unable to determine which function to use.
Solution:
- Explicitly specify parameter types
Conflicts between operator overloaded functions can be resolved by explicitly specifying parameter types. For example, for addition operator overloaded functions, they can be defined as overloaded functions with different parameter types such as int type, float type, etc. to distinguish different uses. - Use different operand order
The operand order of some operators can affect the result. For example, the addition operator overloaded function can be defined in two different orders: a b and b a to distinguish different semantics. This way, you can avoid conflicts with other overloaded functions when using operators.
2. Operator overloaded function cannot match the expected operand type
When overloading an operator, sometimes there may be a problem that the expected operand type cannot be matched, resulting in compilation mistake.
Solution:
- Type conversion
You can convert the operand to the type expected by the overloaded function by defining a type conversion function. For example, for a custom class, if you want to overload the addition operator, you can define a type conversion function that converts other types into the type of the class to achieve matching of the overloaded functions. - Use friend functions
If when overloading operators, the expected operand type matching cannot be achieved through member functions inside the class, you can consider using friend functions. Friend functions can directly access private members of a class and operate operand types more freely.
Code example:
Take the custom Complex class as an example to demonstrate the problem analysis and solution of operator overloading.
class Complex { private: int real; int imag; public: Complex(int r, int i) : real(r), imag(i) {} Complex operator+(const Complex& other) { Complex result(real + other.real, imag + other.imag); return result; } }; int main() { Complex c1(1, 2); Complex c2(3, 4); Complex c3 = c1 + c2; // 编译错误,无法匹配到预期的操作数类型 return 0; }
In the above example, we defined a Complex class and tried to overload the addition operator. However, when using the addition operator, a compilation error occurs because the parameter type of the overloaded function is const Complex&, and the type of the operands c1 and c2 is Complex. In order to solve this problem, you can define a type conversion function in the Complex class to convert other types into the Complex type.
class Complex { private: int real; int imag; public: Complex(int r, int i) : real(r), imag(i) {} Complex operator+(const Complex& other) { Complex result(real + other.real, imag + other.imag); return result; } Complex(int r) : real(r), imag(0) {} }; int main() { Complex c1(1, 2); Complex c2(3, 4); Complex c3 = c1 + Complex(5); // 正常运行 return 0; }
In the modified example, we define a constructor that converts the int type to the Complex type, so that 5 can be converted to the Complex type and the operation can be performed smoothly.
Conclusion:
Operator overloading is a powerful feature of C, but we may encounter some problems during use. By explicitly specifying parameter types, using different operand orders, defining type conversion functions, or using friend functions, you can solve the problem of operator overloaded function conflicts and inability to match expected operand types, and improve program readability and flexibility. sex.
The above is the detailed content of Analysis and solutions to operator overloading problems in C++. For more information, please follow other related articles on the PHP Chinese website!
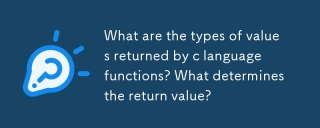
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
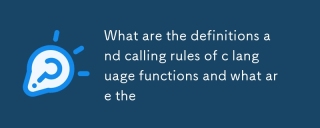
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
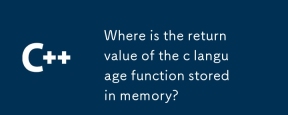
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
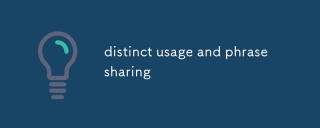
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
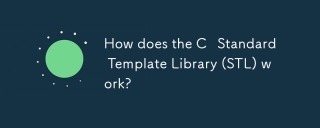
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
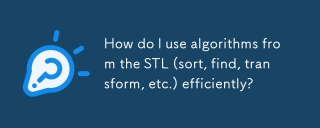
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
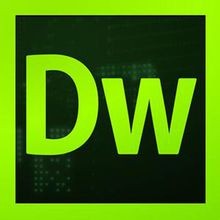
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
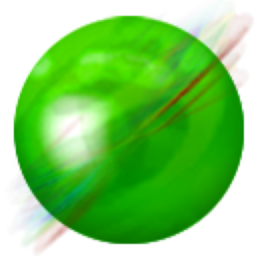
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
