


Data visualization and chart display problems encountered in Vue development
In Vue development, data visualization and chart display are very common requirements. Through visualization and chart display, we can more intuitively understand the distribution, trend and correlation of data, so as to better conduct data analysis and decision support.
However, we will also face some challenges and problems when implementing data visualization and chart display. Below I will use specific code examples to introduce some data visualization and chart display problems I encountered in Vue development, and provide corresponding solutions.
- How to obtain and process data
In data visualization and chart display, you first need to obtain and process data. Vue provides many convenient methods to obtain and process data, such as using the Axios library to send asynchronous requests to obtain data, and using the computed attribute to process data. The following is an example:
<template> <div> <button @click="fetchData">获取数据</button> <ul> <li v-for="item in data" :key="item.id">{{ item.name }}</li> </ul> </div> </template> <script> import axios from 'axios'; export default { data() { return { data: [] }; }, methods: { fetchData() { axios.get('https://api.example.com/data').then(response => { this.data = response.data; }).catch(error => { console.error(error); }); } } } </script>
In this example, we use the Axios library to send an asynchronous request to obtain data, then store the obtained data in the data attribute, and use the v-for instruction to display the data on the page superior.
- How to use common chart libraries
In Vue development, some chart libraries are often used to achieve data visualization and chart display, such as Echarts, Highcharts, etc. These chart libraries provide a wealth of chart types and configuration options to meet various data presentation needs. The following is an example of using the Echarts library to display a histogram:
<template> <div> <div ref="chart" style="width: 400px; height: 300px;"></div> </div> </template> <script> import echarts from 'echarts'; export default { mounted() { this.renderChart(); }, methods: { renderChart() { const chart = echarts.init(this.$refs.chart); chart.setOption({ xAxis: { type: 'category', data: ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun'] }, yAxis: { type: 'value' }, series: [{ data: [820, 932, 901, 934, 1290, 1330, 1320], type: 'bar' }] }); } } } </script>
In this example, we first initialize the Echarts instance in the mounted life cycle hook, and obtain the DOM of the chart div through this.$refs.chart Element, when rendering the chart, we call the setOption method to configure the data and style of the chart.
- How to dynamically update charts
Sometimes, our data changes dynamically and we need to update charts in real time. In Vue development, we can use the watch attribute to monitor data changes and re-render the chart when the data changes. The following is an example of dynamically updating a histogram:
<template> <div> <button @click="updateData">更新数据</button> <div ref="chart" style="width: 400px; height: 300px;"></div> </div> </template> <script> import echarts from 'echarts'; export default { data() { return { data: [820, 932, 901, 934, 1290, 1330, 1320] }; }, mounted() { this.renderChart(); }, methods: { renderChart() { const chart = echarts.init(this.$refs.chart); chart.setOption({ xAxis: { type: 'category', data: ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun'] }, yAxis: { type: 'value' }, series: [{ data: this.data, type: 'bar' }] }); }, updateData() { // 模拟数据更新 for(let i = 0; i < this.data.length; i++) { this.data[i] = Math.round(Math.random() * 1000); } } }, watch: { data() { this.renderChart(); } } } </script>
In this example, we use the watch attribute to monitor changes in data data, and automatically re-render the chart when the data data changes. In the updateData method, we simulated the update of data, updated the data by reassigning this.data, and triggered the watch method to re-render the chart.
Summary
In Vue development, data visualization and chart display are a very important aspect. By properly obtaining and processing data, using common chart libraries, and dynamically updating charts, we can well achieve the needs of data visualization and chart display. Through the visual display of data, we can understand and analyze the data more intuitively, so as to make better decisions and optimization.
The above is the detailed content of Data visualization and chart display problems encountered in using Vue development. For more information, please follow other related articles on the PHP Chinese website!
