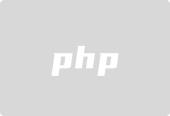
How to use Golang and FFmpeg to extract video frames
Abstract:
This article introduces how to use Golang and FFmpeg to extract video frames, and gives specific code examples. Through this method, each frame can be easily extracted from the video and subsequently processed and analyzed.
- Introduction
As video content continues to increase, the demand for video processing is also growing. Among them, video frame extraction is one of the basic steps for many video analysis and processing tasks. This article will introduce how to use Golang and FFmpeg to extract video frames.
- FFmpeg Overview
FFmpeg is an open source multimedia processing toolset that can convert, encode, and decode audio and video formats. It is one of the go-to tools for many video processing tasks and has a large user community and an active developer base.
- Installing FFmpeg
To use FFmpeg, you first need to install it on your computer. The compiled binaries can be downloaded from the official website (https://www.ffmpeg.org/) or installed through the package manager.
- Use Golang to call FFmpeg
In Golang, external commands can be executed through the os/exec package. We can use this package to call FFmpeg commands and extract video frames to a specified output directory.
The following is a sample code that shows how to use Golang to call the FFmpeg command to extract video frames:
package main
import (
"log"
"os"
"os/exec"
)
func main() {
inputFile := "input.mp4" // 输入视频文件
outputDir := "frames/" // 输出目录,存放提取出的视频帧
// 创建输出目录
err := os.MkdirAll(outputDir, os.ModePerm)
if err != nil {
log.Fatal(err)
}
// 构造FFmpeg命令
cmd := exec.Command("ffmpeg", "-i", inputFile, "-vf", "fps=1", outputDir+"frame-%03d.jpg")
// 执行命令
err = cmd.Run()
if err != nil {
log.Fatal(err)
}
log.Println("视频帧提取完成!")
}
The function of the above code is to put the specified video file (input.mp4) into Each frame is extracted and saved as a jpg format image file, stored in the frames/ directory. Among them, the "-vf fps=1" parameter means extracting one frame per second.
- Run the code
Save the above code as a go file, and then run go run filename.go
on the command line to start extracting video frames. The extraction process may take some time, depending on the size and frame rate of the video.
- Summary
This article introduces how to use Golang and FFmpeg to extract video frames. By calling the FFmpeg command, we can easily convert the video into a frame image and perform subsequent processing and analysis. I hope this article will be helpful to readers who need video frame extraction.
The above is the detailed content of How to extract video frames using Golang and FFmpeg. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn