


Optimization techniques for using RabbitMQ to implement task queues in Golang
Optimization tips for using RabbitMQ to implement task queues in Golang
RabbitMQ is an open source message middleware that supports a variety of message protocols, including AMQP (Advanced Messaging queue protocol). Task queues can be easily implemented using RabbitMQ in Golang to solve the asynchronous and high concurrency issues of task processing. This article will introduce some optimization techniques when using RabbitMQ to implement task queues in Golang, and give specific code examples.
- Persistent Messages
When using RabbitMQ to implement a task queue, we need to ensure that messages can be retained even if the RabbitMQ server restarts or crashes. In order to achieve this, we need to make the message persistent. In Golang, message persistence can be achieved by setting the DeliveryMode field to 2.
Sample code:
err := channel.Publish( "exchange_name", // 交换机名称 "routing_key", // 路由键 true, // mandatory false, // immediate amqp.Publishing{ DeliveryMode: amqp.Persistent, // 将消息设置为持久化的 ContentType: "text/plain", Body: []byte("Hello, RabbitMQ!"), })
- Batch confirmation message
In order to improve the performance of message processing, after each consumer successfully processes a batch of messages, We can confirm these messages in batches rather than one by one. In RabbitMQ, we can use the Channel.Qos method to specify the number of messages processed each time. By setting the autoAck parameter of the Channel.Consume method to false and calling the Delivery.Ack method after the consumer has processed a batch of messages, batch acknowledgment of messages can be achieved.
Sample code:
err := channel.Qos( 1, // prefetch count 0, // prefetch size false, // global ) messages, err := channel.Consume( "queue_name", // 队列名称 "consumer_id", // 消费者ID false, // auto ack false, // exclusive false, // no local false, // no wait nil, // arguments ) for message := range messages { // 处理消息 message.Ack(false) // 在处理完一批消息后调用Ack方法确认消息 if condition { channel.Ack(message.DeliveryTag, true) } }
- Control the number of consumers
In order to ensure the processing efficiency of the message queue, we need to reasonably control the number of consumers. In Golang, we can limit the number of messages processed by the consumer each time by setting the prefetch count parameter of the Channel.Qos method. In addition, we can also use the current limiting mechanism to dynamically control the number of consumers.
Sample code:
err := channel.Qos( 1, // prefetch count (每次处理的消息数量) 0, // prefetch size false, // global ) messages, err := channel.Consume( "queue_name", // 队列名称 "consumer_id", // 消费者ID false, // auto ack false, // exclusive false, // no local false, // no wait nil, // arguments ) // 控制消费者数量 // 当达到最大消费者数量时,将拒绝新的消费者连接 semaphore := make(chan struct{}, max_concurrent_consumers) for message := range messages { semaphore <- struct{}{} // 当有新的消费者连接时,将占用一个信号量 go func(message amqp.Delivery) { defer func() { <-semaphore // 当消费者处理完一批消息后,释放一个信号量 }() // 处理消息 message.Ack(false) }(message) }
With reasonable optimization techniques, we can use RabbitMQ to implement efficient task queues in Golang. Persistent messages, batch acknowledgment of messages and controlling the number of consumers are three important aspects to achieve task queue optimization. I hope this article will bring some help to developers who are using Golang and RabbitMQ.
The above is the detailed content of Optimization techniques for using RabbitMQ to implement task queues in Golang. For more information, please follow other related articles on the PHP Chinese website!
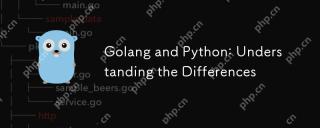
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
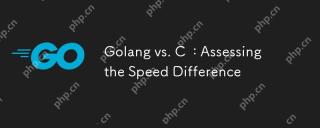
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
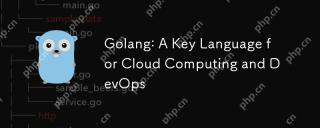
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
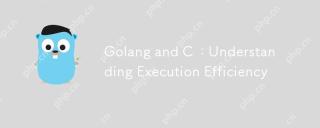
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
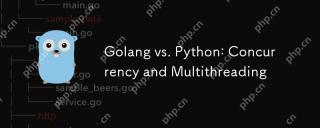
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
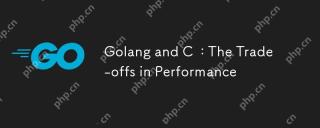
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
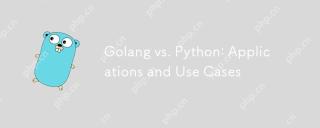
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
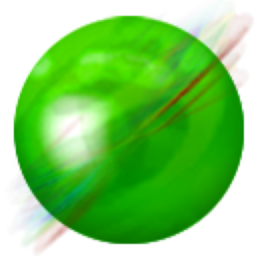
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
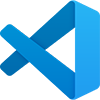
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.