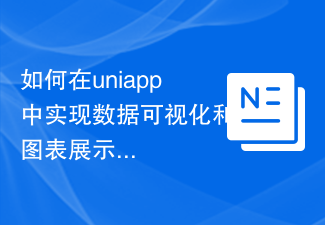
如何在uniapp中实现数据可视化和图表展示数据可视化和图表展示对于分析和展示数据是非常重要的。Uniapp是一款基于Vue.js的跨平台开发框架,可以在一次编写后同时发布到多个平台,包括iOS、Android、Web等,非常适合开发移动应用程序。本文将介绍如何在Uniapp中实现数据可视化和图表展示,并提供具体的代码示例。安装依赖首先,我们需要安装一些图表
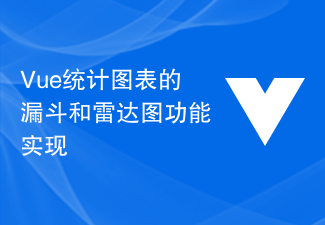
Vue统计图表的漏斗和雷达图功能实现引言:随着数据可视化需求的不断增加,统计图表成为了前端开发中的重要组件之一。本文将介绍如何使用Vue框架实现两种常见的统计图表,即漏斗图和雷达图。代码示例将详细展示如何使用Vue和相应的图表库来实现这两种图表。一、漏斗图功能实现漏斗图可以用于展示多个环节之间的数据流动情况,通常用于分析转化率或者漏斗模型。下面将介绍如何使用
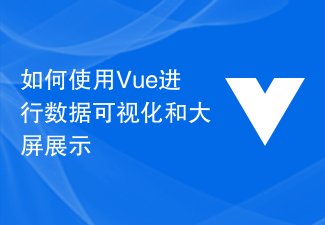
如何使用Vue进行数据可视化和大屏展示引言:随着信息时代的快速发展,数据可视化与大屏展示成为了越来越重要的需求。Vue.js作为一款流行的JavaScript框架,为我们提供了便捷的工具和组件来进行数据可视化和大屏展示。本文将介绍如何使用Vue进行数据可视化和大屏展示,并给出相关的代码示例。一、数据可视化安装依赖在开始使用Vue进行数据可视化之前,我们需要安
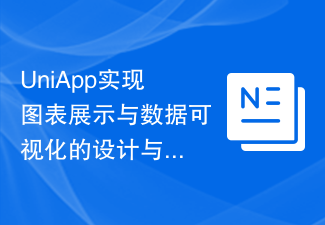
UniApp实现图表展示与数据可视化的设计与开发实践引言:随着大数据时代的到来,数据可视化成为了企业和个人分析数据的必备工具之一。在移动应用开发中,如何在小屏幕上展示丰富的数据图表,成为了开发者面临的挑战之一。本文将介绍如何利用UniApp框架,实现图表展示与数据可视化的设计与开发实践。一、UniApp简介UniApp是一个基于Vue.js的多端开发框架,可
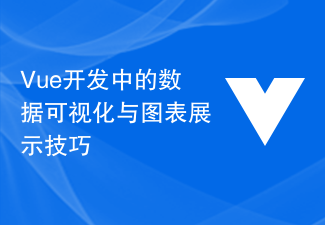
随着大数据时代的到来,数据可视化和图表展示成为了越来越多Web应用程序的必备功能。Vue作为一款颇受欢迎的JavaScript框架,也提供了丰富的工具和技巧来帮助开发者实现数据可视化和图表展示。在本文中,我们将介绍一些常用的数据可视化和图表展示技巧,帮助Vue开发者构建出更加可视化和直观的Web应用程序。使用Vue.js+EchartsEcharts是一个基
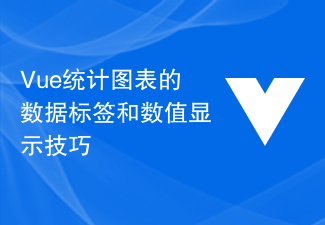
Vue统计图表的数据标签和数值显示技巧在开发Web应用程序时,统计图表是非常重要的数据呈现方式。Vue是一种流行的JavaScript框架,它提供了许多方便的功能来处理和展示数据。在这篇文章中,我们将探讨如何使用Vue来添加数据标签和数值显示到统计图表中。使用数据标签数据标签是指在图表上显示数据对应的值。它们可以帮助用户更清楚地理解图表的内容。Vue提供了一
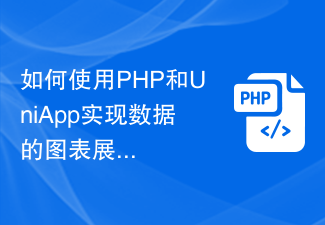
如何使用PHP和UniApp实现数据的图表展示随着互联网的发展,数据可视化已经成为了展示和分析数据的重要手段。而图表是数据可视化的核心,它能够将庞大的数据转化为直观的图形,使得数据更易于理解和分析。本文将介绍如何使用PHP和UniApp这两个实用工具来实现数据的图表展示。一、PHP的入门与安装PHP(全称:HypertextPreprocessor)是一种
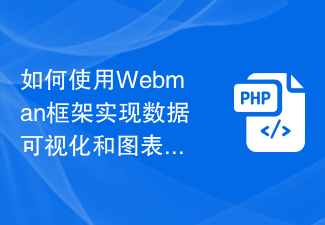
如何使用Webman框架实现数据可视化和图表展示功能?Webman是一个轻量级的PythonWeb框架,它提供了灵活且易于使用的工具,帮助开发者快速构建Web应用。在数据处理和可视化领域,Webman框架有很多功能可以帮助我们实现数据可视化和图表展示的需求。本文将介绍如何使用Webman框架来实现这些功能。首先,我们需要安装Webman框架。可以使用以下命


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
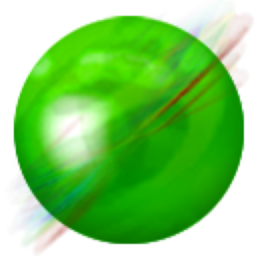
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
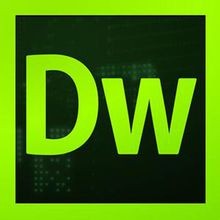
Dreamweaver CS6
Visual web development tools
