


The technical points of decoupling and decoupling between Golang and RabbitMQ require specific code examples
Overview:
In modern distribution In traditional systems, decoupling and decoupling between services is very important. To achieve this goal, we can use Golang and RabbitMQ to build a reliable and high-performance message queue system. This article will introduce how to use Golang and RabbitMQ to achieve decoupling and decoupling between services, and provide corresponding code examples.
Technical Point 1: Using RabbitMQ for message delivery
RabbitMQ is a powerful open source message queuing system that implements the AMQP (Advanced Message Queuing Protocol) protocol. It can be used as middleware between services, ensuring reliable delivery of messages and providing high throughput and low latency performance.
In Golang, we can use RabbitMQ's official client library streadway/amqp to produce and consume messages. The following is a sample code using RabbitMQ for messaging:
package main import ( "fmt" "log" "os" "github.com/streadway/amqp" ) func main() { // 创建连接 conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/") if err != nil { log.Fatal(err) } defer conn.Close() // 创建通道 ch, err := conn.Channel() if err != nil { log.Fatal(err) } defer ch.Close() // 声明队列 q, err := ch.QueueDeclare( "hello", // 队列名称 false, // 是否持久化 false, // 是否自动删除 false, // 是否独占 false, // 是否等待服务器响应 nil, // 其他参数 ) if err != nil { log.Fatal(err) } // 发布消息 body := "Hello, RabbitMQ!" err = ch.Publish( "", // exchange名称 q.Name, // routing key false, // mandatory:是否需要确认 false, // immediate:是否立即发送 amqp.Publishing{ ContentType: "text/plain", Body: []byte(body), }, ) if err != nil { log.Fatal(err) } fmt.Println("消息已发送") }
Technical point 2: Using Golang to implement consumers
In Golang, we can use RabbitMQ’s official client library streadway/amqp to write consumer code. The following is a sample code that uses Golang to implement a RabbitMQ consumer:
package main import ( "fmt" "log" "github.com/streadway/amqp" ) func main() { // 创建连接 conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/") if err != nil { log.Fatal(err) } defer conn.Close() // 创建通道 ch, err := conn.Channel() if err != nil { log.Fatal(err) } defer ch.Close() // 声明队列 q, err := ch.QueueDeclare( "hello", // 队列名称 false, // 是否持久化 false, // 是否自动删除 false, // 是否独占 false, // 是否等待服务器响应 nil, // 其他参数 ) if err != nil { log.Fatal(err) } // 消费消息 msgs, err := ch.Consume( q.Name, // 队列名称 "", // consumer标签 true, // auto-ack:是否自动确认 false, // exclusive:是否独占 false, // no-local:是否禁止本地消费 false, // no-wait:是否等待服务器响应 nil, // 其他参数 ) if err != nil { log.Fatal(err) } // 处理消息 for msg := range msgs { fmt.Printf("收到消息:%s ", msg.Body) } }
