


Optimize memory usage and garbage collection efficiency of Go language applications
Optimizing the memory usage and garbage collection efficiency of Go language applications
As an efficient, reliable, and concise programming language, Go language has been used in the development of applications in recent years. Procedural aspects are becoming more and more popular. However, like other programming languages, Go language applications also face problems with memory usage and garbage collection efficiency during operation. This article will explore some methods of optimizing Go language applications and provide specific code examples.
1. Reduce memory allocation
In the Go language, memory allocation is an expensive operation. Frequent memory allocation will not only cause the program to run slower, but may also trigger garbage collection. Frequent calls to the mechanism. Therefore, reducing memory allocation is very important to improve application performance.
- Using Object Pool
Object pool is a mechanism for reusing objects by pre-allocating a certain number of objects and saving them in a container. Obtain the object directly from the pool when you need to use it, and return it to the pool after use. This can avoid frequent memory allocation and recycling operations and improve memory usage efficiency.
type Object struct { // ... } var objectPool = sync.Pool{ New: func() interface{} { return &Object{} }, } func getObject() *Object { return objectPool.Get().(*Object) } func putObject(obj *Object) { objectPool.Put(obj) }
- Use slices or buffers
In the Go language, using slices or buffers can effectively avoid frequent memory allocation. Slices and buffers can allocate enough memory space in advance. When data needs to be stored, the data can be written directly to the allocated memory space instead of reallocating the memory every time.
const ( bufferSize = 1024 ) var buffer = make([]byte, bufferSize) func writeData(data []byte) { if len(data) > bufferSize { // 扩容 buffer = make([]byte, len(data)) } else { // 复用 buffer = buffer[:len(data)] } copy(buffer, data) }
- Avoid copying large data structures
When you need to copy the data structure, try to avoid copying the entire data structure. You can avoid unnecessary memory copies by passing pointers or using mutable data structures.
2. Reduce the pressure of garbage collection
The garbage collection mechanism of the Go language adopts a three-color marking method, and uses concurrent marking and STW (Stop-The-World) mechanism to reduce the pressure of garbage collection. Application Impact. However, garbage collection still takes up a certain amount of time and resources. Therefore, reducing the triggering frequency and amount of garbage collection is the key to optimizing Go language applications.
- Reduce memory allocation
As mentioned earlier, frequent memory allocation in the Go language will lead to frequent calls to the garbage collection mechanism. Therefore, reducing memory allocation also indirectly reduces the pressure of garbage collection.
- Avoid circular references
In the Go language, if there are circularly referenced data structures, the garbage collection mechanism cannot correctly identify and recycle these data structures, resulting in memory leaks. . Therefore, it is very important to avoid circular references.
type Node struct { data string next *Node } func createNodes() { nodes := make([]*Node, 0, 100) for i := 0; i < 100; i++ { node := &Node{ data: strconv.Itoa(i), } if i > 0 { node.next = nodes[i-1] } nodes = append(nodes, node) } }
- Explicitly trigger garbage collection
If there are a large number of temporary objects in the application, you can explicitly call runtime.GC() at the appropriate time
Method to manually trigger garbage collection. This reduces garbage collection latency and volume, improving application performance.
import "runtime" func doSomething() { // ... if shouldTriggerGC { runtime.GC() } // ... }
Summary
By optimizing memory usage and garbage collection efficiency, the performance and stability of Go language applications can be improved. During the development process, we should pay attention to avoid frequent memory allocation and use object pools and slices or buffers to reuse objects and reduce the overhead of memory allocation. In addition, attention should be paid to avoiding circular references and triggering garbage collection in time to avoid memory leaks and reduce the pressure of garbage collection. I hope that the optimization methods and code examples provided in this article can help you optimize the memory usage and garbage collection efficiency of Go language applications.
The above is the detailed content of Optimize memory usage and garbage collection efficiency of Go language applications. For more information, please follow other related articles on the PHP Chinese website!
