How to use PHP scripts to perform directory operations in Linux
How to use PHP scripts to perform directory operations in Linux
Directory operations are one of the commonly used functions in web development. Directories can be easily added through PHP scripts. , delete, modify, check and other operations. This article will introduce how to use PHP scripts to perform directory operations in Linux and provide relevant code examples.
1. Create a directory
To create a directory, you can use the mkdir()
function in PHP. This function accepts two parameters, the first parameter is the path of the directory to be created, and the second parameter is the permission settings.
The following is a sample code that demonstrates how to create a new directory named "test" in the specified directory of the Linux server:
<?php $dir = '/path/to/directory'; if (!is_dir($dir)) { // 检查目录是否已存在 mkdir($dir, 0777, true); // 创建目录 echo "目录创建成功!"; } else { echo "目录已存在!"; } ?>
2. Delete the directory
To To delete a directory and its contents, you can use the rmdir()
function in PHP. This function accepts one parameter, which is the path to the directory to be deleted. It should be noted that only empty directories can be deleted. If there are files or other subdirectories in the directory, all contents need to be deleted recursively.
The following is a sample code that demonstrates how to delete a directory named "test" and its contents:
<?php $dir = '/path/to/directory'; if (is_dir($dir)) { // 检查目录是否存在 $objects = scandir($dir); // 获取目录中的文件和子目录 foreach ($objects as $object) { if ($object != "." && $object != "..") { // 排除当前目录和上级目录 if (is_dir($dir . "/" . $object)) { rmdir_recursive($dir . "/" . $object); // 递归删除子目录 } else { unlink($dir . "/" . $object); // 删除文件 } } } rmdir($dir); // 删除目录 echo "目录删除成功!"; } else { echo "目录不存在!"; } function rmdir_recursive($dir) { $objects = scandir($dir); foreach ($objects as $object) { if ($object != "." && $object != "..") { if (is_dir($dir . "/" . $object)) { rmdir_recursive($dir . "/" . $object); } else { unlink($dir . "/" . $object); } } } rmdir($dir); } ?>
3. Traverse the directory
To traverse the files in the directory and subdirectories, you can use the scandir()
function in PHP. This function accepts one parameter, which is the path of the directory to be traversed, and returns an array containing the contents of the directory.
The following is a sample code that demonstrates how to traverse a directory named "test" and print the names of files and subdirectories in it:
<?php $dir = '/path/to/directory'; if (is_dir($dir)) { // 检查目录是否存在 $objects = scandir($dir); // 获取目录中的文件和子目录 foreach ($objects as $object) { if ($object != "." && $object != "..") { // 排除当前目录和上级目录 echo $object . "<br>"; } } } else { echo "目录不存在!"; } ?>
4. Modify directory permissions
To modify the permissions of the directory, you can use the chmod()
function in PHP. This function accepts two parameters. The first parameter is the path to the directory where the permissions are to be modified, and the second parameter is the new permission settings.
The following is a sample code that demonstrates how to set the permissions of a directory named "test" to 0777:
<?php $dir = '/path/to/directory'; if (is_dir($dir)) { // 检查目录是否存在 chmod($dir, 0777); // 修改目录权限 echo "目录权限修改成功!"; } else { echo "目录不存在!"; } ?>
With the above code sample, you can use a PHP script in Linux Directory operations, including creating directories, deleting directories, traversing directories, and modifying directory permissions. According to actual needs, these codes can be used flexibly to perform directory operations in web development.
The above is the detailed content of How to use PHP scripts to perform directory operations in Linux. For more information, please follow other related articles on the PHP Chinese website!
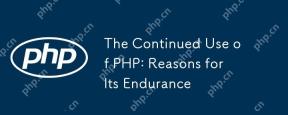
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
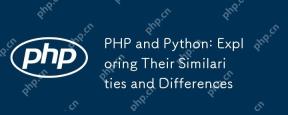
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
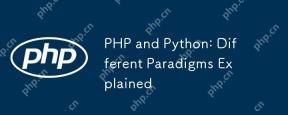
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
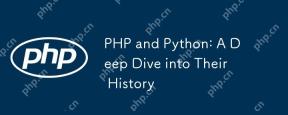
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
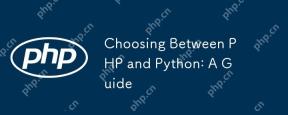
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
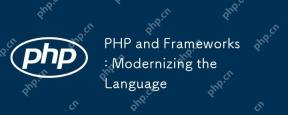
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
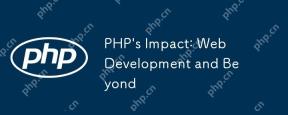
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
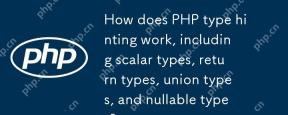
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
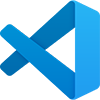
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
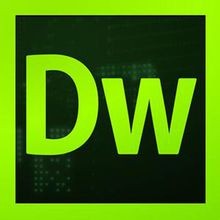
Dreamweaver CS6
Visual web development tools