Conclusion:
By using Golang and RabbitMQ, we can achieve decoupling and decoupling between services. Message queues provide asynchronous communication capabilities and can decouple services, thereby improving the scalability and maintainability of the system. Using the code examples above, you can start building reliable and performant distributed systems. Hope this article is helpful to you.
The above is the detailed content of Golang and RabbitMQ realize the technical points of decoupling and decoupling between services. For more information, please follow other related articles on the PHP Chinese website!
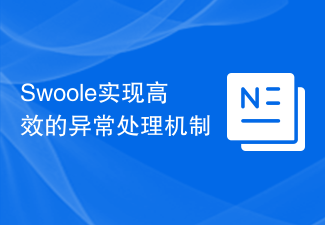
随着Web开发技术的不断发展,开发人员也面临着越来越复杂的业务场景和需求。例如,高并发、大量请求处理、异步任务处理等问题都需要使用高性能的工具和技术来解决。在这种情况下,Swoole成为了一种越来越重要的解决方案。Swoole是一种基于PHP语言的高性能异步网络通信框架。它提供了一些非常有用的功能和特性,例如异步IO、协程、进程管理、定时器和异步客户端,使得
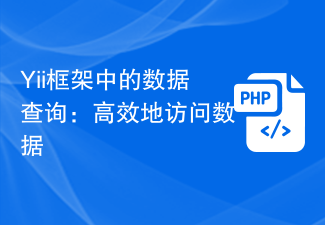
Yii框架是一个开源的PHPWeb应用程序框架,提供了众多的工具和组件,简化了Web应用程序开发的流程,其中数据查询是其中一个重要的组件之一。在Yii框架中,我们可以使用类似SQL的语法来访问数据库,从而高效地查询和操作数据。Yii框架的查询构建器主要包括以下几种类型:ActiveRecord查询、QueryBuilder查询、命令查询和原始SQL查询
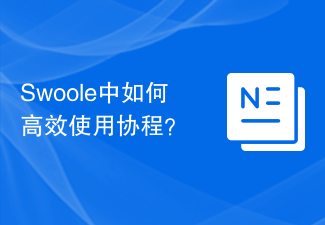
Swoole中如何高效使用协程?协程是一种轻量级的线程,可以在同一个进程内并发执行大量的任务。Swoole作为一个高性能的网络通信框架,对协程提供了支持。Swoole的协程不仅仅是简单的协程调度器,还提供了很多强大的功能,如协程池、协程原子操作,以及各种网络编程相关的协程封装等等,这些功能都可以帮助我们更高效地开发网络应用。在Swoole中使用协程有很多好处
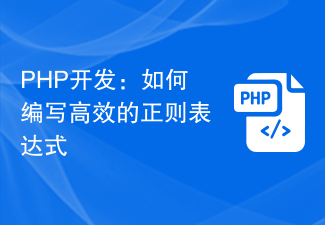
在PHP开发中,正则表达式是非常重要的工具,用于匹配、查找和替换文本中的特定字符串。然而,编写高效的正则表达式并不是一件易事,需要开发者具备一定的技巧和经验。下面是一些可以帮助您编写高效正则表达式的技巧:1.尽可能使用非贪婪匹配默认情况下,正则表达式是贪婪的,即它们将尽可能匹配更多的文本。在某些情况下,可能需要使用非贪婪匹配来避免这种情况。非贪婪匹配使用"
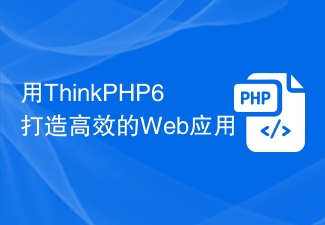
随着Web应用的广泛应用,越来越多的开发者开始寻求一种高效快捷的方式来构建他们的应用。近年来,ThinkPHP6作为一款优秀的PHP框架,逐渐成为了整个领域中的佼佼者。在本文中,我们将会介绍如何使用ThinkPHP6打造出高效的Web应用,让你轻松应对业务中的各种挑战。1.ThinkPHP6简介ThinkPHP6是一款轻量级的高性能PHP框架,它为开发者提
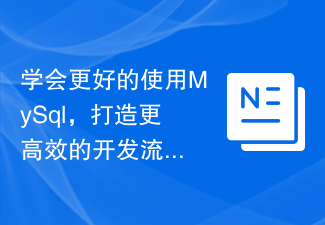
随着互联网的发展,数据已经成为企业和组织最重要的资产,而MySQL作为最流行的开源关系型数据库管理系统,为了有效提升开发效率及质量,各种需求被满足,MySQL经历了多年演化,成为企业常用的数据库之一。在日常工作中,使用MySAL频率较高,学会如何更好地使用MySQL将会对我们存储和管理数据起到非常重要的作用,从而提升我们的工作效率和工作质量,本文将
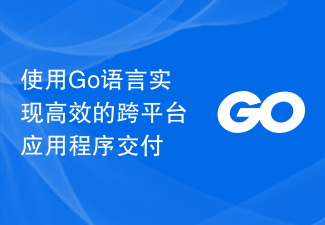
使用Go语言实现高效的跨平台应用程序交付摘要:随着跨平台应用程序需求的增加,开发人员需要一种高效的方式来交付能够在不同操作系统上运行的应用程序。在本文中,我们将介绍如何使用Go语言来实现高效的跨平台应用程序交付,并给出相应的代码示例。一、引言随着移动互联网的快速发展,跨平台应用程序变得越来越重要。在开发过程中,开发人员面临着如何将应用程序在不同操作系统上运行
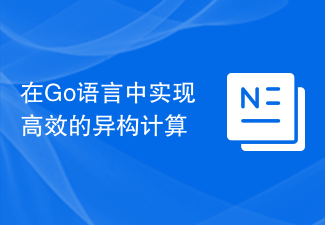
随着信息技术的不断发展,各种计算任务的复杂化和数量化需求日益增加,如何利用多种计算资源高效地完成这些任务已成为亟待解决的问题之一。而异构计算正是解决这一问题的有效手段之一,它可以利用各种不同类型的计算资源,如GPU、FPGA等,协同工作,实现高效的计算。本文将介绍如何在Go语言中实现高效的异构计算。一、异构计算的基本概念异构计算是通过组合不同类型的计算资源,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
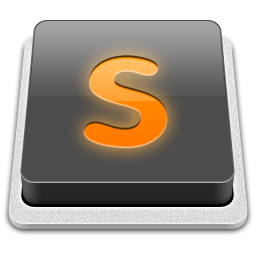
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
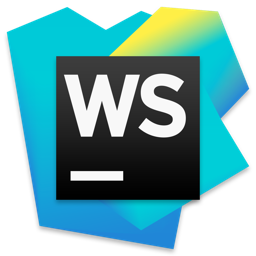
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
