Effectively use Python and the Linux platform for scripting
Effective use of Python and Linux platforms for scripting
In the modern technology and information age, Python and Linux have become very powerful tools and platforms for development and Run various script operations. Python is a high-level programming language with a concise and powerful syntax that can easily perform operations such as data processing, network programming, and automation tasks. Linux is an open source operating system that is widely used in servers, embedded systems and large computer clusters. Its command line operations and scripting functions allow users to easily perform various operations.
This article will introduce how to use the functions of the Linux operating system in Python code and give specific code examples.
Execute Linux commands in Python
Python's os module provides the function of executing operating system commands. We can use the os.system() function to execute Linux commands. The following is a simple example to execute the ls command in Python to list the files in the current directory:
import os # 执行ls命令 os.system("ls")
The above code will directly execute the ls
command in Python and store the results printed on the terminal.
Run Shell Script
In addition to executing a single command, we can also run an entire Shell script in Python. The following is an example of executing a simple Shell script in Python:
import os # 定义Shell脚本 script = ''' echo "Hello World" ls ''' # 将Shell脚本保存到文件 with open("script.sh", "w") as f: f.write(script) # 执行Shell脚本 os.system("sh script.sh")
The above code first defines a Shell script, which contains two commands: printing "Hello World" and listing the current directory document. Then save this script to a file (named script.sh), and finally use the os.system()
function to execute the Shell script.
Create and manage files through Python
Python is also very convenient to operate files. We can use Python to create, read, write and delete files. Here is an example showing how to use Python in Linux to create a file called "test.txt" and write some content to it:
# 创建文件 with open("test.txt", "w") as f: # 向文件中写入内容 f.write("Hello World!") # 读取文件内容 with open("test.txt", "r") as f: # 打印文件内容 print(f.read()) # 删除文件 os.remove("test.txt")
The above code first uses Python's open ()
The function creates a file named "test.txt" and opens it for writing content. Then use the write()
function to write "Hello World!" to the file. Next, we use the open()
function to open the file in read-only mode and the read()
function to read the file contents and print them out. Finally, use the os.remove()
function to delete the file.
Performing system tasks through Python
In practical applications, we may need to perform complex system tasks in Python. Python's subprocess
module provides powerful functionality to perform system tasks and obtain their output in Linux. The following is an example that shows how to execute the ping command in Python and get its output:
import subprocess # 执行ping命令 result = subprocess.run(["ping", "-c", "4", "www.google.com"], capture_output=True, text=True) # 输出ping命令的结果 print(result.stdout)
The above code uses the subprocess.run()
function to execute the ping command and save the result In the result
variable. We capture the output of the command by setting capture_output=True
, and text=True
to obtain the output content in text form. Finally, we use result.stdout
to print the output of the ping command.
Summary
The combination of Python and the Linux platform provides many powerful functions for script operations. Through the os module and subprocess module, we can easily execute Linux commands, run Shell scripts, create and manage files, and even perform complex system tasks in Python. These capabilities allow us to automate tasks and system management operations more efficiently.
Of course, the above are just some simple examples, and actual applications may be more complex and diverse. However, understanding these basic usage methods and code examples will help us better master the scripting capabilities of Python and Linux platforms.
The above is the detailed content of Effectively use Python and the Linux platform for scripting. For more information, please follow other related articles on the PHP Chinese website!
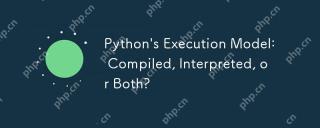
Pythonisbothcompiledandinterpreted.WhenyourunaPythonscript,itisfirstcompiledintobytecode,whichisthenexecutedbythePythonVirtualMachine(PVM).Thishybridapproachallowsforplatform-independentcodebutcanbeslowerthannativemachinecodeexecution.
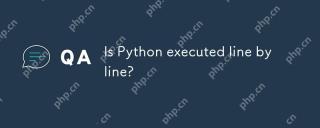
Python is not strictly line-by-line execution, but is optimized and conditional execution based on the interpreter mechanism. The interpreter converts the code to bytecode, executed by the PVM, and may precompile constant expressions or optimize loops. Understanding these mechanisms helps optimize code and improve efficiency.
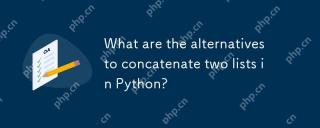
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
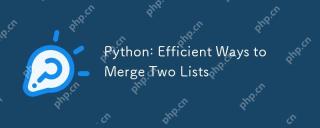
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
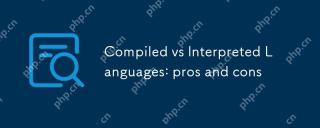
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
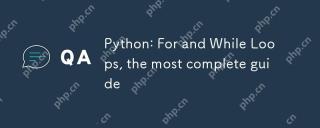
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
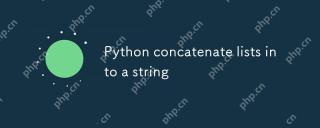
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
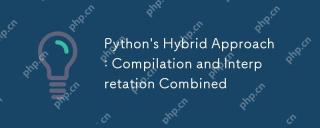
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
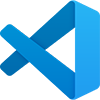
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
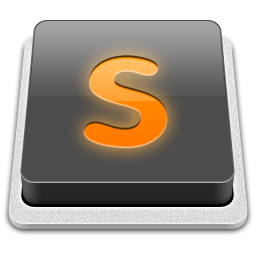
SublimeText3 Mac version
God-level code editing software (SublimeText3)
