


Best Practices for Memory Management and Garbage Collection in Go
Overview
Go is designed to be an efficient concurrent programming language with automatic memory management and garbage collection mechanism. Properly managing memory resources is critical to program performance and stability. This article will introduce some best practices for memory management and garbage collection in the Go language and provide specific code examples.
Avoid unnecessary memory allocation
When writing Go code, try to avoid frequently creating and destroying variables. Every time a variable is created and destroyed, memory space needs to be allocated and released, which will lead to frequent allocation and recycling of memory and reduce the performance of the program. Instead, try to reuse allocated memory space. For example, you can use sync.Pool to cache and reuse objects to avoid repeated memory allocation and recycling.
Sample code:
type MyObject struct { // ... } var myObjectPool = sync.Pool{ New: func() interface{} { return &MyObject{} }, } func GetMyObject() *MyObject { obj := myObjectPool.Get().(*MyObject) // 恢复对象初始状态 obj.Reset() return obj } func PutMyObject(obj *MyObject) { myObjectPool.Put(obj) }
Avoiding memory leaks
In the Go language, a memory leak refers to the inability to access or release memory space that is no longer used. When a variable is no longer used, you need to make sure it is set to nil so that the garbage collector can reclaim the memory space in time. If there are a large number of memory leaks in the program, it will cause excessive memory consumption and eventually cause the program to crash.
Sample code:
func process() { data := make([]byte, 1024) // 分配一块内存空间 // ... 使用data进行一些计算或操作 data = nil // 将data设置为nil,释放内存空间 // ... 其他代码 }
Avoid circular references
Circular references refer to two or more objects that refer to each other, resulting in the inability to be correctly recycled by the garbage collector. In order to avoid circular reference problems, you can use weak references or broken references to ensure that the object can be recycled correctly when it is no longer used.
Sample code:
type MyObject struct { otherObj *OtherObject // 与其他对象相互引用 } type OtherObject struct { // ... } func main() { obj := &MyObject{} otherObj := &OtherObject{} obj.otherObj = otherObj otherObj = nil // 断开引用 // ... 其他代码 }
Performance Tuning
For large data operations or computationally intensive tasks, in order to improve the performance and efficiency of the program, you can use memory pools or efficient data structure. The memory pool can cache allocated memory space to avoid frequent memory allocation and recycling. Efficient data structures can reduce memory usage and increase the speed of data access.
Sample code:
type MyObject struct { // ... } func main() { myObjectPool := make(chan *MyObject, 100) // 内存池,缓存100个对象 // 初始化对象池 for i := 0; i < 100; i++ { myObjectPool <- &MyObject{} } // ... 从对象池中获取对象并使用 obj := <-myObjectPool // ... // 将对象放回对象池 myObjectPool <- obj // ... 其他代码 }