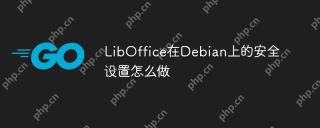
Ensuring overall security on Debian systems is crucial to protecting the running environment of applications such as LibOffice. Here are some general recommendations for improving system security: System updates regularly update the system to patch known security vulnerabilities. Debian12.10 released security updates that fixed a large number of security vulnerabilities, including some critical software packages. User permission management avoids the use of root users for daily operations to reduce potential security risks. It is recommended to create a normal user and join the sudo group to limit direct access to the system. The SSH service security configuration uses SSH key pairs to authenticate, disable root remote login, and restrict login with empty passwords. These measures can enhance the security of SSH services and prevent
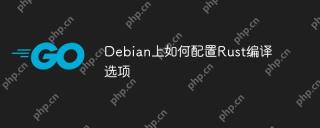
Adjusting Rust compilation options on Debian system can be achieved through various ways. The following is a detailed description of several methods: Use the rustup tool to configure and install rustup: If you have not installed rustup yet, you can use the following command to install: curl--proto'=https'--tlsv1.2-sSfhttps://sh.rustup.rs|sh Follow the prompts to complete the installation process. Set compilation options: rustup can be used to configure compilation options for different toolchains and targets. You can set compilation options for a specific project using the rustupoverride command. For example, if you want to set a specific Rust version for a project
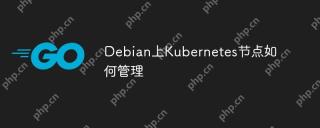
Managing Kubernetes (K8S) nodes on a Debian system usually involves the following key steps: 1. Installing and configuring Kubernetes components preparation: Make sure that all nodes (including master nodes and worker nodes) have the Debian operating system installed and meet the basic requirements for installing a Kubernetes cluster, such as sufficient CPU, memory and disk space. Disable swap partition: In order to ensure that kubelet can run smoothly, it is recommended to disable swap partition. Set firewall rules: allow necessary ports, such as ports used by kubelet, kube-apiserver, kube-scheduler, etc. Install container
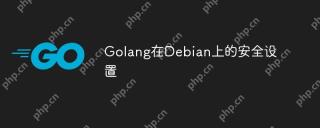
When setting up a Golang environment on Debian, it is crucial to ensure system security. Here are some key security setup steps and suggestions to help you build a secure Golang development environment: Security setup steps System update: Make sure your system is up to date before installing Golang. Update the system package list and installed packages with the following command: sudoaptupdatesudoaptupgrade-y Firewall Configuration: Install and configure a firewall (such as iptables) to limit access to the system. Only necessary ports (such as HTTP, HTTPS, and SSH) are allowed. sudoaptininstalliptablessud
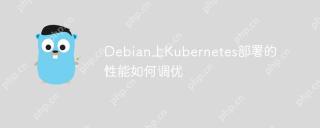
Optimizing and deploying Kubernetes cluster performance on Debian is a complex task involving multiple aspects. Here are some key optimization strategies and suggestions: Hardware resource optimization CPU: Ensure that sufficient CPU resources are allocated to Kubernetes nodes and pods. Memory: Increases the memory capacity of the node, especially for memory-intensive applications. Storage: Use high-performance SSD storage and avoid using network file systems (such as NFS) as they may introduce latency. Kernel parameter optimization edit /etc/sysctl.conf file, add or modify the following parameters: net.core.somaxconn: 65535net.ipv4.tcp_max_syn
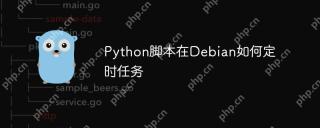
In the Debian system, you can use cron to arrange timed tasks and realize the automated execution of Python scripts. First, start the terminal. Edit the crontab file of the current user by entering the following command: crontab-e If you need to edit the crontab file of other users with root permissions, please use: sudocrontab-uusername-e to replace username with the username you want to edit. In the crontab file, you can add timed tasks in the format as follows: *****/path/to/your/python-script.py These five asterisks represent minutes (0-59) and small
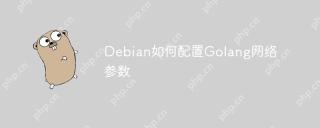
Adjusting Golang's network parameters in Debian system can be achieved in many ways. The following are several feasible methods: Method 1: Temporarily set environment variables by setting environment variables: Enter the following command in the terminal to temporarily set environment variables, and this setting is only valid in the current session. exportGODEBUG="gctrace=1netdns=go" where gctrace=1 will activate garbage collection tracking, and netdns=go will make Go use its own DNS resolver instead of the system default. Set environment variables permanently: add the above command to your shell configuration file, such as ~/.bashrc or ~/.profile
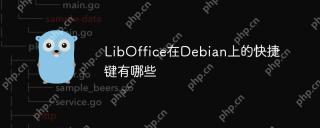
The shortcut keys for customizing LibOffice on Debian systems can be adjusted through system settings. Here are some commonly used steps and methods to set LibOffice shortcut keys: Basic steps to set LibOffice shortcut keys Open system settings: In the Debian system, click the menu in the upper left corner (usually a gear icon), and select "System Settings". Select a device: In the system settings window, select "Device". Select a keyboard: On the Device Settings page, select Keyboard. Find the command to the corresponding tool: In the keyboard settings page, scroll down to the bottom to see the "Shortcut Keys" option. Clicking it will bring a window to a pop-up. Find the corresponding LibOffice worker in the pop-up window


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