Conclusion
By properly performing memory management and garbage collection, we can improve the performance and stability of Go language programs. The above-mentioned best practices include avoiding unnecessary memory allocation, avoiding memory leaks, avoiding circular references, and performing performance tuning, etc., which can help us write efficient and robust Go code.
It is worth noting that although the Go language has automatic memory management and garbage collection mechanisms, we still need to pay attention to the allocation and release of memory to make full use of system resources and improve program performance. Continuous attention and optimization of memory management will make our Go programs more efficient and reliable.
The above is the detailed content of Best practices for memory management and garbage collection in Go language. For more information, please follow other related articles on the PHP Chinese website!
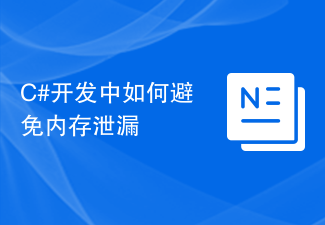
C#开发中如何避免内存泄漏,需要具体代码示例内存泄漏是软件开发过程中常见的问题之一,特别是在使用C#语言进行开发时。内存泄漏会导致应用程序占用越来越多的内存空间,最终导致程序运行缓慢甚至崩溃。为了避免内存泄漏,我们需要注意一些常见的问题并采取相应措施。及时释放资源在C#中,使用完资源后一定要及时释放它们,尤其是涉及到文件操作、数据库连接和网络请求等资源。可以
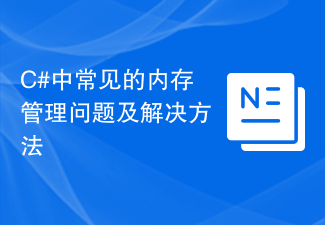
C#中常见的内存管理问题及解决方法,需要具体代码示例在C#开发中,内存管理是一个重要的问题,不正确的内存管理可能会导致内存泄漏和性能问题。本文将向读者介绍C#中常见的内存管理问题,并提供解决方法,并给出具体的代码示例。希望能帮助读者更好地理解和掌握内存管理技术。垃圾回收器不及时释放资源C#中的垃圾回收器(GarbageCollector)负责自动释放不再使
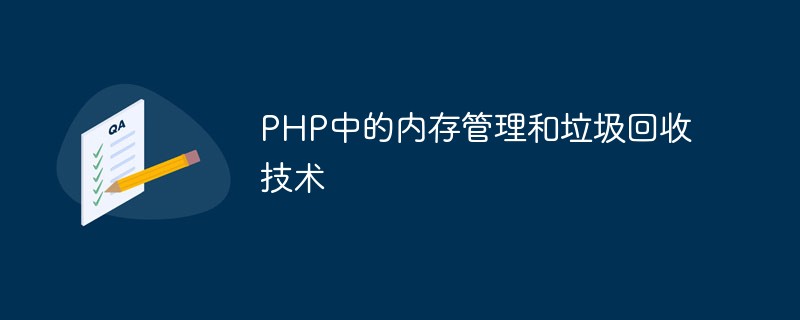
PHP作为一种广泛使用的脚本语言,为了在运行时保证高效执行,具有独特的内存管理和垃圾回收技术。本文将简单介绍PHP内存管理和垃圾回收的原理和实现方式。一、PHP内存管理的原理PHP的内存管理采用了引用计数(ReferenceCounting)来实现,这种方式是现代化的语言中比较常见的内存管理方式之一。当一个变量被使用时,PHP会为其分配一段内存,并将这段内
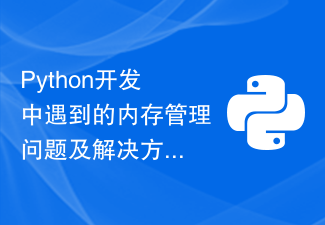
Python开发中遇到的内存管理问题及解决方案摘要:在Python开发过程中,内存管理是一个重要的问题。本文将讨论一些常见的内存管理问题,并介绍相应的解决方案,包括引用计数、垃圾回收机制、内存分配、内存泄漏等。并提供了具体的代码示例来帮助读者更好地理解和应对这些问题。引用计数Python使用引用计数来管理内存。引用计数是一种简单而高效的内存管理方式,它记录每
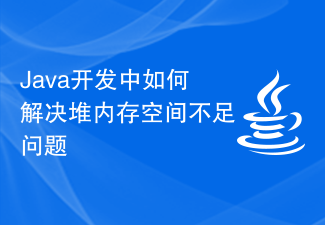
Java作为一门广泛使用的编程语言,由于其自动内存管理机制,特别是垃圾回收机制的存在,使得开发人员无需过多关注内存的分配和释放。然而,在一些特殊情况下,例如处理大数据或者运行复杂的算法时,Java程序可能会遇到堆内存空间不足的问题。本文将讨论如何解决这个问题。一、了解堆内存空间堆内存是Java虚拟机(JVM)中分配给Java程序运行时使用的内存空间。它存储了
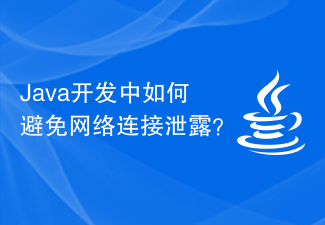
如何解决Java开发中的网络连接泄露问题随着信息技术的高速发展,网络连接在Java开发中变得越来越重要。然而,Java开发中的网络连接泄露问题也逐渐凸显出来。网络连接泄露会导致系统性能下降、资源浪费以及系统崩溃等问题,因此解决网络连接泄露问题变得至关重要。网络连接泄露是指在Java开发中未正确关闭网络连接,导致连接资源无法释放,从而使系统无法正常工作。解决网
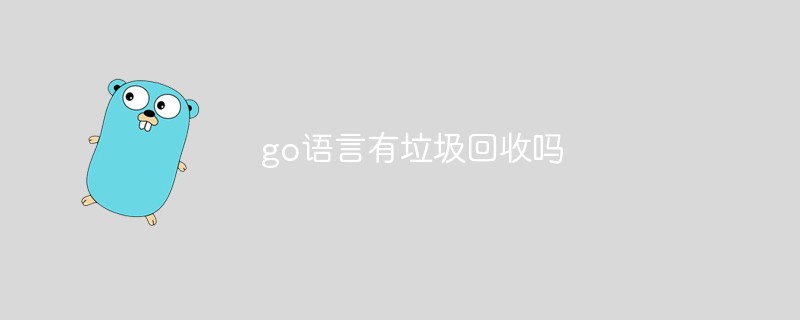
go语言有垃圾回收。Go语言自带垃圾回收机制(GC);GC通过独立的进程执行,它会搜索不再使用的变量,并将其释放。在计算中。内存空间包含两个重要的区域:栈区 (Stack) 和堆区 (Heap);栈区一般存储了函数调用的参数、返回值以及局部变量,不会产生内存碎片,由编译器管理,无需开发者管理;而堆区会产生内存碎片,在Go语言中堆区的对象由内存分配器分配并由垃圾收集器回收。
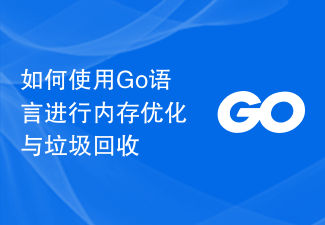
如何使用Go语言进行内存优化与垃圾回收Go语言作为一门高性能、并发、效率高的编程语言,对于内存的优化和垃圾回收有着很好的支持。在开发Go程序时,合理地管理和优化内存使用,能够提高程序的性能和可靠性。使用合适的数据结构在Go语言中,选择合适的数据结构对内存的使用有很大的影响。例如,对于需要频繁添加和删除元素的集合,使用链表代替数组可以减少内存碎片的产生。另外,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
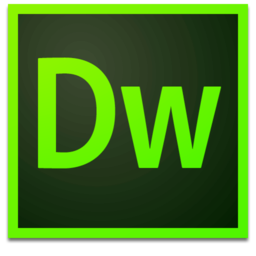
Dreamweaver Mac version
Visual web development tools